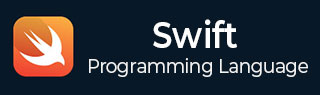
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策制定
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift - fall through 语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 枚举
枚举是一种用户定义的数据类型,它由一组相关的值组成,并提供了一种以类型安全的方式处理这些值的方法。枚举通常不为每个情况提供值,但如果需要,可以为每个枚举情况分配值,并且该值可以是任何类型,例如字符串、整数、浮点数或字符。
Swift 中的枚举
我们可以使用enum关键字后跟名称和花括号来定义枚举,其中花括号包含使用case关键字的枚举情况。枚举名称应以大写字母开头(例如:enum DaysofaWeek)。
语法
以下是枚举的语法:
enum EnumName { // enumeration cases case value1 case value2 ... case valueN }
我们还可以将多个枚举情况定义在一行中,其中每个情况用逗号分隔。
enum EnumName { // enumeration cases case value1, value2, value3,…,valueN }
示例
以下是 Swift 的示例,演示如何创建枚举:
enum CarColor { // enum values case Blue case Green case White case Off-white }
在 Swift 中创建枚举变量
在枚举中,我们可以直接创建一个枚举类型变量并为其分配一个情况。我们可以使用点(.)表示法将情况分配给枚举变量,其中点表示法后跟值(例如:.value)。
语法
以下是创建枚举变量的语法:
var variableName : EnumName
以下是为枚举变量赋值的语法:
variableName = .enumValue
示例
Swift 程序用于创建和访问枚举变量。
// Defining an enumeration with cases representing subjects enum Subjects { case Maths case Science case Social case English case Hindi case ComputerProgramming } // Creating and assigning value to an enum variable var name: Subjects = .English // Access the value of the enum variable and display data accordingly switch name { case .English, .Hindi, .ComputerProgramming: print("Elective subjects!") case .Maths, .Science, .Social: print("Compulsory subjects") }
输出
它将产生以下输出:
Elective subjects!
示例
Swift 程序用于创建和访问枚举变量。
// Defining an enumeration with cases representing car colour enum Subjects { case Black case Blue case MidnightGray case White case OffWhite case Silver } // Creating and assigning value to an enum variable var color: Subjects = .Blue // Using an if statement to check the enum value if color == .Blue { print("Dark edition of car") }
输出
它将产生以下输出:
Dark edition of car
Swift 中带有原始值的枚举
在枚举中,我们还可以为枚举情况分配值,这些值称为原始值。原始值可以是字符串、字符或任何整数或浮点数类型。每个原始值在其枚举声明中必须是唯一的,并且类型相同。
语法
以下是为枚举情况分配原始值的语法:
enum enumName : Type{ // enum values case value1 = RawValue1 case value2 = RawValue2 }
访问原始值
我们可以借助名为rawValue的预定义属性来访问原始值。rawValue属性用于检索与指定枚举情况关联的原始值。
语法
以下是rawValue属性的语法:
let variableName = enumName.enumCase.rawValue
示例
Swift 程序用于使用rawValue属性访问枚举的原始值。
// Defining an enumeration with cases representing car colour enum CarColor : Int { case Black = 2 case Blue = 4 case OffWhite = 5 case Silver = 6 } // Accessing the raw value var colorCount = CarColor.Blue.rawValue // Displaying the raw values print("Raw Value:", colorCount)
输出
它将产生以下输出:
Raw Value: 4
隐式分配原始值
当整数或字符串用作枚举情况的原始值时,则无需为每个枚举情况指定值,因为 Swift 将自动为每个枚举情况分配值。此类方法称为隐式分配原始值。
当我们使用 Integer 类型作为枚举情况的原始值并为第一个情况提供原始值时,Swift 将自动为后续情况分配原始值,其中分配给当前情况的值大于前一个情况的值。
示例
Swift 程序用于隐式分配 Integer 类型的原始值。
// Defining an enumeration with cases representing marks subject enum SubjectMarks: Int { // Assigning raw value to the first case // Now Swift will automatically assign raw values to the cases // for english = 41, hindi = 42, and physics = 43 case maths = 40, english, hindi, physics } // Accessing the raw value let marks = SubjectMarks.hindi.rawValue print("Marks of hindi = ", marks)
输出
它将产生以下输出:
Marks of hindi = 42
如果我们不为第一个情况分配原始值,则默认情况下,Swift 将为第一个情况分配零,然后为第二个情况分配 1,为第三个情况分配 2,依此类推。
示例
// Defining an enumeration with cases representing marks subject enum SubjectMarks: Int { // Here we do not assign raw value to the first case // So Swift will automatically assign default raw values case maths // Default raw value = 0 case english // Default raw value = 1 case hindi // Default raw value = 2 case physics // Default raw value = 3 } // Accessing the raw value let marks = SubjectMarks.hindi.rawValue print("Marks of hindi = ", marks)
输出
它将产生以下输出:
Marks of hindi = 2
当我们使用 String 类型作为枚举情况的原始值并且不为情况提供原始值时。然后,默认情况下,Swift 将隐式分配情况的名称作为原始值。
示例
Swift 程序用于隐式分配 String 类型的原始值。
// Defining an enumeration with cases representing subject names enum Subjects: String{ // Here we do not assign raw value to the cases // So Swift will automatically assign default raw values case maths // Default raw value = maths case english // Default raw value = english case hindi // Default raw value = hindi case physics // Default raw value = physics } // Accessing the raw value let marks = Subjects.hindi.rawValue print("Marks of hindi = ", marks)
输出
它将产生以下输出:
Marks of hindi = hindi
从原始值初始化
在定义带有原始值类型的枚举时,Swift 会自动为该枚举创建一个初始化器,该初始化器将原始值作为参数并返回一个可选值(一个情况或 nil)。如果原始值与任何给定情况匹配,则初始化器将返回包含在可选值中的该情况,而如果原始值与任何给定情况都不匹配,则它将返回 nil。
示例
Swift 程序用于从原始值初始化。
// Defining an enumeration with raw values of string type enum Fruits: String { case value1 = "Mango" case value2 = "Apple" case value3 = "Banana" case value4 = "Orange" } // Initializing from raw values if let result = Fruits(rawValue: "Apple") { print("Case '\(result)' is found for the given raw value") } else { print("No is case found") }
输出
它将产生以下输出:
Case 'value2' is found for the given raw value
Swift 中带有关联值的枚举
带有关联值的枚举是 Swift 提供的最重要的功能。它允许我们在枚举的每个情况中添加一些其他信息。它通常用于类型可以具有不同变体和不同关联数据的情况。
示例
Swift 程序演示带有关联值的枚举。
// Defining an enumeration with associate values enum Student{ case Name(String) case Mark(Int, Int, Int) } // Creating instances of the enum var studDetails = Student.Name("Swift") var studMarks = Student.Mark(98, 97, 95) // Accessing the associate values switch studMarks { case .Name(let studName): print("Student name is: \(studName).") case .Mark(let Mark1, let Mark2, let Mark3): print("Student Marks are: \(Mark1), \(Mark2), \(Mark3).") }
输出
它将产生以下输出:
Student Marks are: 98, 97, 95.
关联值和原始值之间的区别
关联值 | 原始值 |
---|---|
不同数据类型 | 相同数据类型 |
例如:enum {10,0.8,"Hello"} | 例如:enum {10,35,50} |
基于常量或变量创建的值 | 预填充值 |
每次声明时都不同 | 成员的值相同 |
Swift 中使用 Switch 语句的枚举
正如我们所知,Switch 语句也遵循多路选择,这意味着根据指定的条件,一次只能访问一个变量。因此,我们可以使用 Switch 语句来处理枚举的不同情况。
示例
Swift 程序演示使用 Switch 语句的枚举。
// Defining an enumeration with cases enum Climate { case India case America case Africa case Australia } // Accessing enumeration cases using a switch statement var season = Climate.America season = .America switch season { case .India: print("Climate is Hot") case .America: print("Climate is Cold") case .Africa: print("Climate is Moderate") case .Australia: print("Climate is Rainy") }
输出
它将产生以下输出:
Climate is Cold
在 Swift 中迭代枚举
要迭代枚举,我们必须使用“CaseIterable”协议。此协议提供给定枚举的所有情况的集合。然后,我们将在循环中使用allCases属性来迭代每个情况,其中迭代的顺序取决于定义情况的顺序。如果需要按特定顺序排列情况,则相应地定义它们。
示例
Swift 程序用于迭代给定的枚举。
// Defining enumeration with cases enum Veggies: CaseIterable { case Onion case Carrot case Beetroot case Tomato case GreenChilly } // Iterating over the given enumeration for x in Veggies.allCases { print(x) }
输出
它将产生以下输出:
Onion Carrot Beetroot Tomato GreenChilly
Swift 中的递归枚举
在 Swift 中,我们允许创建递归枚举。递归枚举是一种特殊的枚举,其中情况可以具有枚举类型本身的关联值。我们必须在递归枚举情况之前使用indirect关键字,它告诉编译器添加所有必要的间接层。此外,并非给定枚举中存在的所有情况都需要标记为间接,只有那些涉及递归的情况才应标记为间接。
示例
Swift 程序演示递归枚举。
// Recursive enumeration for representing arithmetic expressions enum Calculator { case number(Int) // Cases that involve recursion indirect case sum(Calculator, Calculator) indirect case product(Calculator, Calculator) } // Expressions let num1 = Calculator.number(10) let num2 = Calculator.number(12) let addition = Calculator.sum(num1, num2) let prod = Calculator.product(num1, addition) // Function to evaluate an expression func result(_ exp: Calculator) -> Int { switch exp { case let .number(value): return value case let .sum(left, right): return result(left) + result(right) case let .product(left, right): return result(left) * result(right) } } // Displaying result let output = result(addition) print("Sum is \(output)")
输出
它将产生以下输出:
Sum is 22