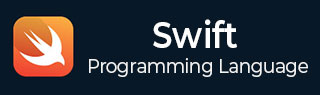
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - 穿透语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 穿透语句
Swift 中的 switch 语句在第一个匹配的 case 完成执行后就立即结束,而不是像 C 和 C++ 编程语言那样贯穿到后续 case 的底部。
C 和 C++ 中 switch 语句的通用语法如下所示:
switch(expression){ case constant-expression : statement(s); break; /* optional */ case constant-expression : statement(s); break; /* optional */ /* you can have any number of case statements */ default : /* Optional */ statement(s); }
这里我们需要使用 break 语句退出 case 语句,否则执行控制将贯穿到匹配的 case 语句下方可用的后续 case 语句。
因此,在 Swift 中,我们可以使用 fallthrough 语句实现相同的功能。此语句允许控制转移到下一个 case 语句,而不管当前 case 的条件是否匹配。由于此原因,开发人员不太喜欢它,因为它使代码可读性降低或可能导致意外行为。
语法
以下是 fallthrough 语句的语法:
switch expression { case expression1 : statement(s) fallthrough /* optional */ case expression2, expression3 : statement(s) fallthrough /* optional */ default : /* Optional */ statement(s); }
如果我们不使用 fallthrough 语句,则程序将在执行匹配的 case 语句后退出 switch 语句。我们将通过以下两个示例来说明其功能。
示例
Swift 程序演示了如何使用不带 fallthrough 的 switch 语句。
import Foundation var index = 10 switch index { case 100 : print( "Value of index is 100") case 10,15 : print( "Value of index is either 10 or 15") case 5 : print( "Value of index is 5") default : print( "default case") }
输出
它将产生以下输出:
Value of index is either 10 or 15
示例
Swift 程序演示了如何使用带 fallthrough 的 switch 语句。
import Foundation var index = 10 switch index { case 100 : print( "Value of index is 100") fallthrough case 10,15 : print( "Value of index is either 10 or 15") fallthrough case 5 : print( "Value of index is 5") default : print( "default case") }
输出
它将产生以下输出:
Value of index is either 10 or 15 Value of index is 5
广告