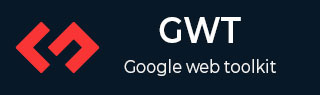
- GWT 教程
- GWT - 首页
- GWT - 概述
- GWT - 环境搭建
- GWT - 应用
- GWT - 创建应用
- GWT - 部署应用
- GWT - 使用 CSS 样式
- GWT - 基本组件
- GWT - 表单组件
- GWT - 复杂组件
- GWT - 布局面板
- GWT - 事件处理
- GWT - 自定义组件
- GWT - UIBinder
- GWT - RPC 通信
- GWT - JUnit 集成
- GWT - 调试应用
- GWT - 国际化
- GWT - History 类
- GWT - 书签支持
- GWT - 日志框架
- GWT 有用资源
- GWT - 问答
- GWT - 快速指南
- GWT - 有用资源
- GWT - 讨论
GWT - FormPanel 组件
介绍
FormPanel 组件表示一个面板,它将其内容包装在 HTML <FORM> 元素中。
类声明
以下是 com.google.gwt.user.client.ui.FormPanel 类的声明:
public class FormPanel extends SimplePanel implements FiresFormEvents, com.google.gwt.user.client.ui.impl.FormPanelImplHost
类构造函数
序号 | 构造函数 & 描述 |
---|---|
1 |
FormPanel() 创建一个新的 FormPanel。 |
2 |
protected FormPanel(Element element) 子类可以使用此构造函数显式使用现有元素。 |
3 |
protected FormPanel(Element element, boolean createIFrame) 子类可以使用此构造函数显式使用现有元素。 |
4 |
FormPanel(NamedFrame frameTarget) 创建一个目标为 NamedFrame 的 FormPanel。 |
5 |
FormPanel(java.lang.String target) 创建一个新的 FormPanel。 |
类方法
序号 | 函数名称 & 描述 |
---|---|
1 |
void addFormHandler(FormHandler handler) 已弃用。请改用 addSubmitCompleteHandler(com.google.gwt.user.client.ui.FormPanel.SubmitCompleteHandler) 和 addSubmitHandler(com.google.gwt.user.client.ui.FormPanel.SubmitHandler)。 |
2 |
HandlerRegistration addSubmitCompleteHandler(FormPanel.SubmitCompleteHandler handler) 添加 FormPanel.SubmitComplete 事件处理程序。 |
3 |
HandlerRegistration addSubmitHandler(FormPanel.SubmitHandler handler) 添加 FormPanel.Submit 事件处理程序。 |
4 |
java.lang.String getAction() 获取与此表单关联的“action”。 |
5 |
java.lang.String getEncoding() 获取用于提交此表单的编码。 |
6 |
java.lang.String getMethod() 获取用于提交此表单的 HTTP 方法。 |
7 |
java.lang.String getTarget() 获取表单的“target”。 |
8 |
protected void onAttach() 当小部件附加到浏览器的文档时,将调用此方法。 |
9 |
protected void onDetach() 当小部件从浏览器的文档中分离时,将调用此方法。 |
10 |
boolean onFormSubmit() 提交表单时触发。 |
11 |
void onFrameLoad() |
12 |
void removeFormHandler(FormHandler handler) 已弃用。请改用 add*Handler 方法返回的对象上的 HandlerRegistration.removeHandler() 方法。 |
13 |
void reset() 重置表单,清除所有字段。 |
14 |
void setAction(java.lang.String url) 设置与此表单关联的“action”。 |
15 |
void setEncoding(java.lang.String encodingType) 设置用于提交此表单的编码。 |
16 |
void setMethod(java.lang.String method) 设置用于提交此表单的 HTTP 方法。 |
17 |
void submit() 提交表单。 |
18 |
static FormPanel wrap(Element element) 创建一个包装现有 <form> 元素的 FormPanel。 |
19 |
static FormPanel wrap(Element element, boolean createIFrame) 创建一个包装现有 <form> 元素的 FormPanel。 |
继承的方法
此类继承自以下类的方法:
com.google.gwt.user.client.ui.UIObject
com.google.gwt.user.client.ui.Widget
com.google.gwt.user.client.ui.Panel
com.google.gwt.user.client.ui.SimplePanel
java.lang.Object
FormPanel 组件示例
本示例将引导您完成简单的步骤,以演示在 GWT 中使用 FormPanel 组件的方法。请按照以下步骤更新我们在GWT - 创建应用章节中创建的 GWT 应用程序:
步骤 | 描述 |
---|---|
1 | 按照GWT - 创建应用章节中的说明,创建一个名为HelloWorld的项目,放在com.tutorialspoint包下。 |
2 | 修改HelloWorld.gwt.xml、HelloWorld.css、HelloWorld.html和HelloWorld.java,如下所述。其余文件保持不变。 |
3 | 编译并运行应用程序以验证已实现逻辑的结果。 |
以下是修改后的模块描述符src/com.tutorialspoint/HelloWorld.gwt.xml的内容。
<?xml version = "1.0" encoding = "UTF-8"?> <module rename-to = 'helloworld'> <!-- Inherit the core Web Toolkit stuff. --> <inherits name = 'com.google.gwt.user.User'/> <!-- Inherit the default GWT style sheet. --> <inherits name = 'com.google.gwt.user.theme.clean.Clean'/> <!-- Specify the app entry point class. --> <entry-point class = 'com.tutorialspoint.client.HelloWorld'/> <!-- Specify the paths for translatable code --> <source path = 'client'/> <source path = 'shared'/> </module>
以下是修改后的样式表文件war/HelloWorld.css的内容。
body { text-align: center; font-family: verdana, sans-serif; } h1 { font-size: 2em; font-weight: bold; color: #777777; margin: 40px 0px 70px; text-align: center; }
以下是修改后的 HTML 宿主文件war/HelloWorld.html的内容。
<html> <head> <title>Hello World</title> <link rel = "stylesheet" href = "HelloWorld.css"/> <script language = "javascript" src = "helloworld/helloworld.nocache.js"> </script> </head> <body> <h1>FormPanel Widget Demonstration</h1> <div id = "gwtContainer"></div> </body> </html>
让我们看看 Java 文件src/com.tutorialspoint/HelloWorld.java的内容,它将演示 FormPanel 组件的使用。
package com.tutorialspoint.client; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.event.dom.client.ClickEvent; import com.google.gwt.event.dom.client.ClickHandler; import com.google.gwt.user.client.Window; import com.google.gwt.user.client.ui.Button; import com.google.gwt.user.client.ui.DecoratorPanel; import com.google.gwt.user.client.ui.FileUpload; import com.google.gwt.user.client.ui.FormPanel; import com.google.gwt.user.client.ui.FormPanel.SubmitCompleteEvent; import com.google.gwt.user.client.ui.FormPanel.SubmitEvent; import com.google.gwt.user.client.ui.ListBox; import com.google.gwt.user.client.ui.RootPanel; import com.google.gwt.user.client.ui.TextBox; import com.google.gwt.user.client.ui.VerticalPanel; public class HelloWorld implements EntryPoint { public void onModuleLoad() { // Create a FormPanel and point it at a service. final FormPanel form = new FormPanel(); form.setAction("/myFormHandler"); // Because we're going to add a FileUpload widget, // we'll need to set the form to use the POST method, // and multipart MIME encoding. form.setEncoding(FormPanel.ENCODING_MULTIPART); form.setMethod(FormPanel.METHOD_POST); // Create a panel to hold all of the form widgets. VerticalPanel panel = new VerticalPanel(); panel.setSpacing(10); form.setWidget(panel); // Create a TextBox, giving it a name so that it will be submitted. final TextBox tb = new TextBox(); tb.setWidth("220"); tb.setName("textBoxFormElement"); panel.add(tb); // Create a ListBox, giving it a name and // some values to be associated with its options. ListBox lb = new ListBox(); lb.setName("listBoxFormElement"); lb.addItem("item1", "item1"); lb.addItem("item2", "item2"); lb.addItem("item3", "item3"); lb.setWidth("220"); panel.add(lb); // Create a FileUpload widget. FileUpload upload = new FileUpload(); upload.setName("uploadFormElement"); panel.add(upload); // Add a 'submit' button. panel.add(new Button("Submit", new ClickHandler() { @Override public void onClick(ClickEvent event) { form.submit(); } })); // Add an event handler to the form. form.addSubmitHandler(new FormPanel.SubmitHandler() { @Override public void onSubmit(SubmitEvent event) { // This event is fired just before the form is submitted. // We can take this opportunity to perform validation. if (tb.getText().length() == 0) { Window.alert("The text box must not be empty"); event.cancel(); } } }); form.addSubmitCompleteHandler(new FormPanel.SubmitCompleteHandler() { @Override public void onSubmitComplete(SubmitCompleteEvent event) { // When the form submission is successfully completed, // this event is fired. Assuming the service returned // a response of type text/html, we can get the result // here. Window.alert(event.getResults()); } }); DecoratorPanel decoratorPanel = new DecoratorPanel(); decoratorPanel.add(form); // Add the widgets to the root panel. RootPanel.get().add(decoratorPanel); } }
完成所有更改后,让我们像在GWT - 创建应用章节中一样,在开发模式下编译并运行应用程序。如果您的应用程序一切正常,则会产生以下结果:
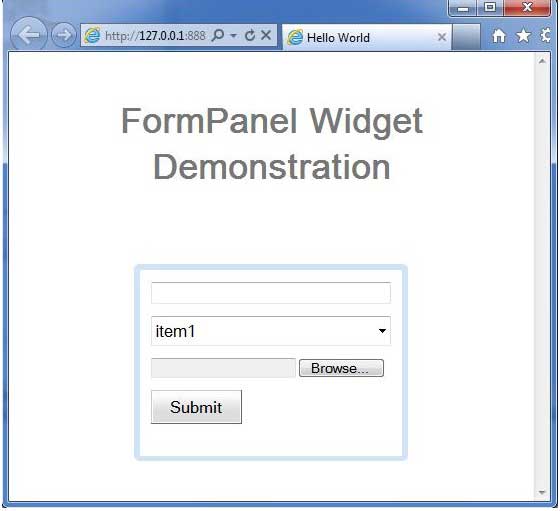