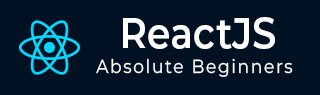
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 校验
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - Reconciliation (协调)
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode (严格模式)
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testRenderer.toTree() 方法
testRenderer.toTree()
是一个测试函数,它记录并表示渲染结构的详细图像,类似于在测试场景中拍摄快照。此函数比其他方法(如 toJSON()
)提供更多信息,并且包含用户编写的组件。通常,在创建我们自己的测试工具时,或者当我们想要在编程测试期间获得创建项的非常具体的视图时,我们会使用它。
这在测试计算机程序中的内容时非常有用,尤其是在我们想要检查其他人创建的部分时。这类似于查看详细地图以了解应用程序的所有不同部分。因此,testRenderer.toTree()
允许我们以详细的方式查看测试中正在发生的事情。
语法
testRenderer.toTree()
参数
testRenderer.toTree()
不需要任何参数。这就像拍摄测试中正在发生的事情的照片,它不需要任何额外信息来做到这一点。
返回值
testRenderer.toTree()
函数返回一个显示渲染树的对象。
示例
示例 - 基本组件
首先,我们将创建一个简单的应用程序,在其中我们将使用 testRenderer.toTree()
函数。这个应用程序就像在 React 中拍摄一条简单消息的图片。消息显示为“Hello, React!”,它在一个框内。testRenderer.toTree()
函数帮助我们查看此消息和框是如何组合在一起的。
import React from 'react'; import TestRenderer from 'react-test-renderer'; // Simple functional component const MyComponent = () => <div><h1>Hello, React! </h1></div>; export default MyComponent; // Creating a test renderer const testRenderer = TestRenderer.create(<MyComponent />); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
输出
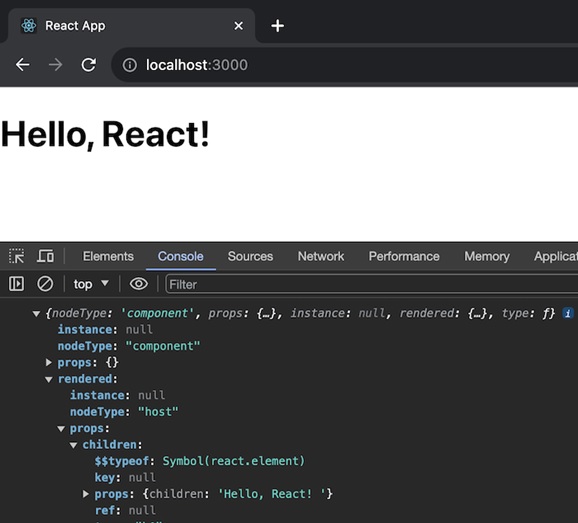
示例 - 属性和状态
在第二个应用程序中,我们将创建一个计数器应用程序。假设计数器就像记分牌上的数字。这个应用程序就像在 React 中拍摄该计数器的快照。我们可以通过单击按钮来增加计数。testRenderer.toTree()
函数向我们展示了这个计数器和按钮是如何设置的。
import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; // Component with props and state const Counter = ({ initialCount }) => { const [count, setCount] = useState(initialCount); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter; // Creating a test renderer with props const testRenderer = TestRenderer.create(<Counter initialCount={5} />); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
输出
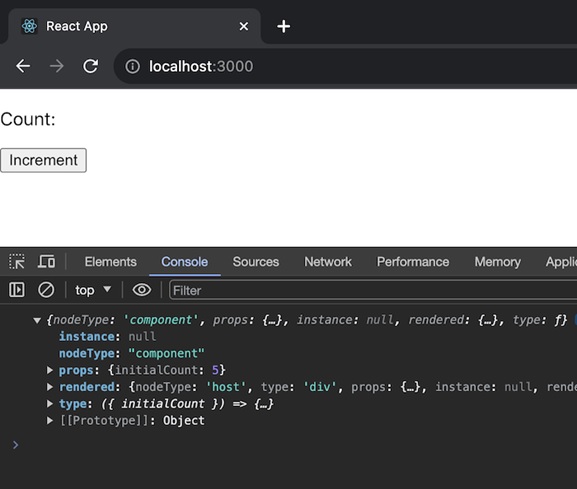
示例 - 带有子组件的组件
最后,我们将创建一个包含子组件的组件。这就像在 React 中拍摄一张全家福。有一个父组件,其中将显示消息“Parent Component”,还有一个子组件,其中显示消息“I'm a child”。testRenderer.toTree()
函数让我们看到这些父组件和子组件是如何排列的。
import React from 'react'; import TestRenderer from 'react-test-renderer'; // Component with children const ParentComponent = ({ children }) => ( <div> <h2>Parent Component</h2> {children} </div> ); export default ParentComponent; // Child component const ChildComponent = () => <p>I'm a child!</p>; // test renderer with nested components const testRenderer = TestRenderer.create( <ParentComponent> <ChildComponent /> </ParentComponent> ); // Using testRenderer.toTree() const tree = testRenderer.toTree(); console.log(tree);
输出
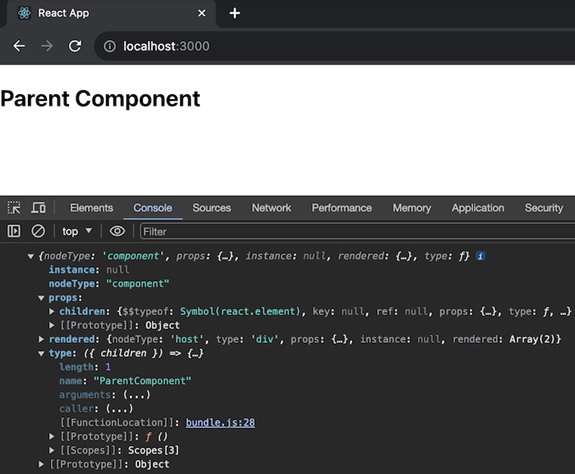
调用 testRenderer.toTree()
时,这三个应用程序的输出看起来都像是生成的树的详细表示。输出将是一个包含组件信息、它们的 props 以及虚拟 DOM 结构的对象。
总结
在本教程中,我们学习了 testRenderer.toTree()
方法。我们探索了三个简单的 React 应用程序,并使用 testRenderer.toTree()
函数拍摄了它们渲染方式的详细快照。每个应用程序都代表不同的场景。它帮助我们理解组件的结构以及它们如何相互交互。