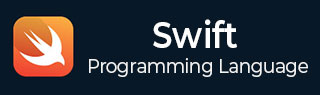
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境配置
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 同一性运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选值(Optionals)
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策语句
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - 穿透语句 (fallthrough)
- Swift 集合
- Swift - 数组
- Swift - 集合 (Sets)
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程 (OOPs)
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 方法重写
- Swift - 初始化
- Swift - 析构
- Swift 高级特性
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法的区别
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 可选值(Optionals)
Swift 引入了一种名为可选类型的新特性。可选类型处理可能不存在或未定义的值。它允许变量表示一个值或 nil。可选值类似于在 Objective-C 中使用 nil 指针,但它们适用于任何类型,而不仅仅是类。要指定可选类型,必须在类型名称后使用问号 (?)。
语法
以下是可选变量的语法:
var perhapsInt: Int?
示例
Swift 程序演示如何创建可选变量。
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 34 // Using optional binding to safely unwrap its value if let x = number { print("Value is \(x)!") } else { print("Value is unknown") }
输出
Value is 34!
带有 nil 的可选值
如果要将可选变量指定为无值状态或没有值,则可以为该变量赋值 **nil**。或者,如果未为可选变量赋值,则 Swift 会自动将该变量的值设置为 nil。在 Swift 中,只允许将 nil 赋值给可选变量,如果尝试将 nil 赋值给非可选变量或常量,则会报错。
语法
以下是将可选值赋值为 nil 的语法:
var perhapsStr: Int? = nil
示例
Swift 程序演示如何将 nil 赋值给可选变量。
// Optional variable var num: Int? = 42 // Setting variable to nil num = nil // Check if the optional has a value or not if num != nil { print("Optional has a value: \(num!)") } else { print("Optional is nil.") }
输出
Optional is nil.
强制展开
如果将变量定义为 **可选** 类型,则要获取此变量的值,必须对其进行展开。因此,要 **展开** 值,必须在变量末尾添加感叹号 (!) 。这告诉编译器您确定可选值包含某个值。
语法
以下是强制展开的语法:
let variableName = optionalValue!
示例
Swift 程序演示如何展开可选值。
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 34 // Using exclamation mark (!) to forcefully unwrapping the optional let value: Int = number! // Displaying the unwrapped value print(value)
输出
34
隐式展开可选值
可以使用感叹号 (!) 而不是问号 (?) 来声明可选变量。此类可选变量将自动展开,无需在变量末尾使用任何其他感叹号 (!) 来获取已赋值的值。
示例
import Cocoa var myString:String! myString = "Hello, Swift 4!" if myString != nil { print(myString) }else{ print("myString has nil value") }
输出
Hello, Swift 4!
可选绑定
使用可选绑定来确定可选值是否包含值,如果包含,则将其值作为临时常量或变量使用。或者可以说,可选绑定允许我们展开和使用可选值中存在的值。它可以防止我们展开 nil 值并导致运行时崩溃。
可以使用 if let、guard 和 while 语句执行可选绑定。允许在一个 if 语句中使用多个可选绑定和布尔条件,用逗号分隔。如果给定的多个可选绑定和布尔条件中的任何一个为 nil,则整个 if 语句都被认为是 false。
语法
以下是可选绑定的语法:
if let constantName = someOptional, let variableName = someOptional { statements }
示例
// Declaring an optional Integer var number: Int? // Assigning a value to the optional number = 50 // Using optional binding to safely unwrap the optional if let x = number { print("Number is \(x)!") } else { print("Number is unknown") }
输出
Number is 50!
空合并运算符 (??)
在 Swift 中,还可以使用空合并运算符 (??) 来处理缺失值,并在可选值为 nil 时提供默认值。它是使用可选绑定和默认值的简写方法。
语法
以下是空合并运算符的语法:
Let output = optionalValue ?? defaultValue
空合并运算符 (??) 检查左侧操作数的值是否不为 nil。如果它不为 nil,则展开该值。如果该值为 nil,则它将提供右侧操作数中的值(或作为备用值)。
示例
// Function to fetch employee salary func getEmpSalary() -> Int? { // Return nil when salary is not available return nil } // Using the nil-coalescing operator to provide a valid salary let salary = getEmpSalary() ?? 5000 // Displaying the result print("Salary: \(salary)")
输出
Salary: 5000