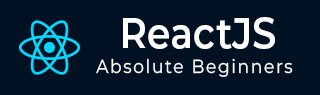
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 碎片
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - WheelEvent 处理程序
在 React JS 中,WheelEvent 处理程序函数用于响应鼠标滚轮移动。这是一种在用户使用鼠标滚动时使我们的 React 应用程序具有交互性的方法。
鼠标滚轮交互在在线应用程序中很常见,主要是在滚动内容时。因此,我们将了解 WheelEvent 接口以及如何在 React 应用程序中使用它来处理鼠标滚轮事件。WheelEvent 接口显示用户移动鼠标滚轮或类似输入设备时发生的事件。它提供了有关滚动操作的有用信息。
语法
<div onWheel={e => console.log('onWheel')} />
请记住,将 onWheel 事件附加到我们想要使其可滚动的元素上。这只是一个基本的介绍,我们可以根据我们的具体需求自定义处理程序函数。
参数
e - 它是一个 React 事件对象。我们可以将其与 WheelEvent 属性一起使用。
WheelEvent 的属性
序号 | 属性和描述 | 数据类型 |
---|---|---|
1 | deltaX 水平滚动量 |
双精度 |
2 | deltaY 垂直滚动量 |
双精度 |
3 | deltaZ z 轴的滚动量 |
双精度 |
4 | deltaMode delta* 值的滚动量的单位 |
无符号长整数 |
示例
示例 - 带有 Wheel Event 处理程序的简单应用程序
现在让我们创建一个小的 React 应用程序来展示如何在应用程序中使用 WheelEvent。使用 WheelEvent 接口,我们将创建一个跟踪滚动事件的组件。代码如下所示:
import React from 'react'; class App extends React.Component { handleWheelEvent = (e) => { console.log('onWheel'); console.log('deltaX:', e.deltaX); console.log('deltaY:', e.deltaY); console.log('deltaZ:', e.deltaZ); console.log('deltaMode:', e.deltaMode); }; render() { return ( <div onWheel={this.handleWheelEvent}> <h1>Mouse WheelEvent Logger</h1> <p>Scroll mouse wheel and check the console for event details.</p> </div> ); } } export default App;
输出
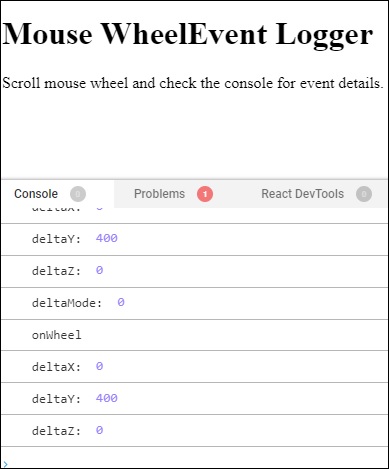
在此组件中,我们创建了一个 onWheel 事件处理程序,它记录有关滚轮事件的信息,例如 deltaX、deltaY、deltaZ 和 deltaMode,如我们在输出中看到的。
示例 - 滚动组件应用程序
在此示例中,当用户使用鼠标滚轮滚动时,会触发 handleScroll 函数。event.deltaY 为我们提供了有关滚动方向和强度的信息。
import React from 'react'; class ScrollComponent extends React.Component { handleScroll = (event) => { // This function will be called when the user scrolls console.log('Mouse wheel moved!', event.deltaY); }; render() { return ( <div onWheel={this.handleScroll}> <p>Scroll me with the mouse wheel!</p> </div> ); } } export default ScrollComponent;
输出
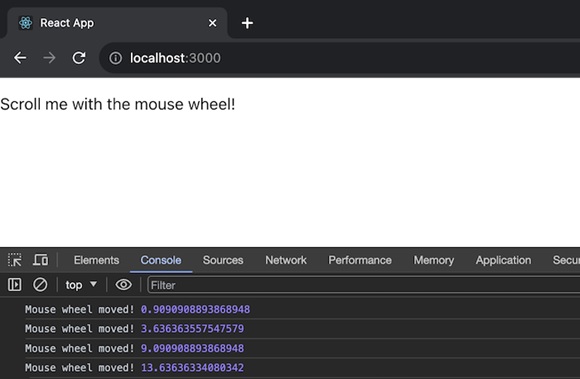
示例 - 滚动时更改背景颜色
这是另一个使用 WheelEvent 处理程序函数的简单 React 应用程序。这次,我们将创建一个应用程序,其中背景颜色根据鼠标滚轮滚动的方向而变化:
import React, { useState } from 'react'; const ScrollColorChangeApp = () => { const [backgroundColor, setBackgroundColor] = useState('lightblue'); const handleScroll = (event) => { // Change the background color based on the scroll direction if (event.deltaY > 0) { setBackgroundColor('lightgreen'); } else { setBackgroundColor('lightcoral'); } }; return ( <div onWheel={handleScroll} style={{ height: '100vh', display: 'flex', alignItems: 'center', backgroundColor: backgroundColor, transition: 'background-color 0.5s ease', }} > <h1>Scroll to Change Background Color</h1> </div> ); }; export default ScrollColorChangeApp;
输出
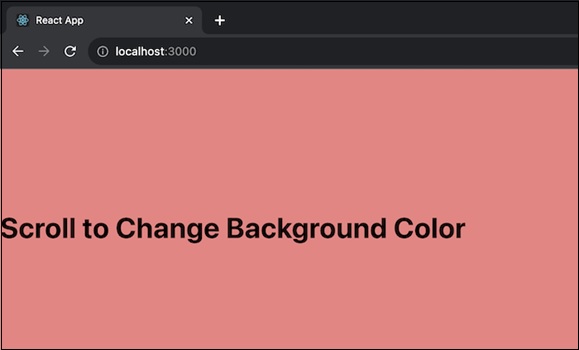
总结
WheelEvent 接口在 React 应用程序中用于处理鼠标滚轮事件非常有用。它提供了有关用户滚动行为的有用信息,例如滚动方向和强度。通过使用 WheelEvent 接口,我们可以创建更动态和响应式的 Web 应用程序,从而改善用户体验。