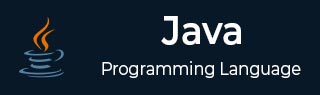
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java vs. C++
- JVM - Java虚拟机
- Java - JDK vs JRE vs JVM
- Java - Hello World程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java控制语句
- Java - 循环控制
- Java - 决策机制
- Java - if-else语句
- Java - switch语句
- Java - for循环
- Java - for-each循环
- Java - while循环
- Java - do-while循环
- Java - break语句
- Java - continue语句
面向对象编程
- Java - 面向对象编程概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造器
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造器
- Java - 枚举字符串
Java内置类
Java文件处理
Java错误和异常
- Java - 异常
- Java - try-catch块
- Java - try-with-resources语句
- Java - 多重catch块
- Java - 嵌套try块
- Java - finally块
- Java - throw异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程合并
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java同步
Java网络编程
- Java - 网络编程
- Java - Socket编程
- Java - URL处理
- Java - URL类
- Java - URLConnection类
- Java - HttpURLConnection类
- Java - Socket类
- Java -泛型
Java集合
Java接口
Java数据结构
Java集合算法
高级Java
- Java - 命令行参数
- Java - Lambda表达式
- Java - 发送邮件
- Java - Applet基础
- Java - Javadoc注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本Jar文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64编码解码
- Java - switch表达式
- Java - Teeing收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态CDS归档
- Java - Z垃圾收集器(ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾回收
- Java - JIT编译器
Java杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强的@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs和框架
Java类引用
- Java - Scanner类
- Java - 数组
- Java - 字符串
- Java - Date类
- Java - ArrayList类
- Java - Vector类
- Java - Stack类
- Java - PriorityQueue类
- Java - LinkedList类
- Java - ArrayDeque类
- Java - HashMap类
- Java - LinkedHashMap类
- Java - WeakHashMap类
- Java - EnumMap类
- Java - TreeMap类
- Java - IdentityHashMap类
- Java - HashSet类
- Java - EnumSet类
- Java - LinkedHashSet类
- Java - TreeSet类
- Java - BitSet类
- Java - Dictionary类
- Java - Hashtable类
- Java - Properties类
- Java - Collection类
- Java - Array类
Java有用资源
Java - extends关键字
Java 的extends关键字用于继承类的属性。以下是extends关键字的语法。
语法
class Super { ..... ..... } class Sub extends Super { ..... ..... }
示例代码
以下是一个演示Java继承的示例。在这个例子中,您可以看到两个类,名为Calculation和My_Calculation。
使用extends关键字,My_Calculation继承了Calculation类的addition()和Subtraction()方法。
复制并粘贴以下程序到名为My_Calculation.java的文件中
示例
class Calculation { int z; public void addition(int x, int y) { z = x + y; System.out.println("The sum of the given numbers:"+z); } public void Subtraction(int x, int y) { z = x - y; System.out.println("The difference between the given numbers:"+z); } } public class My_Calculation extends Calculation { public void multiplication(int x, int y) { z = x * y; System.out.println("The product of the given numbers:"+z); } public static void main(String args[]) { int a = 20, b = 10; My_Calculation demo = new My_Calculation(); demo.addition(a, b); demo.Subtraction(a, b); demo.multiplication(a, b); } }
输出
编译并执行上述代码,如下所示。
javac My_Calculation.java java My_Calculation
程序执行后,将产生以下结果:
The sum of the given numbers:30 The difference between the given numbers:10 The product of the given numbers:200
在给定的程序中,当创建My_Calculation类的对象时,超类的内容副本将被创建在其内。这就是为什么,使用子类的对象,您可以访问超类的成员。
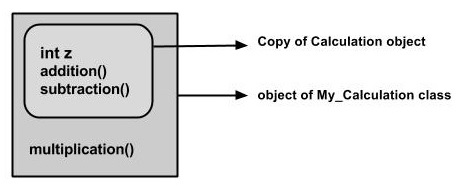
超类引用变量可以持有子类对象,但是使用该变量只能访问超类的成员,因此为了访问两个类的成员,建议始终为子类创建引用变量。
如果您考虑上述程序,您可以如下所示实例化类。但是使用超类引用变量(本例中为cal)您无法调用属于子类My_Calculation的方法multiplication()。
Calculation demo = new My_Calculation(); demo.addition(a, b); demo.Subtraction(a, b);
以下示例展示了相同的概念。
示例
class Calculation { int z; public void addition(int x, int y) { z = x + y; System.out.println("The sum of the given numbers:"+z); } public void Subtraction(int x, int y) { z = x - y; System.out.println("The difference between the given numbers:"+z); } } public class My_Calculation extends Calculation { public void multiplication(int x, int y) { z = x * y; System.out.println("The product of the given numbers:"+z); } public static void main(String args[]) { int a = 20, b = 10; Calculation demo = new My_Calculation(); demo.addition(a, b); demo.Subtraction(a, b); } }
输出
编译并执行上述代码,如下所示。
javac My_Calculation.java java My_Calculation
程序执行后,将产生以下结果:
The sum of the given numbers:30 The difference between the given numbers:10
注意 - 子类继承其超类的所有成员(字段、方法和嵌套类)。构造器不是成员,因此不会被子类继承,但是可以从子类调用超类的构造器。
java_basic_syntax.htm
广告