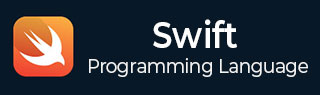
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 类型转换
什么是 Swift 中的类型转换?
类型转换是 Swift 中的一项特殊功能,用于检查实例的类型或将类、结构体或枚举的实例类型更改为另一个类、结构体或枚举。它非常重要,因为它允许在运行时进行类型检查,并安全地将实例向下转换为子类类型。
Swift 支持两个运算符:is 和 as。‘is’ 运算符用于检查值的类型,而 ‘as’ 用于将类型值转换为不同的类型。类型转换还检查实例类型是否遵循特定的协议一致性标准。
Swift 支持两种类型的类型转换:
- 向上转型
- 向下转型
Swift 中的向上转型
将子类实例转换为其基类类型的过程称为向上转型。或者我们可以说,向上转型是一个将派生类实例视为其基类实例的过程。借助向上转型,我们只能调用超类的方法和属性。向上转型后,我们不允许直接调用子类的方法或属性,如果尝试这样做,将会出现错误。
在 Swift 中,向上转型是隐式的,这意味着我们不需要任何特殊的语法来进行向上转型,我们可以直接将子类实例转换为超类实例。直接使用它是安全的,因为子类实例始终被视为超类实例。向上转型通常在我们处理多态代码或希望将不同类型的实例视为其公共基类实例时使用。
示例
Swift 程序,用于将子类实例向上转型为超类实例。
// Base class class Shape { func display(){ print("Ball is in the shape of sphere") } } // Sub class class Rectangle: Shape { func show(){ print("Rectangle is the most commonly used shape") } } // Creating an instance of the Rectangle class let rect = Rectangle() // Upcasting the instance of the rectangle class as the instance of the Shape class let obj : Shape = rect // Accessing the method of superclass obj.display() // Now we are not able to directly access the method or properties of the sub-class // If we do we will get an error // obj.show()
输出
它将产生以下输出:
Ball is in the shape of sphere
Swift 中的向下转型
将超类类型实例转换为子类类型的过程称为向下转型。或者我们可以说,向下转型是一个将基类实例视为派生类实例的过程。向下转型不一定会成功,它也可能失败。Swift 支持两种类型的向下转型:
- 条件向下转型 (as?)
- 强制向下转型 (as!)
让我们详细讨论这两种类型。
条件向下转型 (as?)
条件向下转型 (as?) 运算符用于将超类类型实例向下转换为特定的子类类型。当向下转型成功时,此运算符将返回包含子类实例的可选值。当向下转型不成功时,此运算符将返回 nil。此运算符通常在我们不确定向下转型是否会成功时使用。
语法
以下是条件向下转型运算符 (as?) 的语法:
if let constName = instance as? Type { // Statement that will execute when downcast is successful } else { // Statement that will execute when downcast is unsuccessful }
示例
Swift 程序,演示如何使用条件向下转型运算符 (as?)。
// Base Class class ProgrammingLanguage { func show() { print("Welcome to the great world of learning") } } // Subclass class Swift: ProgrammingLanguage { func display() { print("Welcome to Swift tutorial") } } let obj: ProgrammingLanguage = Swift() // Here the conditional downcasting will be successful if let result1 = obj as? Swift { print("Downcast is successful!") // Accessing subclass method result1.display() } else { print("Downcast is unsuccessful") } // Here the conditional downcasting will be unsuccessful let newObj: ProgrammingLanguage = ProgrammingLanguage() if let result2 = newObj as? Swift { print("\nDowncast is successful!") result2.display() } else { print("Downcast is unsuccessful") }
输出
它将产生以下输出:
Downcast is successful! Welcome to Swift tutorial Downcast is unsuccessful
强制向下转型 (as!)
强制向下转型 (as!) 运算符用于强制将实例向下转换为给定的子类类型。此运算符将返回子类实例。如果向下转型不成功,则会引发运行时错误。此运算符通常在我们确定向下转型一定会成功时使用。
语法
以下是强制向下转型 (as!) 的语法:
let constantName = instance as! type
示例
Swift 程序,演示如何使用强制向下转型运算符 (as!)。
// Base Class class ProgrammingLanguage { func show() { print("Welcome to the great world of learning") } } // Subclass class Swift: ProgrammingLanguage { func display() { print("Welcome to Swift tutorial") } } let obj: ProgrammingLanguage = Swift() // Here the forced downcasting will be successful let res1 = obj as! Swift // Accessing the method of Swift class res1.display() // Here the forced downcasting will be successful so we will get an error /*let newobj: ProgrammingLanguage = ProgrammingLanguage() let res2 = newobj as! Swift res2.display()*/
输出
它将产生以下输出:
Welcome to Swift tutorial
类型检查
在 Swift 中,类型检查用于确定给定实例是否属于指定的子类。我们可以使用 is 运算符执行类型检查。如果实例属于指定的类,则此运算符将返回 true。如果实例不属于指定的类,则此运算符将返回 false。
语法
以下是类型检查的语法:
let constantName = instance as! type
示例
Swift 程序,演示如何检查实例的类型。
// Base Class class ProgrammingLanguage { func show() { print("Welcome to the great world of learning") } } // Subclass class Swift: ProgrammingLanguage { func display() { print("Welcome to Swift tutorial") } } let obj: ProgrammingLanguage = Swift() // Type Checking if obj is Swift{ print("obj is the instance of Swift class") } else { print("No obj is not the instance of Swift class") }
输出
它将产生以下输出:
obj is the instance of Swift class
Any 和 AnyObject 的类型转换
Swift 支持两种用于处理未知类型的值和类实例的特殊类型,它们是
Any - Any 用于表示属于任何类型的实例,包括函数类型。它还包括可选类型。
AnyObject - AnyObject 用于表示任何类类型的实例。
我们可以对 Any 和 AnyObject 执行类型转换和类型检查。
示例
Swift 程序,演示 Any 的类型转换。
// Type Casting for Any var myValue: Any = 22 // Type Checking using is operator if myValue is Int { print("Yes myValue is of Int type!") } else { print("No myValue is not of Int type!") } // Type Casting using as? operator if let res = myValue as? Int { print("Successfully casted to Int: \(res)") } else { print("Could not be able to cast into Int") }
输出
它将产生以下输出:
Yes myValue is of Int type! Successfully casted to Int: 22
示例
Swift 程序,演示 AnyObject 的类型转换。
// Base Class class ProgrammingLanguage { func show() { print("Welcome to the great world of learning") } } // Subclass class Swift: ProgrammingLanguage { func display() { print("Welcome to Swift tutorial") } } // Instance of any object type let obj: AnyObject = Swift() // Type Checking using is operator if obj is Swift { print("Yes it is the instance of Swift") } else { print("No it is not the instance of Swift") } // Type Casting using as? operator if let res = obj as? Swift { print("Successfully casted to Swift") res.display() } else { print("Could not cast to Swift") }
输出
它将产生以下输出:
Yes it is the instance of Swift Successfully casted to Swift Welcome to Swift tutorial