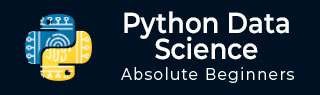
- Python 数据科学教程
- Python 数据科学 - 首页
- Python 数据科学 - 快速入门
- Python 数据科学 - 环境设置
- Python 数据科学 - Pandas
- Python 数据科学 - NumPy
- Python 数据科学 - SciPy
- Python 数据科学 - Matplotlib
- Python 数据处理
- Python 数据操作
- Python 数据清洗
- Python 处理 CSV 数据
- Python 处理 JSON 数据
- Python 处理 XLS 数据
- Python 关系型数据库
- Python NoSQL 数据库
- Python 日期和时间
- Python 数据整理
- Python 数据聚合
- Python 读取 HTML 页面
- Python 处理非结构化数据
- Python 词元化
- Python 词干提取和词形还原
- Python 数据可视化
- Python 图表属性
- Python 图表样式
- Python 箱线图
- Python 热力图
- Python 散点图
- Python 气泡图
- Python 3D 图表
- Python 时间序列
- Python 地理数据
- Python 图数据
Python - NoSQL 数据库
随着越来越多的数据以非结构化或半结构化的形式出现,通过 NoSQL 数据库管理这些数据的需求日益增长。Python 可以像与关系型数据库交互一样,以类似的方式与 NoSQL 数据库交互。本章将使用 Python 与 MongoDB(作为 NoSQL 数据库)进行交互。如果您不熟悉 MongoDB,可以在我们的教程这里学习。
为了连接到 MongoDB,Python 使用一个名为pymongo的库。您可以使用 Anaconda 环境中的以下命令将此库添加到您的 Python 环境中。
conda install pymongo
此库使 Python 能够使用数据库客户端连接到 MongoDB。连接后,我们选择要用于各种操作的数据库名称。
插入数据
要将数据插入 MongoDB,我们使用数据库环境中提供的 insert() 方法。首先,我们使用下面所示的 Python 代码连接到数据库,然后我们以一系列键值对的形式提供文档详细信息。
# Import the python libraries from pymongo import MongoClient from pprint import pprint # Choose the appropriate client client = MongoClient() # Connect to the test db db=client.test # Use the employee collection employee = db.employee employee_details = { 'Name': 'Raj Kumar', 'Address': 'Sears Streer, NZ', 'Age': '42' } # Use the insert method result = employee.insert_one(employee_details) # Query for the inserted document. Queryresult = employee.find_one({'Age': '42'}) pprint(Queryresult)
执行上述代码后,将产生以下结果。
{u'Address': u'Sears Streer, NZ', u'Age': u'42', u'Name': u'Raj Kumar', u'_id': ObjectId('5adc5a9f84e7cd3940399f93')}
更新数据
更新现有的 MongoDB 数据类似于插入数据。我们使用 MongoDB 自带的 update() 方法。在下面的代码中,我们用新的键值对替换现有记录。请注意我们如何使用条件标准来决定要更新哪个记录。
# Import the python libraries from pymongo import MongoClient from pprint import pprint # Choose the appropriate client client = MongoClient() # Connect to db db=client.test employee = db.employee # Use the condition to choose the record # and use the update method db.employee.update_one( {"Age":'42'}, { "$set": { "Name":"Srinidhi", "Age":'35', "Address":"New Omsk, WC" } } ) Queryresult = employee.find_one({'Age':'35'}) pprint(Queryresult)
执行上述代码后,将产生以下结果。
{u'Address': u'New Omsk, WC', u'Age': u'35', u'Name': u'Srinidhi', u'_id': ObjectId('5adc5a9f84e7cd3940399f93')}
删除数据
删除记录也很直接,我们使用 delete 方法。在这里,我们也提到了用于选择要删除的记录的条件。
# Import the python libraries from pymongo import MongoClient from pprint import pprint # Choose the appropriate client client = MongoClient() # Connect to db db=client.test employee = db.employee # Use the condition to choose the record # and use the delete method db.employee.delete_one({"Age":'35'}) Queryresult = employee.find_one({'Age':'35'}) pprint(Queryresult)
执行上述代码后,将产生以下结果。
None
因此,我们看到该特定记录不再存在于数据库中。
广告