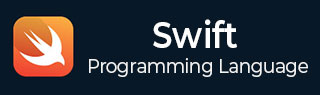
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 嵌套 if 语句
在 Swift 中,允许将一个 if 语句嵌套在另一个 if 语句中。因此,当外部 if 语句的条件为真时,控制权才能访问嵌套的 if 语句。否则,控制权将跳过嵌套的 if 语句,并执行外部 if 语句之后存在的代码块。
您还可以将 if 语句嵌套在 if-else 语句中,反之亦然。您可以根据需要嵌套任意数量的 if 语句,但尽量避免过度嵌套,因为如果遇到错误,过度嵌套的代码难以维护。
语法
以下是嵌套 if 语句的语法:
if boolean_expression_1{ /* statement(s) will execute if the boolean expression 1 is true */ If boolean_expression_2{ /* statement(s) will execute if the boolean expression 2 is true */ } }
您可以像嵌套 if 语句一样,以类似的方式嵌套else if...else。
流程图
以下流程图将显示嵌套 if 语句的工作原理。
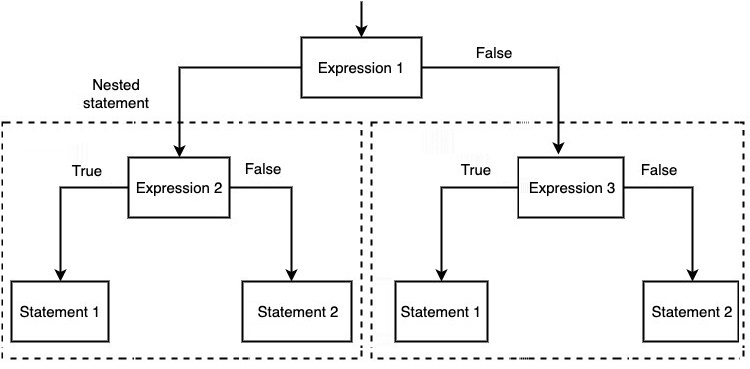
示例
Swift 程序演示嵌套 if 语句的使用。
import Foundation var varA:Int = 100; var varB:Int = 200; /* Check the boolean condition using if statement */ if varA == 100 { /* If the condition is true then print the following */ print("First condition is satisfied") if varB == 200 { /* If the condition is true then print the following */ print("Second condition is also satisfied") } } print("Value of variable varA is \(varA)") print("Value of variable varB is \(varB)")
输出
它将产生以下输出:
First condition is satisfied Second condition is also satisfied Value of variable varA is 100 Value of variable varB is 200
示例
Swift 程序使用嵌套 if-else 语句查找闰年。
import Foundation let myYear = 2027 // Checking leap year if myYear % 4 == 0 { if myYear % 100 == 0 { if myYear % 400 == 0 { print("\(myYear) is a leap year.") } else { print("\(myYear) is not a leap year.") } } else { print("\(myYear) is a leap year.") } } else { print("\(myYear) is not a leap year.") }
输出
它将产生以下输出:
2027 is not a leap year.
示例
Swift 程序检查给定数字是正数还是负数,是偶数还是奇数。
import Foundation let num = -11 // Checking if the given number is positive // or negative even or odd number if num > 0 { if num % 2 == 0 { print("Positive even number.") } else { print("Positive odd number.") } } else if num < 0 { if num % 2 == 0 { print("Negative even number.") } else { print("Negative odd number.") } } else { print("Number is zero.") }
输出
它将产生以下输出:
Negative odd number.
广告