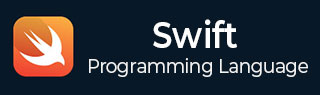
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选值
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fallthrough 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级特性
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - switch 语句
switch 语句是最强大的控制语句,它允许我们以易于阅读的方式表示多分支逻辑。它提供了一种方法来根据多个给定的 case 评估值,并执行第一个匹配 case 中的语句块。
switch 语句是 if-else 语句的替代方案,因为它比 if-else 语句更有效地处理多个条件。
语法
以下是 switch 语句的语法:
switch expression{ case 1: // Statement fallthrough // Optional case 2: // Statement break // Optional default: // Statement
示例
以下是 switch 语句的示例:
import Foundation var index = 10 switch index { case 100 : print( "Value of index is 100") case 10,15 : print( "Value of index is either 10 or 15") case 5 : print( "Value of index is 5") default : print( "default case") }
输出
它将产生以下输出:
Value of index is either 10 or 15
Swift switch 语句中的 break 语句
break 语句用于在执行匹配的 case 语句块后立即终止 switch 块,并将控制转移到 switch 块之后出现的语句。break 语句用于 case 块中。
语法
以下是 break 语句的语法:
switch expression{ case x: // Statement break default: // Statement }
示例
以下是 break 语句的示例:
import Foundation let day = 4 switch day { // Case with the break statements case 1: print("Monday") break case 2: print("Tuesday") break case 3: print("Wednesday") break case 4: print("Thursday") break case 5: print("Friday") break default: print("Weekend") }
输出
它将产生以下输出:
Thursday
Swift switch 语句中的 fallthrough
switch 语句一旦遇到第一个匹配的 case 就会结束执行,即使我们使用或不使用 break 语句也是如此。与 C 和 C++ 编程不同,控制不会贯穿后续 case 的底部。
如果我们想在 Swift 中实现 fallthrough 功能,则必须在每个 case 中显式使用 fallthrough 语句。fallthrough 语句允许控制传递到下一个 case,即使该 case 的条件不匹配。
语法
以下是 fallthrough 语句的语法:
switch expression{ case x: // Statement fallthrough default: // Statement }
示例
以下是 fallthrough 语句的示例:
import Foundation let number = 2 switch number { // Case statement with fall through case 1: print("Hello i am case 1") fallthrough case 2: print("Hello i am case 2") fallthrough case 3: print("Hello i am case 3") default: print("Not found") }
输出
它将产生以下输出:
Hello i am case 2 Hello i am case 3
Swift switch 语句中的范围
在 Swift 中,我们允许在 case 语句中使用范围来根据一系列元素匹配值。当我们想要处理值落在给定范围内的 case 时,通常会使用它。
语法
以下是 case 语句中范围的语法:
switch expression{ case x…y: // Statement default: // Statement }
示例
以下是 case 语句中范围的示例:
import Foundation let temp = 27 switch temp { // Case statement with ranges case ..<0: print("Freezing.") case 0...20: print("Cold.") case 21...30: print("Moderate.") case 31...: print("Hot") default: print("Please enter valid temperature") }
输出
它将产生以下输出:
Moderate.
Swift switch 语句中的元组匹配
众所周知,元组用于将多个值存储在一起,无论它们是否为同一类型。因此,我们允许在 switch 语句中使用元组作为变量表达式,也可以使用元组在 case 语句中测试多个值。我们也可以在元组中使用通配符模式“_”。
语法
以下是元组匹配的语法:
switch (x1, x2){ case (y, x1): // Statement default: // Statement }
示例
以下是元组匹配的示例:
import Foundation // Switch expression in tuple let number = (num1: 25, num2: 10, num3: 11) switch number { // Case expression in tuple case (25, 10, 0): print("Numbers are (25, 10, 0)") case (13, _, 11): print("Numbers are (13, 11)") case (25, 10, 11): print("Number are (25, 10, 11)") default: print("Number is not found") }
输出
它将产生以下输出:
Number are (25, 10, 11)
Swift switch 语句中的值绑定
Swift 提供了一个名为值绑定的新属性。使用此属性,我们允许在 case 语句中初始化一个临时变量,并且此变量仅在其定义的 case 的主体中可用。或者我们可以说它将匹配值绑定到相关块中的临时变量或常量。
语法
以下是值绑定的语法:
switch expression{ case (let x, let y): // Statement default: // Statement }
示例
以下是值绑定的示例:
import Foundation let num = 17 switch num { // Value binding case let y where y % 2 == 0: print("\(num) is an even number.") case let z where z % 2 != 0: print("\(num) is an odd number.") default: print("\(num) is invalid number.") }
输出
它将产生以下输出:
17 is an odd number.
Swift 带 where 子句的 switch 语句
我们还可以将 where 子句与 case 条件一起使用以检查额外条件。当我们想根据附加条件筛选 case 时,可以使用它。
语法
以下是带有 where 子句的 case 的语法:
switch expression{ case "0"…"8" where expression.isNumber: // Statement default: // Statement }
示例
以下是带有 where 子句的 case 的示例:
import Foundation let marks = 77 switch marks { case 0...59: print("Sorry! You are Failed") // Case with where clause case 60...79 where marks < 75: print("Congratulations! You are Passed") case 60...79 where marks >= 75: print("Congratulations! You are Passed with distinction") case 80...100: print("Excellent! You topped the Class") default: print("Not a valid score") }
输出
它将产生以下输出:
Congratulations! You are Passed with distinction
Swift 中的复合 case
在 switch 语句中,我们允许在一个 case 中使用多个模式,其中 case 中的所有模式都用逗号分隔,并共享相同的代码块。
如果任何给定的模式匹配,则将执行该 case 中的代码块。当我们想要为各种 case 执行相同的代码块时,通常会使用复合 case。
语法
以下是复合 case 的语法:
switch expression{ case a, b, c: // Statement case x, y, z, w: // Statement default: // Statement }
示例
以下是复合 case 的示例:
import Foundation let number = 20 switch number { // Compound case case 10, 20, 30: print("Selected number is 10, 20 or 30") case 40, 50, 60: print("Selected number is 40, 50, 60") default: print("Value not found") }
输出
它将产生以下输出:
Selected number is 10, 20 or 30