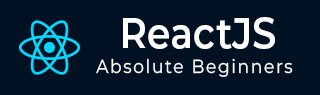
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 功能
- ReactJS - 优势与劣势
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 触摸事件处理程序
触摸事件对于增强用户体验非常重要,尤其是在智能手机和平板电脑等触摸设备上。我们将了解几种触摸事件、它们的类型以及如何在 React 应用中处理它们。
触摸事件是更大的 Web 开发 UI 事件家族的一部分。这些事件允许我们记录和响应用户的活动,例如点击、滑动和捏合。
语法
<div onTouchStart={e => console.log('onTouchStart')} onTouchMove={e => console.log('onTouchMove')} onTouchEnd={e => console.log('onTouchEnd')} onTouchCancel={e => console.log('onTouchCancel')} />
参数
e − 这是一个 React 事件对象,我们可以将其与 TouchEvent 属性一起使用。
TouchEvent 类型
需要注意四种主要的触摸事件类型:
序号 | 类型与描述 |
---|---|
1 | touchstart 当用户将手指放在触摸表面上时,会触发此事件。 |
2 | touchend 当用户将手指从表面移开或触摸点移出表面边缘时,会发生 touchend 事件。 |
3 | touchmove 当用户在表面上移动手指时发生此事件。它会持续监控接触点的移动。 |
4 | touchcancel 当触摸点以某种方式被打扰时,会发送此消息。 |
TouchEvent 属性
需要注意一些触摸事件属性:
序号 | 属性与描述 |
---|---|
1 | altKey 显示在事件发生时是否按下了 Alt 键。 |
2 | ctrlKey 指示在事件期间是否点击了 Ctrl 键。 |
3 | changedTouches 显示已更改触摸的 Touch 对象列表。 |
4 | getModifierState(key) 一个返回布尔值的函数,显示是否按下了修饰键(例如,Shift、Alt 或 Ctrl)。 |
5 | metaKey 显示在事件期间是否按下了 Meta 键。 |
6 | shiftKey 指示在事件期间是否点击了 Shift 键。 |
7 | touches 代表触摸表面上所有当前触摸的 Touch 对象列表。 |
8 | targetTouches 显示目标元素上触摸的 Touch 对象列表。 |
9 | detail 显示用于其他事件特定信息的整数值。 |
10 | view 引用生成事件的 Window 对象。 |
示例
示例 - 简单触摸应用
我们将创建一个简单的 React 应用来显示 TouchEvent 处理程序以及下面的代码。这是一个小型 React 应用,它在控制台中显示触摸事件:
import React from "react"; class App extends React.Component { render() { return ( <div onTouchStart={(e) => console.log("onTouchStart")} onTouchMove={(e) => console.log("onTouchMove")} onTouchEnd={(e) => console.log("onTouchEnd")} onTouchCancel={(e) => console.log("onTouchCancel")} style={{ width: "200px", height: "200px", backgroundColor: "lightblue", textAlign: "center", lineHeight: "200px" }} > TouchEvent </div> ); } } export default App;
输出
This code generates the App React component. When one of the four touch events (touchstart, touchmove, touchend, and touchcancel) happens, the message is logged in the console. The component also provides a clickable <div> element with which we can interact on a touch-enabled device.
示例 - 长按应用
给定的 React 应用名为“LongPressApp”,旨在检测触摸设备上的长按操作。该应用有一个具有浅蓝色背景的 div 元素,其宽度和高度均为 200 像素。当我们触摸并按住应用至少 1000 毫秒时,它会在控制台中记录一条消息,指出“检测到长按”。
import React from 'react'; class App extends React.Component { handleTouchStart = () => { this.longPressTimer = setTimeout(() => { console.log('Long press detected'); }, 1000); // Adjust the duration for long press threshold }; handleTouchEnd = () => { clearTimeout(this.longPressTimer); }; render() { return ( <div onTouchStart={this.handleTouchStart} onTouchEnd={this.handleTouchEnd} style={{ width: '200px', height: '200px', backgroundColor: 'lightblue', textAlign: 'center', lineHeight: '200px', }} > LongPressApp </div> ); } } export default App;
输出
Open the app in a browser. Touch the app and keep your touch held for at least 1000 milliseconds. After the specified duration, we will be able to see the message "Long press detected" logged to the browser's console.
示例 - 捏合缩放应用
Pinch Zoom React 应用旨在检测捏合手势的开始和结束,通常用于触摸设备上的缩放操作。该应用有一个具有浅蓝色背景的 div 元素,其宽度和高度均为 200 像素。当用户开始捏合手势时,它会在控制台中记录消息“捏合开始”。类似地,当用户结束捏合手势时,它会记录消息“捏合结束”。
import React from 'react'; class App extends React.Component { handleTouchStart = (e) => { if (e.touches.length === 2) { console.log('Pinch started'); } }; handleTouchEnd = (e) => { if (e.touches.length < 2) { console.log('Pinch ended'); } }; render() { return ( <div onTouchStart={this.handleTouchStart} onTouchEnd={this.handleTouchEnd} style={{ width: '200px', height: '200px', backgroundColor: 'lightblue', textAlign: 'center', lineHeight: '200px', }} > PinchZoomApp </div> ); } } export default App;
输出
Open the app in a browser. Use a touch-enabled device (like smartphone or tablet). Touch the app with two fingers simultaneously to trigger the "Pinch started" message. Release one or both fingers to trigger the "Pinch ended" message.
总结
触摸事件在 React 应用中扮演着重要的角色,用于创建引人入胜和交互式用户界面,尤其是在触摸设备上。了解各种触摸事件以及如何管理它们,使我们能够为网站或应用程序用户提供轻松的用户体验。