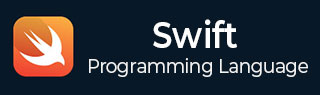
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选值
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级特性
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 断言和前提条件
Swift 提供了两种特殊的机制:断言和前提条件,允许开发者在程序中强制执行一些特殊条件,用于调试和确保程序的正确性。这两种方法通过捕获和处理应用程序开发不同阶段出现的意外情况,创建健壮可靠的 Swift 应用程序。
Swift 断言
断言是一个强大的调试工具,用于检查和修复应用程序开发过程中的错误或bug。或者可以说,其主要目的是在运行时捕获和识别错误或bug。它用布尔条件表示,如果条件计算结果为真,则程序将继续执行。而如果条件计算结果为假,则程序的执行将终止。断言是不可恢复的,这意味着如果断言失败,我们无法恢复或捕获它。我们可以使用 `assert()` 函数实现断言。
**`assert(_:_:file:line)`** 函数在开发期间执行运行时检查。或者可以说,它实现了 C 风格的断言。
语法
以下是 `assert()` 函数的语法:
func assert(_condition: @autoclosure()->Bool, _message: @autoclosure() -> String = String(), file: StaticString = #file, line: UInt = #line)
参数
此函数采用以下参数:
**`condition`** - 它表示测试条件。
**`message`** - 它表示当条件设置为假时将显示的消息。默认值为为空。
**`file`** - 它表示断言所在的文件名,如果断言失败,则会与消息一起显示。这是一个可选参数。
**`line`** - 它表示断言所在的行号,如果断言失败,则会与消息一起显示。这是一个可选参数。
示例
import Foundation func validateMarks(_ marks: Int) { // Assert that the marks is non-negative // If the given condition fails, then the program will terminated // And will display this message assert(marks >= 0, "Marks must be non-negative value") print("Marks are valid") } validateMarks(345)
输出
Marks are valid
示例
如果我们输入负数分数,则断言中的给定条件将失败,程序将终止。
import Foundation func validateMarks(_ marks: Int) { // Assert that the marks is non-negative // If the given condition fails, then the program will terminated // And will display this message assert(marks >= 0, "Marks must be non-negative value") print("Marks are valid") } validateMarks(-39)
输出
main/main.swift:8: Assertion failed: Marks must be non-negative value Current stack trace: 0 libswiftCore.so 0x00007f8870bbcdc0 _swift_stdlib_reportFatalErrorInFile + 112 1 libswiftCore.so 0x00007f887088691c <unavailable> + 1444124 2 libswiftCore.so 0x00007f8870886738 <unavailable> + 1443640 3 libswiftCore.so 0x00007f8870885220 _assertionFailure(_:_:file:line:flags:) + 419 6 swift-frontend 0x0000557401fc6b3d <unavailable> + 26479421 7 swift-frontend 0x00005574012fadb9 <unavailable> + 13061561 8 swift-frontend 0x00005574010cb4c6 <unavailable> + 10769606 9 swift-frontend 0x00005574010c79b6 <unavailable> + 10754486 ....................................................................... .................................................................... ..................................................................
Swift 前提条件
前提条件用于在代码执行时捕获和处理意外状态和假设。或者可以说,前提条件帮助开发者在应用程序的生产状态下查找问题。
它也用布尔条件表示,如果条件计算结果为真,则程序将继续执行。而如果条件计算结果为假,则程序的执行将终止。它也是不可恢复的。我们可以使用 **`precondition()`** 函数实现前提条件。
**`precondition(_:_:file:line)`** 函数用于在程序执行期间强制执行条件。
语法
以下是 `assert()` 函数的语法:
func precondition(_condition: @autoclosure()->Bool, _message: @autoclosure() -> String = String(), file: StaticString = #file, line: UInt = #line)
参数
此函数采用以下参数:
**`condition`** - 它表示测试条件。
**`message`** - 它表示当条件设置为假时将显示的消息。默认值为为空。
**`file`** - 它表示前提条件所在的文件名,如果前提条件失败,则会与消息一起显示。这是一个可选参数。
**`line`** - 它表示前提条件所在的行号,如果前提条件失败,则会与消息一起显示。这是一个可选参数。
示例
import Foundation func modulus(_ num: Int, by deno: Int) -> Int { // Ensure that the denominator is not zero before performing the modulus precondition(deno != 0, "The value of denominator must be non-zero") return num % deno } let output = modulus(19, by: 3) print("Result: \(output)")
输出
Result: 1
示例
如果我们输入零分母,则前提条件中的给定条件将失败,程序将终止。
import Foundation func modulus(_ num: Int, by deno: Int) -> Int { // Ensure that the denominator is not zero before performing the modulus precondition(deno != 0, "The value of denominator must be non-zero") return num % deno } let output = modulus(19, by: 0) print("Result: \(output)")
输出
main/main.swift:6: Precondition failed: The value of denominator must be non-zero Current stack trace: 0 libswiftCore.so 0x00007fbee7245dc0 _swift_stdlib_reportFatalErrorInFile + 112 1 libswiftCore.so 0x00007fbee6f0f91c <unavailable> + 1444124 2 libswiftCore.so 0x00007fbee6f0f738 <unavailable> + 1443640 3 libswiftCore.so 0x00007fbee6f0e220 _assertionFailure(_:_:file:line:flags:) + 419 6 swift-frontend 0x0000558e85a69b3d <unavailable> + 26479421 7 swift-frontend 0x0000558e84d9ddb9 <unavailable> + 13061561 8 swift-frontend 0x0000558e84b6e4c6 <unavailable> + 10769606 9 swift-frontend 0x0000558e84b6a9b6 <unavailable> + 10754486 ....................................................................... .................................................................... ..................................................................