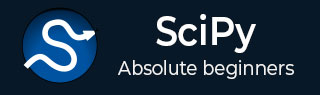
- SciPy 教程
- SciPy - 首页
- SciPy - 介绍
- SciPy - 环境设置
- SciPy - 基本功能
- SciPy - 集群
- SciPy - 常量
- SciPy - FFTpack
- SciPy - 整合
- SciPy - 插值
- SciPy - 输入和输出
- SciPy - 线性代数
- SciPy - Nd 图像
- SciPy - 优化
- SciPy - 统计
- SciPy - CSGraph
- SciPy - 空间
- SciPy - ODR
- SciPy - 特殊包
- SciPy 的有用资源
- SciPy - 参考
- SciPy - 快速指南
- SciPy - 有用资源
- SciPy - 讨论
SciPy - convert_temperature()方法
SciPy convert_temperature()方法用于计算任何刻度形式的温标,例如摄氏度、开尔文、华氏度和兰金。
以下是各种刻度的关键理解 −
- 摄氏度 − 温度单位。
- 华氏度 − 这是温度当水在 32 度时结冰并在 212 度时沸腾的刻度。
- 开尔文 − 这是温度为 0 时反射的刻度。
- 兰金 − 绝对温度单位。
语法
以下是 SciPy convert_temperature() 方法的语法 −
convert_temperature(val, old_scale, new_scale)
参数
此方法接受以下参数 −
- val− 指定温度范围值。
- old_scale− 指定原始字符串。
- new_scale− 指定将温度转换为其中的新字符串。
返回值
此函数返回浮点值数组。
示例 1
以下是基本 SciPy convert_temperature() 方法,说明如何转换摄氏度、华氏度和开尔文。
import scipy.constants as const def convert_temperature(value, from_unit, to_unit): if from_unit == to_unit: return value # Convert the input temperature to Celsius first if from_unit == 'Celsius': temp_c = value elif from_unit == 'Fahrenheit': temp_c = (value - 32) * 5/9 elif from_unit == 'Kelvin': temp_c = value - const.zero_Celsius else: raise ValueError("Unsupported temperature unit") # Convert from Celsius to the desired unit if to_unit == 'Celsius': return temp_c elif to_unit == 'Fahrenheit': return temp_c * 9/5 + 32 elif to_unit == 'Kelvin': return temp_c + const.zero_Celsius else: raise ValueError("Unsupported temperature unit") # Show the result print(convert_temperature(100, 'Celsius', 'Fahrenheit')) print(convert_temperature(32, 'Fahrenheit', 'Celsius')) print(convert_temperature(273, 'Kelvin', 'Celsius'))
输出
以上代码将产生以下结果: −
212.0 0.0 -0.14999999999997726
示例 2
在下面的示例中,使用更多 scipy 常量将基本单位转换为温度单位。
import scipy.constants as const def convert_temperature(value, from_unit, to_unit): if from_unit == to_unit: return value # convert input to Kelvin if from_unit == 'Celsius': temp_k = value + const.zero_Celsius elif from_unit == 'Fahrenheit': temp_k = (value - 32) * 5/9 + const.zero_Celsius elif from_unit == 'Kelvin': temp_k = value else: raise ValueError("Unsupported temperature unit") # convert Kelvin to the desired unit if to_unit == 'Celsius': return temp_k - const.zero_Celsius elif to_unit == 'Fahrenheit': return (temp_k - const.zero_Celsius) * 9/5 + 32 elif to_unit == 'Kelvin': return temp_k else: raise ValueError("Unsupported temperature unit") # Show the result print(convert_temperature(100, 'Celsius', 'Fahrenheit')) print(convert_temperature(32, 'Fahrenheit', 'Kelvin')) print(convert_temperature(273.15, 'Kelvin', 'Celsius'))
输出
以上代码将产生以下结果: −
212.0 273.15 0.0
scipy_reference.htm
广告