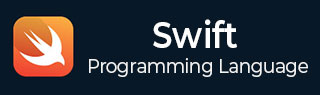
- Swift 教程
- Swift - 首页
- Swift - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 变量
- Swift - 常量
- Swift - 字面量
- Swift - 注释
- Swift 运算符
- Swift - 运算符
- Swift - 算术运算符
- Swift - 比较运算符
- Swift - 逻辑运算符
- Swift - 赋值运算符
- Swift - 位运算符
- Swift - 其他运算符
- Swift 高级运算符
- Swift - 运算符重载
- Swift - 算术溢出运算符
- Swift - 恒等运算符
- Swift - 范围运算符
- Swift 数据类型
- Swift - 数据类型
- Swift - 整数
- Swift - 浮点数
- Swift - Double
- Swift - 布尔值
- Swift - 字符串
- Swift - 字符
- Swift - 类型别名
- Swift - 可选类型
- Swift - 元组
- Swift - 断言和前提条件
- Swift 控制流
- Swift - 决策
- Swift - if 语句
- Swift - if...else if...else 语句
- Swift - if-else 语句
- Swift - 嵌套 if 语句
- Swift - switch 语句
- Swift - 循环
- Swift - for in 循环
- Swift - while 循环
- Swift - repeat...while 循环
- Swift - continue 语句
- Swift - break 语句
- Swift -贯穿语句
- Swift 集合
- Swift - 数组
- Swift - 集合
- Swift - 字典
- Swift 函数
- Swift - 函数
- Swift - 嵌套函数
- Swift - 函数重载
- Swift - 递归
- Swift - 高阶函数
- Swift 闭包
- Swift - 闭包
- Swift - 转义和非转义闭包
- Swift - 自动闭包
- Swift 面向对象编程
- Swift - 枚举
- Swift - 结构体
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 重写
- Swift - 初始化
- Swift - 析构
- Swift 高级特性
- Swift - ARC 概述
- Swift - 可选链
- Swift - 错误处理
- Swift - 并发
- Swift - 类型转换
- Swift - 嵌套类型
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- Swift - 函数与方法
- Swift - SwiftyJSON
- Swift - 单例类
- Swift 随机数
- Swift 不透明类型和装箱类型
- Swift 有用资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用资源
- Swift - 讨论
Swift - 嵌套函数
函数是一组语句块,用于执行特定任务。当一个函数定义在另一个函数内部时,这种类型的函数被称为嵌套函数。嵌套函数的作用域仅限于其定义所在的函数,这意味着它们只能在其外部函数内部访问。如果尝试在外部函数之外访问它们,则会报错。
在 Swift 中,单个函数可以包含多个嵌套函数,并且必须在调用它们之前定义它们。
嵌套函数提高了可读性,并通过将复杂任务分解成更小、可重用的组件来组织这些任务。
嵌套函数将逻辑封装在有限的作用域内,并可以防止外部访问。
它们只能访问其外部函数的变量。
嵌套函数的语法
以下是嵌套函数的语法:
func outerFunction(){ func nestedFunction(){ // code } func nestedFunction2(){ //code } nestedFunction1() nestedFunction2() }
Swift 中无参数的嵌套函数
在 Swift 中,可以定义没有任何参数的嵌套函数。这种类型的函数在调用时不接受任何参数,并且可能返回或不返回值。
语法
以下是无参数列表的嵌套函数的语法:
func nestedFunction(){ // Code }
示例
使用嵌套函数显示世界杯冠军名称的 Swift 程序。
import Foundation // Outer function func worldCupTrophy(){ // Nested function without parameter list func winnerName() { print("Winner of World Cup is INDIA") } // Calling nested function winnerName() } // Calling the outer function to display the result worldCupTrophy()
输出
它将产生以下输出:
Winner of World Cup is INDIA
Swift 中带有参数的嵌套函数
嵌套函数可以包含参数列表而没有返回类型。这种类型的嵌套函数只能访问外部函数的变量和参数。嵌套函数可以包含多个参数,它们由参数标签分隔。
语法
以下是带有参数的嵌套函数的语法:
func nestedFunction(name1: Int, name2: Int){ // code }
示例
使用嵌套函数添加两个字符串的 Swift 程序。
import Foundation // Outer function func concatenateStrings(str1: String, str2: String){ // Nested function with parameter list func displayResult(finalStr: String) { print("Concatenated String: \(finalStr)") } let result = str1 + str2 // Calling nested function displayResult(finalStr: result) } // Input strings var string1 = "Welcome " var string2 = "TutorialsPoint" // Calling the outer function to display the result concatenateStrings(str1: string1, str2: string2)
输出
它将产生以下输出:
Concatenated String: Welcome TutorialsPoint
Swift 中无返回类型的嵌套函数
无返回类型的嵌套函数不返回值。它可能包含或不包含参数。
语法
以下是无返回类型的嵌套函数的语法:
func nestedFunction(name: Int){ // code return }
示例
使用嵌套函数计算矩形面积的 Swift 程序。
import Foundation // Outer function func Rectangle(length: Int, width: Int){ // Nested function without return type func displayArea(finalArea: Int) { print("Area of Rectangle: \(finalArea)") } let result = length * width // Calling nested function displayArea(finalArea: result) } // Input strings var l = 10 var w = 12 // Calling the outer function to display the result Rectangle(length: l, width: w)
输出
它将产生以下输出:
Area of Rectangle: 120
Swift 中有返回类型的嵌套函数
嵌套函数可以包含参数列表而没有返回类型。有返回类型的嵌套函数总是返回某些值。
语法
以下是带有返回类型的嵌套函数的语法:
func nestedFunction(name1: Int, name2: Int) -> ReturnType{ // code return }
示例
使用嵌套函数计算两个图形(矩形和正方形)的总面积的 Swift 程序。
import Foundation // Outer function without return type func Areas(length: Int, width: Int){ // Nested function with return type func sumOfAreas(area1: Int, area2: Int)-> Int { let Sum = area1 + area2 return Sum } let areaOfRectangle = length * width let areaOfSquare = length * length // Calling nested function print("Total Area: \(sumOfAreas(area1: areaOfRectangle, area2: areaOfSquare))") } // Calling the outer function to display the result Areas(length: 23, width: 25)
输出
它将产生以下输出:
Total Area: 1104
广告