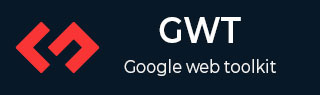
- GWT 教程
- GWT - 首页
- GWT - 概述
- GWT - 环境搭建
- GWT - 应用
- GWT - 创建应用
- GWT - 部署应用
- GWT - 使用 CSS 样式
- GWT - 基本控件
- GWT - 表单控件
- GWT - 复杂控件
- GWT - 布局面板
- GWT - 事件处理
- GWT - 自定义控件
- GWT - UIBinder
- GWT - RPC 通信
- GWT - JUnit 集成
- GWT - 调试应用
- GWT - 国际化
- GWT - History 类
- GWT - 书签支持
- GWT - 日志框架
- GWT 有用资源
- GWT - 问答
- GWT - 快速指南
- GWT - 有用资源
- GWT - 讨论
GWT - ListBox 控件
简介
ListBox 控件向用户呈现一系列选择项,可以是列表框或下拉列表。
类声明
以下是 com.google.gwt.user.client.ui.ListBox 类的声明:
public class ListBox extends FocusWidget implements SourcesChangeEvents, HasName
CSS 样式规则
以下默认 CSS 样式规则将应用于所有 ListBox 控件。您可以根据需要覆盖它。
.gwt-ListBox {}
类构造函数
序号 | 构造函数 & 描述 |
---|---|
1 |
ListBox() 以单选模式创建一个空的列表框。 |
2 |
ListBox(boolean isMultipleSelect) 创建一个空的列表框。 |
3 |
ListBox(Element element) 子类可以使用此构造函数显式使用现有元素。 |
类方法
序号 | 函数名称 & 描述 |
---|---|
1 |
void addItem(java.lang.String item) 向列表框添加一个项目。 |
2 |
void addItem(java.lang.String item, java.lang.String value) 向列表框添加一个项目,并为该项目指定一个初始值。 |
3 |
void clear() 移除列表框中的所有项目。 |
4 |
int getItemCount() 获取列表框中存在的项目数。 |
5 |
java.lang.String getItemText(int index) 获取与指定索引处的项目关联的文本。 |
6 |
java.lang.String getName() 获取控件的名称。 |
7 |
int getSelectedIndex() 获取当前选定的项目。 |
8 |
java.lang.String getValue(int index) 获取与给定索引处的项目关联的值。 |
9 |
int getVisibleItemCount() 获取可见的项目数。 |
10 |
void insertItem(java.lang.String item, int index) 将一个项目插入列表框。 |
11 |
void insertItem(java.lang.String item, java.lang.String value, int index) 将一个项目插入列表框,并为该项目指定一个初始值。 |
12 |
boolean isItemSelected(int index) 确定某个列表项是否被选中。 |
13 |
boolean isMultipleSelect() 获取此列表是否允许多选。 |
14 |
void onBrowserEvent(Event event) 每当接收到浏览器事件时触发。 |
15 |
protected void onEnsureDebugId(java.lang.String baseID) 受影响的元素:-item# = 指定索引处的选项。 |
16 |
void removeChangeListener(ChangeListener listener) 移除之前添加的监听器接口。 |
17 |
void removeItem(int index) 移除指定索引处的项目。 |
18 |
void setItemSelected(int index, boolean selected) 设置某个列表项是否被选中。 |
19 |
void setItemText(int index,java.lang.String text) 设置给定索引处的文本。 |
20 |
void setMultipleSelect(boolean multiple) 设置此列表是否允许多选。 |
21 |
void setName(java.lang.String name) 设置控件的名称。 |
22 |
void setSelectedIndex(int index) 设置当前选定的索引。 |
23 |
void setValue(int index, java.lang.String value) 设置与给定索引处的项目关联的值。 |
24 |
void setVisibleItemCount(int visibleItems) 设置可见的项目数。 |
25 |
static ListBox wrap(Element element) 创建一个包装现有 <select> 元素的 ListBox 控件。 |
26 |
void addChangeListener(ChangeListener listener) 添加一个监听器接口以接收更改事件。 |
继承的方法
此类继承自以下类的方法:
com.google.gwt.user.client.ui.UIObject
com.google.gwt.user.client.ui.Widget
com.google.gwt.user.client.ui.FocusWidget
java.lang.Object
ListBox 控件示例
此示例将引导您完成简单的步骤,以演示如何在 GWT 中使用 ListBox 控件。按照以下步骤更新我们在GWT - 创建应用章节中创建的 GWT 应用:
步骤 | 描述 |
---|---|
1 | 在GWT - 创建应用章节中说明的包com.tutorialspoint下创建一个名为HelloWorld的项目。 |
2 | 修改HelloWorld.gwt.xml、HelloWorld.css、HelloWorld.html和HelloWorld.java,如下所述。保持其余文件不变。 |
3 | 编译并运行应用程序以验证已实现逻辑的结果。 |
以下是修改后的模块描述符src/com.tutorialspoint/HelloWorld.gwt.xml的内容。
<?xml version = "1.0" encoding = "UTF-8"?> <module rename-to = 'helloworld'> <!-- Inherit the core Web Toolkit stuff. --> <inherits name = 'com.google.gwt.user.User'/> <!-- Inherit the default GWT style sheet. --> <inherits name = 'com.google.gwt.user.theme.clean.Clean'/> <!-- Specify the app entry point class. --> <entry-point class = 'com.tutorialspoint.client.HelloWorld'/> <!-- Specify the paths for translatable code --> <source path = 'client'/> <source path = 'shared'/> </module>
以下是修改后的样式表文件war/HelloWorld.css的内容。
body { text-align: center; font-family: verdana, sans-serif; } h1 { font-size: 2em; font-weight: bold; color: #777777; margin: 40px 0px 70px; text-align: center; } .gwt-ListBox{ color:green; }
以下是修改后的 HTML 宿主文件war/HelloWorld.html的内容。
<html> <head> <title>Hello World</title> <link rel = "stylesheet" href = "HelloWorld.css"/> <script language = "javascript" src = "helloworld/helloworld.nocache.js"> </script> </head> <body> <h1>ListBox Widget Demonstration</h1> <div id = "gwtContainer"></div> </body> </html>
让我们看看 Java 文件src/com.tutorialspoint/HelloWorld.java的内容,它将演示 ListBox 控件的使用。
package com.tutorialspoint.client; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.user.client.ui.ListBox; import com.google.gwt.user.client.ui.RootPanel; import com.google.gwt.user.client.ui.VerticalPanel; public class HelloWorld implements EntryPoint { public void onModuleLoad() { // Make a new list box, adding a few items to it. ListBox listBox1 = new ListBox(); listBox1.addItem("First"); listBox1.addItem("Second"); listBox1.addItem("Third"); listBox1.addItem("Fourth"); listBox1.addItem("Fifth"); // Make a new list box, adding a few items to it. ListBox listBox2 = new ListBox(); listBox2.addItem("First"); listBox2.addItem("Second"); listBox2.addItem("Third"); listBox2.addItem("Fourth"); listBox2.addItem("Fifth"); // Make enough room for all five items listBox1.setVisibleItemCount(5); //setting itemcount value to 1 turns listbox into a drop-down list. listBox2.setVisibleItemCount(1); // Add listboxes to the root panel. VerticalPanel panel = new VerticalPanel(); panel.setSpacing(10); panel.add(listBox1); panel.add(listBox2); RootPanel.get("gwtContainer").add(panel); } }
完成所有更改后,让我们像在GWT - 创建应用章节中一样,在开发模式下编译并运行应用程序。如果您的应用程序一切正常,这将产生以下结果:
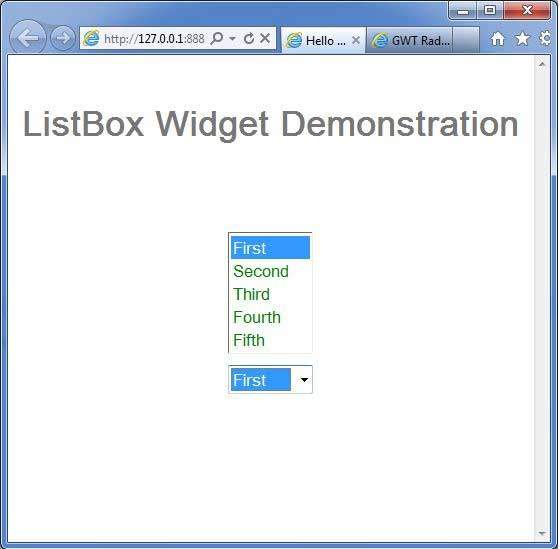