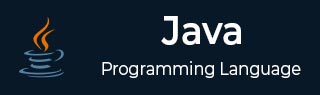
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java vs. C++
- JVM - Java虚拟机
- Java - JDK vs JRE vs JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for循环
- Java - for-each循环
- Java - while循环
- Java - do-while循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch块
- Java - try-with-resources
- Java - 多重catch块
- Java - 嵌套try块
- Java - finally块
- Java - throw异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - Socket编程
- Java - URL处理
- Java - URL类
- Java - URLConnection类
- Java - HttpURLConnection类
- Java - Socket类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级Java
- Java - 命令行参数
- Java - Lambda表达式
- Java - 发送邮件
- Java - Applet基础
- Java - Javadoc注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本Jar文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64编码解码
- Java - switch表达式
- Java - Teeing Collectors
- Java - 微基准测试
- Java - 文本块
- Java - 动态CDS归档
- Java - Z垃圾收集器(ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾回收
- Java - JIT编译器
Java 其他
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs和框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 类方法
Java 类方法
类方法是在类中声明的方法。它们执行特定的操作,可以访问和修改类属性。
创建(声明)Java类方法
类方法的声明类似于用户定义的方法声明,只是类方法是在类中声明的。
类方法的声明通过指定访问修饰符、返回类型、方法名和参数列表来实现。
语法
使用以下语法声明Java类方法:
public class class_name { modifier returnType nameOfMethod(Parameter List) { // method body } }
上述语法包括:
修饰符 - 定义方法的访问类型,使用可选。
返回类型 - 类方法的返回数据类型。
方法名 - 这是方法的名称。方法签名由方法名称和参数列表组成。
参数列表 - 参数列表,包括方法的参数类型、顺序和数量。这些是可选的,方法可以包含零个参数。
方法体 - 方法体定义方法使用语句执行的操作。
示例
以下是上面定义的名为minimum()方法的源代码。此方法采用两个参数n1和n2,并返回两者之间的最小值:
class Util { /** the snippet returns the minimum between two numbers */ public int minimum(int n1, int n2) { int min; if (n1 > n2) min = n2; else min = n1; return min; } }
访问Java类方法
要访问类方法(公共类方法),您需要首先创建一个对象,然后使用该对象(借助点(.)运算符)访问类方法。
语法
使用以下语法访问Java公共类方法:
object_name.method_name([parameters]);
示例
下面的示例演示如何定义类方法以及如何访问它。在这里,我们创建了一个Util类的对象,并调用其minimum()方法以获取给定两个数字的最小值:
package com.tutorialspoint; class Util { public int minimum(int n1, int n2) { int min; if (n1 > n2) min = n2; else min = n1; return min; } } public class Tester { public static void main(String[] args) { int a = 11; int b = 6; Util util = new Util(); int c = util.minimum(a, b); System.out.println("Minimum Value = " + c); } }
输出
Minimum value = 6
Java类方法中的this关键字
this是Java中的一个关键字,用作当前类对象的引用,位于实例方法或构造函数内。使用this,您可以引用类的成员,例如构造函数、变量和方法。
注意 - this关键字仅在实例方法或构造函数中使用。
通常,this关键字用于:
在构造函数或方法中,区分实例变量与局部变量(如果它们具有相同的名称)。
class Student { int age; Student(int age) { this.age = age; } }
在一个类中从另一个构造函数(参数化构造函数或默认构造函数)调用一种类型的构造函数。这被称为显式构造函数调用。
class Student { int age Student() { this(20); } Student(int age) { this.age = age; } }
示例:在Java类方法中使用this关键字
这是一个使用this关键字访问类成员的示例。将以下程序复制并粘贴到名为Tester.java的文件中。
package com.tutorialspoint; public class Tester { // Instance variable num int num = 10; Tester() { System.out.println("This is an example program on keyword this"); } Tester(int num) { // Invoking the default constructor this(); // Assigning the local variable num to the instance variable num this.num = num; } public void greet() { System.out.println("Hi Welcome to Tutorialspoint"); } public void print() { // Local variable num int num = 20; // Printing the local variable System.out.println("value of local variable num is : "+num); // Printing the instance variable System.out.println("value of instance variable num is : "+this.num); // Invoking the greet method of a class this.greet(); } public static void main(String[] args) { // Instantiating the class Tester obj1 = new Tester(); // Invoking the print method obj1.print(); // Passing a new value to the num variable through parametrized constructor Tester obj2 = new Tester(30); // Invoking the print method again obj2.print(); } }
输出
This is an example program on keyword this value of local variable num is : 20 value of instance variable num is : 10 Hi Welcome to Tutorialspoint This is an example program on keyword this value of local variable num is : 20 value of instance variable num is : 30 Hi Welcome to Tutorialspoint
公共类方法与静态类方法
类方法有两种类型:公共类方法和静态类方法。公共类方法通过对象访问,而静态类方法无需对象即可访问。您可以直接访问静态方法。
示例
下面的例子演示了公共类方法和静态类方法的区别
public class Main { // Creating a static method static void fun1() { System.out.println("fun1: This is a static method."); } // Creating a public method public void fun2() { System.out.println("fun2: This is a public method."); } // The main() method public static void main(String[] args) { // Accessing static method through the class fun1(); // Creating an object of the Main class Main obj = new Main(); // Accessing public method through the object obj.fun2(); } }
输出
fun1: This is a static method. fun2: This is a public method.
finalize() 方法
可以定义一个方法,在垃圾回收器最终销毁对象之前调用该方法。此方法称为finalize(),可用于确保对象干净地终止。
例如,您可以使用 finalize() 来确保关闭该对象拥有的打开文件。
要向类添加终结器,只需定义 finalize() 方法即可。Java 运行时会在即将回收该类对象时调用该方法。
在 finalize() 方法中,您将指定在销毁对象之前必须执行的操作。
finalize() 方法具有以下一般形式:
protected void finalize( ) { // finalization code here }
这里,关键字 protected 是一个说明符,它防止其类外部定义的代码访问 finalize()。
这意味着您无法知道何时甚至是否会执行 finalize()。例如,如果您的程序在垃圾收集发生之前结束,则不会执行 finalize()。