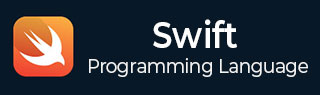
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 逃逸闭包和非逃逸闭包
- Swift - 自动闭包
- Swift 面向对象
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - if...else if...else 语句
一个if语句后面可以跟着一个可选的else if...else语句,这在使用单个if...else if语句测试各种条件时非常有用。或者我们可以说,if… else if…else语句允许你按顺序检查多个条件。
它创建了一个决策树,其中每个条件都按顺序进行评估,第一个为真的条件的语句块将被执行。如果没有任何条件为真,则 else 部分将被执行。if…else if…else 语句也称为 if… else 阶梯。
使用if、else if 和 else语句时,需要记住以下几点。
一个if可以有零个或一个 else,并且它必须出现在任何 else if 之后。
一个if可以有零到多个 else if,并且它们必须出现在 else 之前。
一旦一个else if成功,则不会测试任何剩余的else if或else。
语法
以下是 if…else if…else 语句的语法:
if boolean_expression1{ /* Execute when the boolean expression 1 is true */ } else if boolean_expression2{ /*Execute when the boolean expression 2 is true*/ } else if boolean_expression3{ /*Execute when the boolean expression 3 is true*/ }else{ /* Execure when none of the above conditions is true */ }
流程图
下面的流程图将展示 if…else if…else 语句的工作原理。
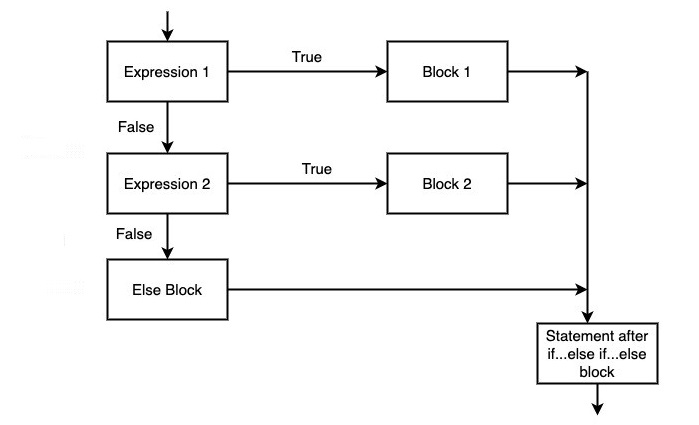
示例
Swift 程序演示if… else if…else语句的使用。
import Foundation var varA:Int = 100; /* Check the boolean condition using the if statement */ if varA == 20 { /* If the condition is true then print the following */ print("varA is equal to than 20"); } else if varA == 50 { /* If the condition is true then print the following */ print("varA is equal to than 50"); } else { /* If the condition is false then print the following */ print("None of the values is matching"); } print("Value of variable varA is \(varA)");
输出
它将产生以下输出:
None of the values is matching Value of variable varA is 100
示例
Swift 程序使用if…else阶梯检查当前季节。
import Foundation let myMonth = 5 // Checking Season using if...else ladder if myMonth >= 3 && myMonth <= 5 { print("Current Season is Spring") } else if myMonth >= 6 && myMonth <= 8 { print("Current Season is Summer") } else if myMonth >= 9 && myMonth <= 11 { print("Current Season is Autumn") } else { print("Current Season is Winter") }
输出
它将产生以下输出:
Current Season is Spring
示例
Swift 程序使用 if…else if…else 语句计算学生的成绩。
import Foundation let marks = 89 // Determine the grade of the student if marks >= 90 { print("Grade A") } else if marks >= 80 { print("Grade B") } else if marks >= 70 { print("Grade C") } else if marks >= 60 { print("Grade D") } else { print("Grade F") }
输出
它将产生以下输出:
Grade B
广告