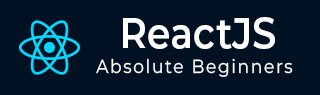
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优势与劣势
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 协调
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 行内样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - UNSAFE_componentWillUpdate() 方法
在 Web 开发中,UNSAFE_componentWillUpdate() 函数充当警告。它在我们即将修改网站一部分时出现。
请记住,此警告仅在更新任何内容时显示,而不在首次创建时显示。因此,UNSAFE_componentWillUpdate() 充当警告,帮助我们检测更新网站时出现的问题。
语法
UNSAFE_componentWillUpdate(nextProps, nextState)
参数
此方法接受两个参数:nextProps 和 nextState。
nextProps − 这些是我们的 Web 组件将获得的新特性(如信息)。它就像获得一套新的指令。
nextState − 这反映了我们组件的新状态。它类似于了解当前情况。
返回值
UNSAFE_componentWillUpdate() 不返回任何结果。它更像是进行更改之前的起点,因此它不提供结果或答案。
示例
示例 1
在这个示例中,我们将在 React 应用中使用 UNSAFE_componentWillUpdate() 函数创建一个简单的示例。
我们将有一个名为 App 的基本 React 组件。它允许我们通过点击按钮来更改页面的背景颜色。UNSAFE_componentWillUpdate() 函数将检测背景颜色的变化并相应地调整页面的背景颜色。
import React, { Component } from 'react'; class App extends Component { constructor(props) { super(props); this.state = { backgroundColor: 'white', }; } // This is the UNSAFE_componentWillUpdate() function. UNSAFE_componentWillUpdate(nextProps, nextState) { if (nextState.backgroundColor !== this.state.backgroundColor) { document.body.style.backgroundColor = nextState.backgroundColor; } } handleChangeColor = (color) => { this.setState({ backgroundColor: color }); } render() { return ( <div> <h1>Color Change App</h1> <button onClick={() => this.handleChangeColor('red')}>Change to Red</button> <button onClick={() => this.handleChangeColor('blue')}>Change to Blue</button> <button onClick={() => this.handleChangeColor('green')}>Change to Green</button> </div> ); } } export default App;
输出
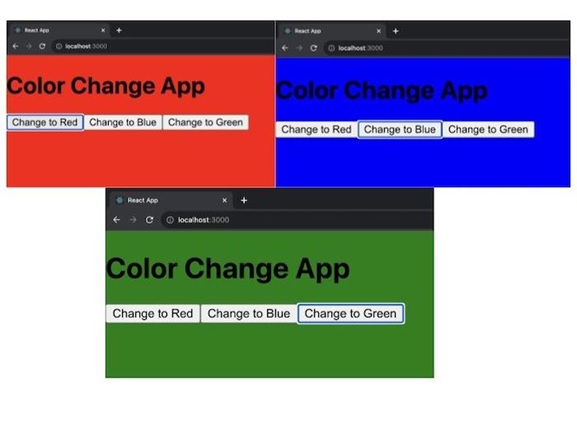
示例 2
在这个示例中,我们将使用 UNSAFE_componentWillUpdate() 函数将温度从摄氏度更新为华氏度。我们可以输入摄氏度或华氏度的温度,程序将立即调整另一个温度值。例如,如果我们输入摄氏度温度,应用程序将显示相应的华氏度温度,反之亦然。当我们输入新的温度时,它使用 UNSAFE_componentWillUpdate() 方法处理转换。
以下是相同的代码:
// TempConverterApp.js import React, { Component } from 'react'; import './App.css'; class TempConverterApp extends Component { constructor(props) { super(props); this.state = { celsius: 0, fahrenheit: 32, }; } // Usage of UNSAFE_componentWillUpdate() to update the temperature values UNSAFE_componentWillUpdate(nextProps, nextState) { if (nextState.celsius !== this.state.celsius) { // Convert Celsius to Fahrenheit nextState.fahrenheit = (nextState.celsius * 9) / 5 + 32; } else if (nextState.fahrenheit !== this.state.fahrenheit) { // Convert Fahrenheit to Celsius nextState.celsius = ((nextState.fahrenheit - 32) * 5) / 9; } } handleChangeCelsius = (value) => { this.setState({ celsius: value }); }; handleChangeFahrenheit = (value) => { this.setState({ fahrenheit: value }); }; render() { return ( <div className='App'> <h1>Temperature Converter</h1> <label> Celsius: <input type="number" value={this.state.celsius} onChange={(e) => this.handleChangeCelsius(e.target.value)} /> </label> <br /> <label> Fahrenheit: <input type="number" value={this.state.fahrenheit} onChange={(e) => this.handleChangeFahrenheit(e.target.value)} /> </label> </div> ); } } export default TempConverterApp;
输出
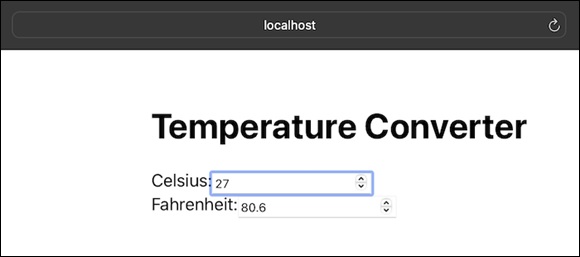
普通文本:用于普通文本。
示例 3
此应用程序是一个简单的计数器应用程序,可以向上和向下计数。有两个按钮:一个用于增加计数,另一个用于减少计数。程序还将告诉我们当前计数是奇数还是偶数。它通过调用 UNSAFE_componentWillUpdate() 方法来更新基于计数是奇数还是偶数的消息。如果我们有一个偶数,它将显示“偶数计数”,如果我们有一个奇数,它将显示“奇数计数”。
因此,此应用程序的代码如下:
// CounterApp.js import React, { Component } from 'react'; import './App.css'; class CounterApp extends Component { constructor(props) { super(props); this.state = { count: 0, message: '', }; } // Usage of UNSAFE_componentWillUpdate() to update the message based on the count value UNSAFE_componentWillUpdate(nextProps, nextState) { if (nextState.count !== this.state.count) { nextState.message = nextState.count % 2 === 0 ? 'Even Count' : 'Odd Count'; } } handleIncrement = () => { this.setState((prevState) => ({ count: prevState.count + 1 })); }; handleDecrement = () => { this.setState((prevState) => ({ count: prevState.count - 1 })); }; render() { return ( <div className='App'> <h1>Counter App</h1> <p>{this.state.message}</p> <button onClick={this.handleIncrement}>Increment</button> <button onClick={this.handleDecrement}>Decrement</button> <p>Count: {this.state.count}</p> </div> ); } } export default CounterApp;
输出

注意
因此,我们可以看到上面所示的输出图像中,有两个按钮可用:一个用于递增,另一个用于递减。并且根据奇数和偶数计数显示消息。
总结
UNSAFE_componentWillUpdate() 是一个 React 函数,它在组件更新和重新渲染之前不久使用。它使我们有机会为将来的更改做好准备。