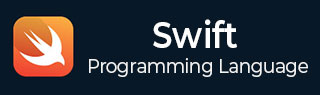
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 同一性运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选值
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - 穿透语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级特性
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 字典
字典用于存储相同类型值的无序列表。Swift 进行了严格的检查,即使是错误的类型,也不会允许您将其添加到字典中。
字典使用一个称为键的唯一标识符来存储值,稍后可以通过相同的键来引用和查找该值。与数组不同,字典中的项目没有指定的顺序。当您需要根据标识符查找值时,可以使用字典。在字典中,键可以是整数或字符串,没有限制,但必须在字典中唯一。而值可以重复。
如果您将创建的字典赋值给一个变量,那么它始终是可变的,这意味着您可以通过添加、删除或更改其项目来更改它。但是,如果您将字典赋值给一个常量,那么该字典是不可变的,其大小和内容都不能更改。
在 Swift 中创建字典
字典包含键值对。键值对的类型可以相同也可以不同,这意味着键和值的类型不必相同。因此,我们可以使用以下语法创建一个特定类型的字典。
语法
以下是创建字典的语法:
var someDict = [KeyType: ValueType](key:value)
我们也可以在不指定类型的情况下创建字典。在这种情况下,编译器将根据赋值的值自动获取字典的类型。以下是创建字典的语法:
var someArray = [key1: value, key2: value, key3: value3]
在 Swift 中过滤字典元素
为了过滤字典元素,Swift 提供了一个名为 filter() 的预定义函数。filter() 函数将闭包作为参数,并返回一个新的字典,该字典只包含闭包中满足给定条件的键值对。
语法
以下是 filter() 函数的语法:
func filter(closure)
示例
import Foundation // Defining and initializing a dictionary var myDict = [3: "Blue", 4: "Pink", 5:"Green", 7:"Pink"] // Filtering out only pink color using filter() function let color = myDict.filter { $0.value == "Pink" } print(color)
输出
它将产生以下输出:
[4: "Pink", 7: "Pink"]
在 Swift 中访问字典
为了访问给定字典的键值对,我们可以使用以下任何一种方法:
使用下标语法
我们可以通过使用下标语法从字典中检索值,在下标语法中,将要检索的值的键放在字典名称后面的方括号内。
语法
以下是访问字典的语法:
var someVar = someDict[key]
示例
import Foundation // Defining a dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Accessing the value of key = 1 using subscript syntax var someVar = someDict[1] // Checking if the value is not nil before using it if let result = someVar { print("Value of key = 1 is \(result)") } else { print("Value not found") }
输出
它将产生以下输出:
Value of key = 1 is One
使用 keys 属性
我们可以使用 keys 属性单独访问键。此属性将返回字典中存在的全部键。
语法
以下是 keys 属性的语法:
dictionary.keys
示例
import Foundation // Defining a dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Accessing the keys var output = someDict.keys print(output)
输出
它将产生以下输出:
[1, 2, 3]
使用 values 属性
我们可以使用 values 属性单独访问值。此属性将返回字典中存在的全部值。
语法
以下是 values 属性的语法:
dictionary.values
示例
import Foundation // Defining a dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Accessing the values var output = someDict.values print(output)
输出
它将产生以下输出:
["Two", "One", "Three"]
在 Swift 中修改字典
为了修改关联键的现有值,Swift 提供了一个名为 updateValue(forKey:) 的预定义方法。如果给定的键值对在给定的字典中不存在,则此方法会将该对添加到字典中。此方法返回替换的值,如果添加新的键值对则返回 nil。
语法
以下是 updateValue() 函数的语法:
func updateValue(value, forKey: key)
示例
import Foundation // Declaring dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] print("Original Dictionary:", someDict) // Updating the value of key = 2 with new value // Using updateValue() function someDict.updateValue("Four", forKey: 2) // Displaying output print("Updated Dictionary:", someDict)
输出
它将产生以下输出:
Original Dictionary: [1: "One", 2: "Two", 3: "Three"] Updated Dictionary: [1: "One", 2: "Four", 3: "Three"]
修改字典中的元素
我们可以使用 [] 在给定键处赋值新值来修改字典的现有元素。方括号 [] 更改给定字典中指定键的值。
语法
以下是更新值的语法:
Dictionary[key] = value
示例
import Foundation // Declaring dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Updating the value of key = 1 with new value someDict[1] = "New value of one" // Displaying the updated value print("Updated Dictionary:", someDict)
输出
它将产生以下输出:
Updated Dictionary: [1: "New value of one", 3: "Three", 2: "Two"]
从字典中移除键值对
为了从字典中移除键值对,Swift 提供了一个名为 removeValue(forKey:) 的预定义方法。此方法移除指定的键及其关联的值(如果存在),并返回移除的值,如果不存在值则返回 nil。
语法
以下是 removeValue() 属性的语法:
Dictionary.removeValue(forKey: Key)
示例
import Foundation // Declaring dictionary var dict = [101: "Blue", 102: "Pink", 103: "Black", 104: "Brown"] print("Original Dictionary:", dict) // Removing a key-Value pair // Using removeValue() method dict.removeValue(forKey: 102) // Displaying output print("Updated Dictionary:", dict)
输出
它将产生以下输出:
Original Dictionary: [102: "Pink", 103: "Black", 104: "Brown", 101: "Blue"] Updated Dictionary: [103: "Black", 104: "Brown", 101: "Blue"]
一次移除所有元素
Swift 提供了另一个名为 removeAll() 的预定义方法。此方法从给定的字典中移除所有键及其关联的值。
示例
import Foundation // Declaring dictionary var dict = [101: "Blue", 102: "Pink", 103: "Black", 104: "Brown"] print("Original Dictionary:", dict) // Removing all the key-Value pairs // Using removeAll() method dict.removeAll() // Displaying output print("Updated Dictionary:", dict)
输出
它将产生以下输出:
Original Dictionary: [101: "Blue", 103: "Black", 104: "Brown", 102: "Pink"] Updated Dictionary: [:]
在 Swift 中迭代字典
为了迭代字典,Swift 提供了 for-in 循环。for-in 循环迭代字典中的所有键值对。
示例
import Foundation // Declaring dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Iterating over the dictionary // Using for-in loop for (key, value) in someDict { print("Dictionary key \(key) - Dictionary value \(value)") }
输出
它将产生以下输出:
Dictionary key 3 - Dictionary value Three Dictionary key 1 - Dictionary value One Dictionary key 2 - Dictionary value Two
使用 enumerated() 函数
我们还可以将 enumerated() 函数与 for-in 循环一起使用,它将返回项目的索引及其 (键,值) 对。
示例
import Foundation // Declaring dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Getting the index of the key-value pairs // Using enumerated() function for (key, value) in someDict.enumerated() { print("Dictionary key \(key) - Dictionary value \(value)") }
输出
它将产生以下输出:
Dictionary key 0 - Dictionary value (key: 1, value: "One") Dictionary key 1 - Dictionary value (key: 2, value: "Two") Dictionary key 2 - Dictionary value (key: 3, value: "Three")
在 Swift 中将字典转换为数组
为了将字典转换为数组,请从给定的字典中提取键值对列表,为键和值分别构建数组。
示例
import Foundation // Declaring dictionary var someDict:[Int:String] = [1:"One", 2:"Two", 3:"Three"] // Declaring two arrays and storing keys and values separately let dictKeys = [Int](someDict.keys) let dictValues = [String](someDict.values) // Displaying the arrays print("Print Dictionary Keys") for (key) in dictKeys { print("\(key)") } print("Print Dictionary Values") for (value) in dictValues { print("\(value)") }
输出
它将产生以下输出:
Print Dictionary Keys 1 3 2 Print Dictionary Values One Three Two
字典的“count”属性
count 属性用于计算字典中存在的元素总数。
语法
以下是 count 属性的语法:
Dictionary.count
示例
import Foundation // Declaring dictionaries var someDict1 : [Int:String] = [1:"One", 2:"Two", 3:"Three"] var someDict2 : [Int:String] = [4:"Four", 5:"Five"] // Counting the elements of dictionaries // Using count property var size1 = someDict1.count var size2 = someDict2.count print("Total items in someDict1 = \(size1)") print("Total items in someDict2 = \(size2)")
输出
它将产生以下输出:
Total items in someDict1 = 3 Total items in someDict2 = 2
字典的“empty”属性
empty 属性用于检查给定的字典是否为空。如果给定的字典为空,则此属性将返回 true。如果给定的字典包含一些元素,则此属性将返回 false。
语法
以下是 empty 属性的语法:
Dictionary.isEmpty
示例
import Foundation // Declaring dictionaries var someDict1:[Int:String] = [1:"One", 2:"Two", 3:"Three"] var someDict2:[Int:String] = [4:"Four", 5:"Five"] var someDict3:[Int:String] = [Int:String]() // Checking if the dictionary is empty or not // Using isEmpty property print("someDict1 = \(someDict1.isEmpty)") print("someDict2 = \(someDict2.isEmpty)") print("someDict3 = \(someDict3.isEmpty)")
输出
它将产生以下输出:
someDict1 = false someDict2 = false someDict3 = true