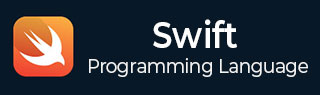
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift逃逸闭包和非逃逸闭包
- Swift - 自动闭包
- Swift 面向对象
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift逃逸闭包和非逃逸闭包
Swift 闭包
就像其他编程语言一样,Swift 也支持闭包。闭包是自包含的功能块,可以传递和使用在代码中执行任何特定任务。它可以分配给变量或作为参数传递给函数。闭包可以捕获其周围上下文中的值,并且可以用作回调或内联代码。Swift 支持以下类型的闭包:
- 逃逸闭包
- 非逃逸闭包
让我们详细讨论它们。
Swift 中的逃逸闭包
当闭包作为参数传递给函数,但该闭包在函数返回后被调用时,则这种类型的闭包称为逃逸闭包。默认情况下,作为参数传递给函数的闭包是非逃逸参数,这意味着闭包将在函数执行期间执行。
要将闭包声明为逃逸闭包,我们必须在表示逃逸闭包的参数类型之前使用@escaping关键字。
语法
以下是逃逸闭包的语法:
func methodname(closure: @escaping() -> Void){ // body // Calling the closure closure() }
示例
Swift 程序演示逃逸闭包。
import Foundation class Operation{ // Method that takes two numbers and an escaping closure to find their product func product(_ x: Int, _ y: Int, productResult: @escaping (Int) -> Void) { // Activating an asynchronous task DispatchQueue.global().async { let output = x * y // Calling escaping closure productResult(output) } } } // Creating object of Operation class let obj = Operation() // Accessing the method obj.product(10, 4) { res in print("Product of 10 * 4 = \(res)") } // Activating the passage of time DispatchQueue.main.asyncAfter(deadline: .now() + 2) { // It will be executed after a delay, simulating an asynchronous operation }
输出
它将产生以下输出:
Product of 10 * 4 = 40
Swift 中的非逃逸闭包
非逃逸闭包是 Swift 中的默认设置,我们不需要任何特殊符号来表示非逃逸闭包。这种类型的闭包在其传递到的函数的执行期间执行,一旦函数的执行结束,闭包将不再可用。它们不会被存储以供以后执行。它们不会被存储供以后执行。
语法
以下是非逃逸闭包的语法:
mutating func methodname(Parameters) -> returntype { Statement }
示例
Swift 程序演示非逃逸闭包。
class Operation { // Function that takes two numbers and a non-escaping closure to calculate their sum func sum(_ X: Int, _ Y: Int, add: (Int) -> Void) { let result = X + Y // Calling the non-escaping closure add(result) } } // Creating the object of operation class let obj = Operation() obj.sum(10, 12) { add in print("sum: \(add)") }
输出
它将产生以下输出:
Sum: 22
逃逸闭包与非逃逸闭包
以下是逃逸闭包和非逃逸闭包的主要区别:
逃逸闭包 | 非逃逸闭包 |
---|---|
它可以超出其传递到的函数的生命周期。 | 它在函数执行期间执行。 |
它可以存储为属性或分配给变量。 | 它不能存储,也不允许在函数作用域之外使用。 |
它通常用于异步操作,如网络请求等。 | 它通常用于同步操作,如简单的计算等。 |
@escaping 关键字用于创建逃逸闭包。 | 它是默认的闭包,不需要任何特殊的语法。 |
广告