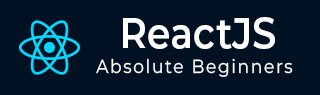
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 碎片
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - UNSAFE_componentWillReceiveProps() 方法
UNSAFE_componentWillReceiveProps 是一个 React 函数。它用于表示组件即将获得新的 props 或上下文。请记住,如果组件使用 getDerivedStateFromProps 或 getSnapshotBeforeUpdate,则不会调用 UNSAFE_componentWillReceiveProps。此外,它不能保证组件一定会收到新的 props,这在使用 Suspense 等最新的 React 功能时尤其重要。
语法
UNSAFE_componentWillReceiveProps(nextProps, nextContext)
参数
nextProps − 这些是组件从父组件接收到的新 props。我们可以通过比较 nextProps 和 this.props 来查看发生了哪些变化。
nextContext − 组件将需要从最近的提供者那里获取新的上下文。要找出有什么不同,请将 nextContext 与 this.context 进行比较。这仅在提供 static contextType 或 static contextTypes 时可用。
返回值
该方法不返回任何内容。它只是一个 React 在 props 或上下文即将更改时调用的函数。
示例
示例 - 消息应用
以下是如何在一个小型 React 应用中使用 UNSAFE_componentWillReceiveProps 的示例。在此示例中,我们将创建一个显示消息并在接收新 props 时更新消息的组件。
import React, { Component } from 'react'; class MessageComponent extends Component { constructor(props) { super(props); this.state = { message: props.initialMessage, }; } // This is the UNSAFE_componentWillReceiveProps function UNSAFE_componentWillReceiveProps(nextProps) { if (nextProps.newMessage !== this.props.newMessage) { // Check if the new message is different this.setState({ message: nextProps.newMessage }); } } render() { return <div>{this.state.message}</div>; } } class App extends Component { constructor() { super(); this.state = { message: 'Hello, Tutorialspoint!', newMessage: 'Welcome to the React App!', }; } updateMessage = () => { this.setState({ newMessage: 'Message is Updated!' }); }; render() { return ( <div> <MessageComponent initialMessage={this.state.message} newMessage={this.state.newMessage} /> <button onClick={this.updateMessage}>Update Message</button> </div> ); } } export default App;
输出
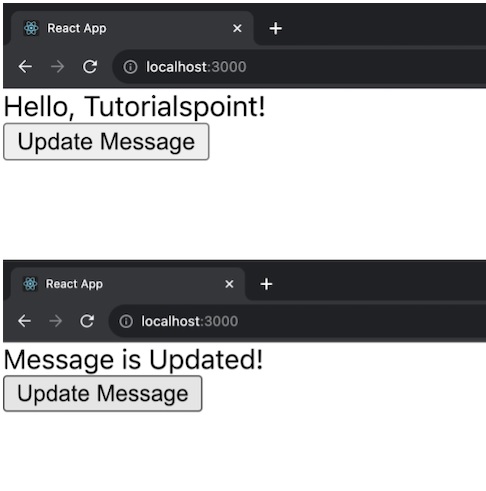
上述示例中有两个组件:App 和 MessageComponent。当接收到新的 props 时,MessageComponent 使用 UNSAFE_componentWillReceiveProps 更新其消息。App 组件有一个按钮可以更改消息。
示例 - 计数器应用
让我们再创建一个简单的 React 应用,并为应用添加 CSS 进行样式设置。这个应用将是一个基本的待办事项列表,它展示了 UNSAFE_componentWillReceiveProps() 函数的使用。以下是为此应用编写的代码:
TodoList.js
import React, { Component } from 'react'; import './TodoList.css'; class TodoList extends Component { constructor(props) { super(props); this.state = { todos: [], newTodo: '', }; } UNSAFE_componentWillReceiveProps(nextProps) { // Update todos when receiving new props if (nextProps.todos !== this.props.todos) { this.setState({ todos: nextProps.todos }); } } handleInputChange = (event) => { this.setState({ newTodo: event.target.value }); }; handleAddTodo = () => { if (this.state.newTodo) { const updatedTodos = [...this.state.todos, this.state.newTodo]; this.setState({ todos: updatedTodos, newTodo: '' }); } }; render() { return ( <div className="todo-container App"> <h2>ToDo List</h2> <ul> {this.state.todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> <div className="input-container"> <input type="text" value={this.state.newTodo} onChange={this.handleInputChange} placeholder="Add a new todo" /> <button onClick={this.handleAddTodo}>Add</button> </div> </div> ); } } export default TodoList;
TodoList.css
.todo-container { max-width: 400px; margin: 20px auto; padding: 20px; border: 1px solid #ccc; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } ul { list-style: none; padding: 0; } li { margin-bottom: 8px; } .input-container { margin-top: 16px; display: flex; } input { flex-grow: 1; padding: 8px; margin-right: 8px; } button { padding: 8px 16px; background-color: #4caf50; color: #fff; border: none; border-radius: 4px; cursor: pointer; } button:hover { background-color: #45a049; }
输出
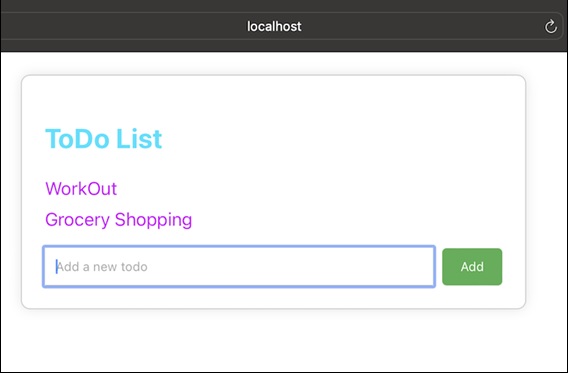
示例 - 个人资料应用
以下是如何使用 UNSAFE_componentWillReceiveProps() 以及 CSS 进行样式设置的另一个 React 应用示例。这个应用是一个简单的“用户个人资料”组件,显示用户的姓名和年龄。
UserProfile.js
import React, { Component } from 'react'; import './UserProfile.css'; class UserProfile extends Component { constructor(props) { super(props); this.state = { name: 'John', age: 20, }; } UNSAFE_componentWillReceiveProps(nextProps) { // Update state when receiving new props if (nextProps.user !== this.props.user) { this.setState({ name: nextProps.user.name, age: nextProps.user.age, }); } } render() { return ( <div className="user-profile-container"> <p className="user-name">Name: {this.state.name}</p> <p className="user-age">Age: {this.state.age}</p> </div> ); } } export default UserProfile;
UserProfile.css
.user-profile-container { max-width: 300px; margin: 20px; padding: 16px; border: 1px solid #ddd; border-radius: 4px; box-shadow: 0 0 8px rgba(0, 0, 0, 0.1); } .user-name, .user-age { font-size: 18px; color: #333; margin: 8px 0; }
输出
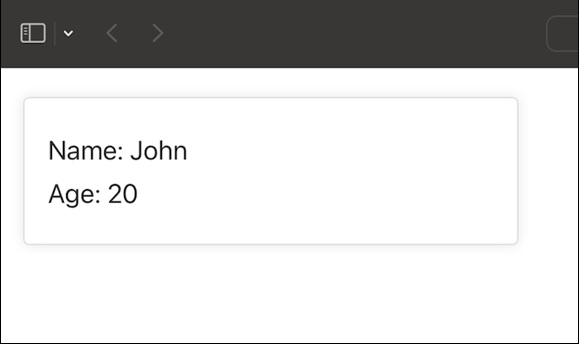
请记住,在现代 React 中,我们通常使用其他生命周期方法或 Hooks 来实现与 UNSAFE_componentWillReceiveProps 类似的功能。
总结
UNSAFE_componentWillReceiveProps 是一个旧的 React 生命周期函数,当组件将要从其父组件接收新的 props 时调用。它用于通过比较新的 props 和现有的 props 来响应 props 更改。在新的 React 代码中处理 props 更改时,最好使用替代方案以获得更安全和一致的行为。