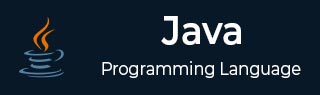
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java vs. C++
- JVM - Java 虚拟机
- Java - JDK vs JRE vs JVM
- Java - Hello World 程序
- Java - 环境设置
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for 循环
- Java - for-each 循环
- Java - while 循环
- Java - do-while 循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多发行版 JAR 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 归档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾收集
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强的 @Deprecated 注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs 和框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 模块系统
在 Java 9 中,引入了模块系统来增强 Java 代码的模块化。模块是对包的一种抽象。这个模块系统也称为JPMS,Java 平台模块系统。它通常被称为模块。
什么是模块?
模块是一个自描述的代码和数据集合,并具有一个名称来标识它。它是一种新型的编程组件,可以包含包、配置和特定于特定功能的资源。模块能够限制对其包含包的访问。默认情况下,模块中包中的代码对外部世界不可见,即使通过反射也不行。从 Java 9 开始,Java 平台本身就是模块化的。如果我们使用 list-modules 命令列出 Java 模块,它将打印 Java 支持的各种模块,如下所示:
C:\Users\Mahesh>java --list-modules [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] [email protected] C:\Users\Mahesh>
在这里我们可以看到,jdk 特定的包在 jdk 模块中,库特定的包在 java 模块中。
Java 模块系统的特性
使用模块组件,在 Java 9 中添加了以下增强功能:
引入了一个新的可选阶段,链接时间。此阶段介于编译时间和运行时间之间。在此阶段,可以组装和优化一组模块,使用 jlink 工具创建自定义运行时映像。
javac、jlink 和 java 具有其他选项来指定模块路径,这些路径进一步定位模块的定义。
JAR 格式更新为模块化 JAR,其根目录中包含 module-info.class 文件。
引入了JMOD 格式,这是一种打包格式(类似于 JAR),可以包含本地代码和配置文件。
声明模块
为了声明一个模块,我们需要在应用程序的根文件夹中创建一个 module-info.java 文件。此文件包含所有元数据或模块描述。
示例
module-info.java
module com.tutorialspoint.greetings { }
添加依赖模块
我们可以在模块中声明其他模块的依赖项。例如,如果我们想使用 com.tutorialspoint.util 模块,我们可以添加如下声明:
示例
module com.tutorialspoint.greetings { requires com.tutorialspoint.util; }
添加可选模块
我们可以使用模块中的 static 关键字声明其他模块的可选依赖项。例如,如果我们想使用 com.tutorialspoint.logging 模块,我们可以添加如下声明:
示例
module com.tutorialspoint.greetings { requires com.tutorialspoint.util; requires static com.tutorialspoint.logging; }
添加传递模块
我们可以使用模块中的 transitive 关键字声明其他模块的传递依赖项。例如,如果我们想将 com.tutorialspoint.base 模块用作 com.tutorialspoint.util 模块的依赖项,我们可以添加如下声明:
示例
module com.tutorialspoint.greetings { requires com.tutorialspoint.util; requires static com.tutorialspoint.logging; requires transitive com.tutorialspoint.base; }
这意味着如果一个模块想要使用 com.tutorialspoint.greetings,那么该模块也需要在模块声明中添加 com.tutorialspoint.base 模块。
导出公共类
默认情况下,模块的包的任何公共类都不会公开给外部世界。为了使用公共类,我们必须像下面这样导出它:
示例
module com.tutorialspoint.greetings { requires com.tutorialspoint.util; requires static com.tutorialspoint.logging; requires transitive com.tutorialspoint.base; exports com.tutorialspoint.greetings.HelloWorld; }
允许反射
默认情况下,模块的包的任何私有成员都不能通过反射访问。为了允许反射检查类或模块,我们必须使用 opens 命令,如下所示:
示例
module com.tutorialspoint.greetings { requires com.tutorialspoint.util; requires static com.tutorialspoint.logging; requires transitive com.tutorialspoint.base; exports com.tutorialspoint.greetings.HelloWorld; opens com.tutorialspoint.greetings.HelloWorld; }
如果我们需要允许整个模块对反射开放,我们可以使用 open 命令,如下所示:
open module com.tutorialspoint.greetings { requires com.tutorialspoint.util; requires static com.tutorialspoint.logging; requires transitive com.tutorialspoint.base; exports com.tutorialspoint.greetings.HelloWorld; }
创建和使用 Java 模块
按照以下步骤创建一个名为 com.tutorialspoint.greetings 的模块。
步骤 1
创建一个文件夹 C:\>JAVA\src。现在创建一个与我们正在创建的模块名称相同的文件夹 com.tutorialspoint.greetings。
步骤 2
在 C:\>JAVA\src\com.tutorialspoint.greetings 文件夹中创建 module-info.java 文件,其中包含以下代码。
module-info.java
module com.tutorialspoint.greetings { }
module-info.java 是用于创建模块的文件。在此步骤中,我们创建了一个名为 com.tutorialspoint.greetings 的模块。按照约定,此文件应该位于名称与模块名称相同的文件夹中。
步骤 3
将源代码添加到模块中。在 C:\>JAVA\src\com.tutorialspoint.greetings\com\tutorialspoint\greetings 文件夹中创建 Java9Tester.java 文件,其中包含以下代码。
Java9Tester.java
package com.tutorialspoint.greetings; public class Java9Tester { public static void main(String[] args) { System.out.println("Hello World!"); } }
按照约定,模块的源代码位于与模块名称相同的目录中。
步骤 4
创建一个文件夹 C:\>JAVA\mods。现在创建一个与我们创建的模块名称相同的文件夹 com.tutorialspoint.greetings。现在将模块编译到 mods 目录。
C:/ > JAVA > javac -d mods/com.tutorialspoint.greetings src/com.tutorialspoint.greetings/module-info.java src/com.tutorialspoint.greetings/com/tutorialspoint/greetings/Java9Tester.java
步骤 5
让我们运行模块以查看结果。运行以下命令。
C:/>JAVA>java --module-path mods -m com.tutorialspoint.greetings/com.tutorialspoint.greetings.Java9Tester
此处 module-path 提供模块位置为 mods,-m 表示主模块。
它将在控制台打印以下输出。
Hello World!