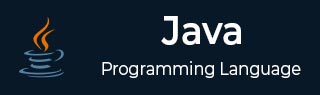
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java vs. C++
- JVM - Java虚拟机
- Java - JDK vs JRE vs JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期与时间
Java 控制语句
- Java - 循环控制
- Java - 决策机制
- Java - if-else
- Java - switch
- Java - for 循环
- Java - for-each 循环
- Java - while 循环
- Java - do-while 循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs 概念
- Java - 对象与类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造器
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造器
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误与异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重catch块
- Java - 嵌套try块
- Java - finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - Socket编程
- Java - URL处理
- Java - URL类
- Java - URLConnection类
- Java - HttpURLConnection类
- Java - Socket类
- Java -泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级Java
- Java - 命令行参数
- Java - Lambda表达式
- Java - 发送邮件
- Java - Applet基础
- Java - Javadoc注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本Jar文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64编码解码
- Java - switch表达式
- Java - Teeing收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态CDS归档
- Java - Z垃圾收集器(ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁的数字格式化
- Java - 垃圾回收
- Java - JIT编译器
Java 其他
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强的@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs与框架
Java类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - continue 语句
Java continue 语句
continue语句可以用于任何循环控制结构。它会导致循环立即跳转到循环的下一个迭代。
在for循环中,continue关键字导致控制立即跳转到更新语句。
在while循环或do/while循环中,控制立即跳转到布尔表达式。
语法
continue的语法是在任何循环内的一个单一语句:
continue;
流程图
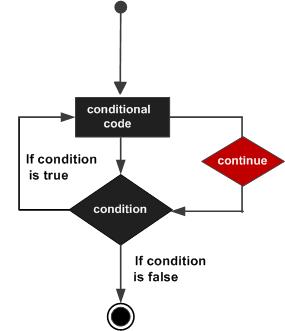
例子
示例1:在while循环中使用continue
在这个例子中,我们展示了如何使用continue语句跳过while循环中值为15的元素,该循环用于打印10到19的元素。这里我们用值为10的int 变量 x进行了初始化。然后在while循环中,我们检查x是否小于20,并在while循环内打印x的值并将x的值加1。while循环将运行直到x变为15。一旦x为15,continue语句将跳过while循环的执行体,循环继续。
public class Test { public static void main(String args[]) { int x = 10; while( x < 20 ) { x++; if(x == 15){ continue; } System.out.print("value of x : " + x ); System.out.print("\n"); } } }
输出
value of x : 11 value of x : 12 value of x : 13 value of x : 14 value of x : 16 value of x : 17 value of x : 18 value of x : 19 value of x : 20
示例2:在for循环中使用continue
在这个例子中,我们展示了如何在for循环中使用continue语句跳过要打印的数组的元素。这里我们创建一个整数数组numbers并初始化一些值。我们在for循环中创建了一个名为index的变量来表示数组的索引,检查它是否小于数组的大小并将其加1。在for循环体中,我们使用索引表示法打印数组的元素。一旦遇到值为30,continue语句就会跳转到for循环的更新部分,循环继续。
public class Test { public static void main(String args[]) { int [] numbers = {10, 20, 30, 40, 50}; for(int index = 0; index < numbers.length; index++) { if(numbers[index] == 30){ continue; } System.out.print("value of item : " + numbers[index] ); System.out.print("\n"); } } }
输出
value of item : 10 value of item : 20 value of item : 40 value of item : 50
示例3:在do while循环中使用continue
在这个例子中,我们展示了如何使用continue语句跳过do while循环中值为15的元素,该循环用于打印10到19的元素。这里我们用值为10的int变量x进行了初始化。然后在do while循环中,我们在循环体之后检查x是否小于20,并在while循环内打印x的值并将x的值加1。while循环将运行直到x变为15。一旦x为15,continue语句将跳过while循环的执行体,循环继续。
public class Test { public static void main(String args[]) { int x = 10; do { x++; if(x == 15){ continue; } System.out.print("value of x : " + x ); System.out.print("\n"); } while( x < 20 ); } }
输出
value of x : 11 value of x : 12 value of x : 13 value of x : 14 value of x : 16 value of x : 17 value of x : 18 value of x : 19 value of x : 20
java_loop_control.htm
广告