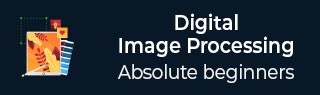
- Java 数字图像处理
- 数字图像处理 - 首页
- 数字图像处理 - 简介
- 数字图像处理 - Java BufferedImage 类
- 数字图像处理 - 图像下载和上传
- 数字图像处理 - 图像像素
- 数字图像处理 - 灰度转换
- 数字图像处理 - 增强图像对比度
- 数字图像处理 - 增强图像亮度
- 数字图像处理 - 增强图像锐度
- 数字图像处理 - 图像压缩技术
- 数字图像处理 - 添加图像边框
- 数字图像处理 - 图像金字塔
- 数字图像处理 - 基本阈值化
- 数字图像处理 - 图像形状转换
- 数字图像处理 - 高斯滤波器
- 数字图像处理 - 盒式滤波器
- 数字图像处理 - 腐蚀和膨胀
- 数字图像处理 - 水印
- 数字图像处理 - 卷积理解
- 数字图像处理 - Prewitt算子
- 数字图像处理 - Sobel算子
- 数字图像处理 - Kirsch算子
- 数字图像处理 - Robinson算子
- 数字图像处理 - Laplacian算子
- 数字图像处理 - 加权平均滤波器
- 数字图像处理 - 创建缩放效果
- 数字图像处理 - 开源库
- 数字图像处理 - OpenCV简介
- 数字图像处理 - OpenCV灰度转换
- 数字图像处理 - 颜色空间转换
- 数字图像处理 有用资源
- 数字图像处理 - 快速指南
- 数字图像处理 - 有用资源
- 数字图像处理 - 讨论
Java 数字图像处理 - 基本阈值化
阈值化能够以最简单的方式实现图像分割。图像分割是指将完整图像划分为一组像素,使得每组像素具有某些共同特征。图像分割在定义对象及其边界方面非常有用。
在本节中,我们将对图像执行一些基本的阈值化操作。
我们使用OpenCV函数threshold。它可以在Imgproc包中找到。其语法如下所示:
Imgproc.threshold(source, destination, thresh , maxval , type);
参数说明如下:
序号 | 参数及描述 |
---|---|
1 |
source 源图像。 |
2 |
destination 目标图像。 |
3 |
thresh 阈值。 |
4 |
maxval 对于THRESH_BINARY和THRESH_BINARY_INV阈值类型,使用的最大值。 |
5 |
type 可能的类型包括THRESH_BINARY、THRESH_BINARY_INV、THRESH_TRUNC和THRESH_TOZERO。 |
除了这些阈值化方法外,Imgproc类还提供了其他方法。简要描述如下:
序号 | 方法及描述 |
---|---|
1 |
cvtColor(Mat src, Mat dst, int code, int dstCn) 将图像从一个颜色空间转换为另一个颜色空间。 |
2 |
dilate(Mat src, Mat dst, Mat kernel) 使用特定的结构元素膨胀图像。 |
3 |
equalizeHist(Mat src, Mat dst) 均衡灰度图像的直方图。 |
4 |
filter2D(Mat src, Mat dst, int ddepth, Mat kernel, Point anchor, double delta) 将图像与内核进行卷积。 |
5 |
GaussianBlur(Mat src, Mat dst, Size ksize, double sigmaX) 使用高斯滤波器模糊图像。 |
6 |
integral(Mat src, Mat sum) 计算图像的积分。 |
示例
以下示例演示了如何使用Imgproc类对图像执行阈值化操作:
import org.opencv.core.Core; import org.opencv.core.CvType; import org.opencv.core.Mat; import org.opencv.highgui.Highgui; import org.opencv.imgproc.Imgproc; public class main { public static void main( String[] args ) { try{ System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); Mat source = Highgui.imread("digital_image_processing.jpg", Highgui.CV_LOAD_IMAGE_COLOR); Mat destination = new Mat(source.rows(),source.cols(),source.type()); destination = source; Imgproc.threshold(source,destination,127,255,Imgproc.THRESH_TOZERO); Highgui.imwrite("ThreshZero.jpg", destination); } catch (Exception e) { System.out.println("error: " + e.getMessage()); } } }
输出
执行给定代码后,将看到以下输出:
原始图像
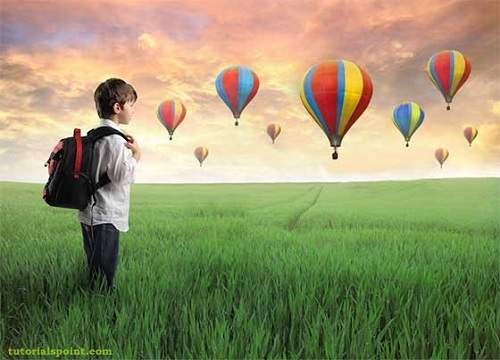
在上面的原始图像上,执行了一些阈值化操作,输出如下所示:
二值阈值
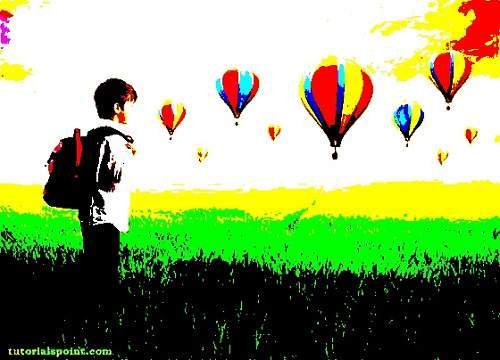
反二值阈值
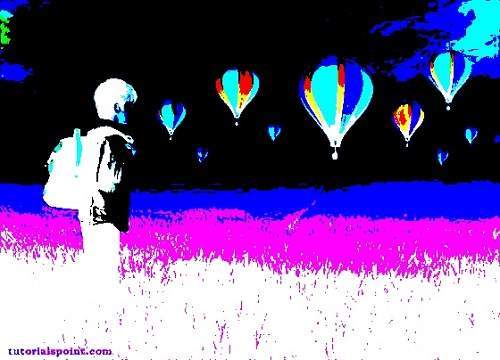
截断阈值
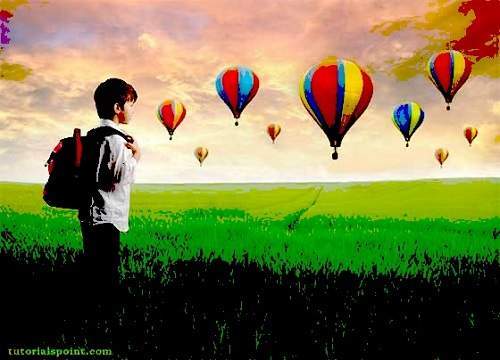