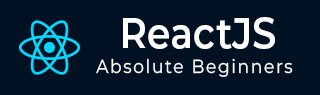
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - Reconciliation (协调)
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode (严格模式)
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - UNSAFE_componentWillMount() 方法
React 中的 componentWillMount() 函数就像组件出现在网页上之前的准备步骤。它发生在组件显示之前。此函数获取数据,但需要注意的是,在组件第一次显示之前,它不会提供任何结果。由于获取数据可能需要一些时间,因此组件不会等待此阶段完成才显示自身。
在最新的 React 版本中,不再推荐使用 componentWillMount()。他们建议改用 componentDidMount()。如果我们确实想要使用 componentWillMount(),我们可以使用,但是我们必须将其称为 UNSAFE_componentWillMount()。这就像一个警告标志,提醒 React 开发者应该避免使用它。
语法
UNSAFE_componentWillMount() { //the action will come here that we want to execute }
参数
此方法不接受任何参数。
返回值
此方法不应该返回任何值。
示例
示例 - 显示警告消息
借助 UNSAFE_componentWillMount() 函数,这是一个在组件加载之前显示警告的 React 应用示例。
import React, { Component } from 'react'; class App extends Component { UNSAFE_componentWillMount() { alert("This message will appear before the component loads."); } render() { return ( <div> <h1>Welcome to My React App</h1> <p>This is a simple application using UNSAFE_componentWillMount().</p> </div> ); } } export default App;
输出
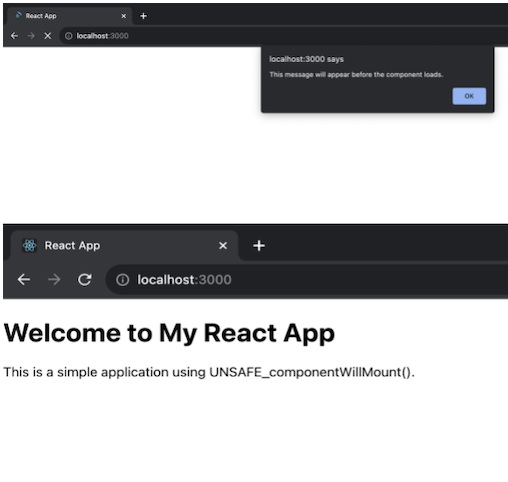
这是一个简单的 UNSAFE_componentWillMount() 应用示例,用于在网页上加载 React 组件之前显示消息。请记住,在最新的 React 版本中,对于类似的功能,建议使用 componentDidMount() 而不是 UNSAFE_componentWillMount()。
示例 - 数据获取应用
在这个示例中,我们将创建一个组件,在即将挂载时从 JSONPlaceholder API 获取数据。然后将获取到的数据存储在组件的状态中,组件在数据可用后呈现数据。因此,此应用的代码如下:
import React, { Component } from 'react'; import './App.css' class DataFetchingApp extends Component { constructor() { super(); this.state = { data: null, }; } UNSAFE_componentWillMount() { // fetching data from an API fetch('https://jsonplaceholder.typicode.com/todos/1') .then((response) => response.json()) .then((data) => { this.setState({ data }); }) .catch((error) => console.error('Error fetching data:', error)); } render() { const { data } = this.state; return ( <div className='App'> <h1>Data Fetching App</h1> {data ? ( <div> <h2>Data from API:</h2> <p>Title: {data.title}</p> <p>User ID: {data.userId}</p> <p>Completed: {data.completed ? 'Yes' : 'No'}</p> </div> ) : ( <p>Loading data...</p> )} </div> ); } } export default DataFetchingApp;
App.css
.App { align-items: center; justify-content: center; margin-left: 500px; margin-top: 50px; } h1 { color: red; } p { margin: 8px 0; color: green; }
输出
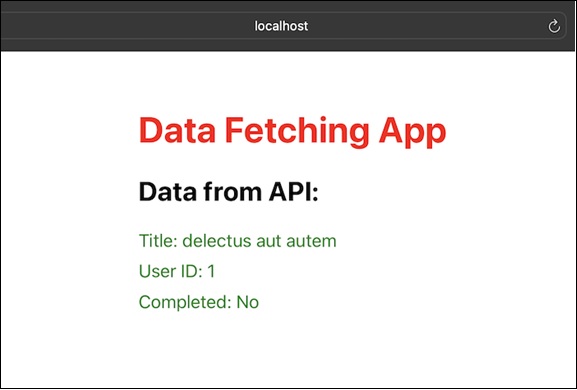
在这个 React 应用中,我们使用了 UNSAFE_componentWillMount() 来在组件挂载之前从 API 获取数据。因此,我们使用了 API 来获取数据。
示例 - 倒计时器应用
现在我们还有一个简单的 React 应用,它使用 UNSAFE_componentWillMount() 来设置倒计时器。在这个应用程序中,我们将有一个计时器,它将倒计时 10 秒,10 秒后,它将在屏幕上显示一条警告消息,表明时间已达到零。因此,此应用的代码如下:
import React, { Component } from 'react'; import './App.css'; class CountdownTimerApp extends Component { constructor() { super(); this.state = { seconds: 10, }; } UNSAFE_componentWillMount() { this.startTimer(); } startTimer() { this.timerInterval = setInterval(() => { this.setState((prevState) => ({ seconds: prevState.seconds - 1, }), () => { if (this.state.seconds === 0) { clearInterval(this.timerInterval); alert('Countdown timer reached zero!'); } }); }, 1000); } render() { return ( <div className='App'> <h1>Countdown Timer App</h1> <p>Seconds remaining: {this.state.seconds}</p> </div> ); } } export default CountdownTimerApp;
输出
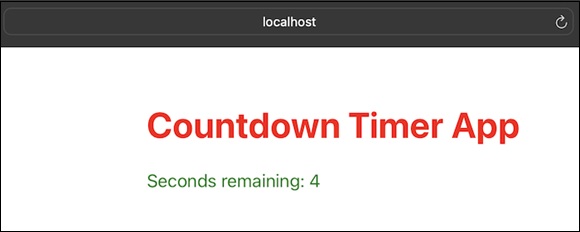
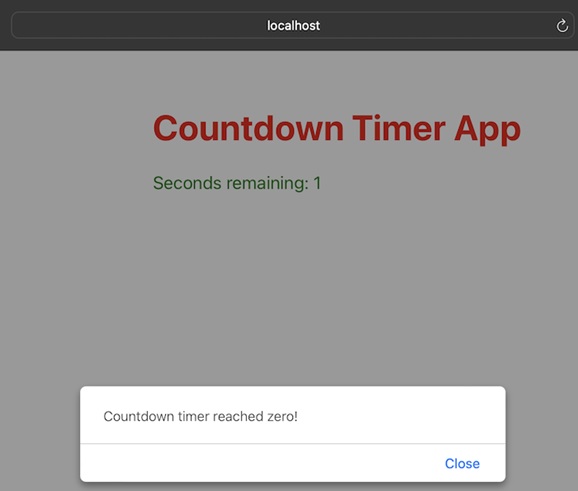
在这个示例中,组件在 UNSAFE_componentWillMount() 生命周期方法中开始倒计时器。每秒,计时器都会递减 seconds 状态。当倒计时达到零时,间隔被清除并显示警报。
限制
如果组件使用 getDerivedStateFromProps 或 getSnapshotBeforeUpdate,则不会调用此方法。
UNSAFE_componentWillMount 不能保证组件在某些当前 React 场景(如 Suspense)中会被挂载。
它被称为“不安全”是因为在某些情况下,React 可以丢弃正在进行的组件树并重建它,这使得 UNSAFE_componentWillMount 变得不可靠。
如果我们需要执行依赖于组件挂载的活动,请改用 componentDidMount。
UNSAFE_componentWillMount 是服务器端渲染时唯一执行的生命周期方法。
对于大多数实际用途,它与构造函数类似,因此最好使用构造函数来实现等效逻辑。
总结
UNSAFE_componentWillMount() 是一个较旧的 React 生命周期函数,用于组件加载前的任务,但在现代 React 编程中,建议遵循当前的最佳实践,并为此类任务使用 componentDidMount()。