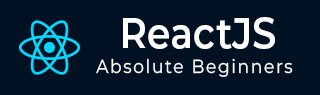
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 功能
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 例子
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testRenderer.unmount() 方法
卸载内存树听起来可能很困难,但这是一个简单的操作,只需要执行正确的生命周期事件。在本教程中,我们将讨论使用 testRenderer.unmount() 方法的各个阶段。
内存树是我们 React 组件在计算机内存中存在的表示。它类似于用户界面的虚拟版本。
有时我们必须从内存树中卸载或取消安装组件。这可能是因为我们不再需要它,或者我们正在清理测试后的内容。
React 测试中的 testRenderer.unmount() 方法用于卸载组件。可以把它想象成删除虚拟标志或显示。
语法
testRenderer.unmount()
参数
unmount() 方法不接受任何参数。
返回值
unmount() 方法不生成任何输出。当我们调用 unmount() 时,React 会执行其工作而无需返回任何内容。
示例
使用 testRenderer.unmount() 卸载内存树是一个简单的过程。因此,我们将通过不同的示例来了解此函数的用法。
示例 - 基本组件卸载
// Basic Component Unmount import React from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { // Render a simple component const testRenderer = TestRenderer.create(<div>Hello, App!</div>); //unmounting the component testRenderer.unmount(); // The component is now removed from the tree return null; // This component doesn't render anything visible } export default App;
输出
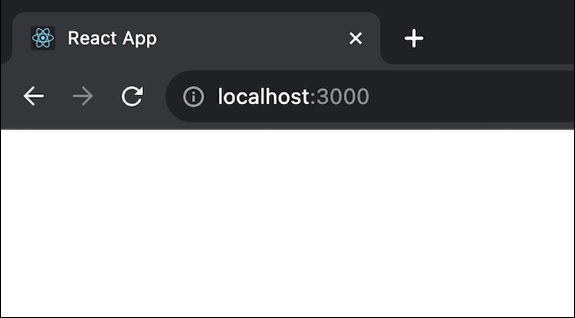
由于组件立即卸载,因此不会有任何可见的输出。组件在渲染后立即从内存树中移除。
示例 - 条件渲染和卸载
在这个应用中,我们将根据状态 (showComponent) 有条件地渲染组件。延迟 2 秒后,状态将更新为隐藏组件,并使用 testRenderer.unmount() 函数来显示卸载。
// Conditional Rendering and Unmount import React, { useState, useEffect } from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { const [showComponent, setShowComponent] = useState(true); useEffect(() => { // After a delay, toggle the state to hide the component const timeout = setTimeout(() => { setShowComponent(false); }, 2000); return () => clearTimeout(timeout); // Cleanup to avoid memory leaks }, []); return ( <div> {showComponent && <div>Hello, App!</div>} {!showComponent && ( // unmounting the component after it is hidden <TestRenderer.unmount /> )} </div> ); } export default App;
输出
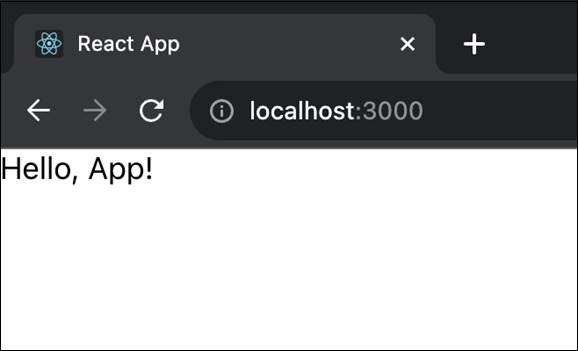
最初,我们将看到显示“Hello, App!”。2 秒后,组件消失,并调用 testRenderer.unmount() 函数。输出将为空页面。
示例 - 使用状态的动态组件卸载
这个应用将根据按钮点击动态渲染或卸载组件。组件可见性将由 componentVisible 状态控制,当组件隐藏时将使用 testRenderer.unmount() 函数。
// Dynamic Component Unmount with State import React, { useState } from 'react'; import TestRenderer from 'react-test-renderer'; const App = () => { const [componentVisible, setComponentVisible] = useState(true); const handleUnmount = () => { // Toggle the state to hide or show the component setComponentVisible(!componentVisible); }; return ( <div> {componentVisible && <div>Hello, App!</div>} <button onClick={handleUnmount}> {componentVisible ? 'Unmount Component' : 'Mount Component'} </button> {!componentVisible && ( // unmounting the component based on state <TestRenderer.unmount /> )} </div> ); } export default App;
输出
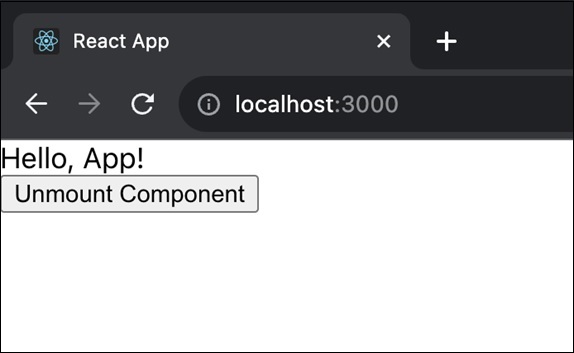
最初,我们将看到“Hello, App!”和一个按钮。单击按钮会更改组件的可见性,当它隐藏时,将调用 testRenderer.unmount() 函数。
总结
testRenderer.unmount() 是清理 React 测试中组件的有用工具。记住,它是一行代码,没有参数,没有返回值,只是一个简单的命令来整理我们的内存树。我们创建了三个不同的示例来展示使用 testRenderer.unmount() 函数的各种场景。