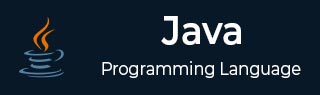
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境设置
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重捕获块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 停止钩子
Java 同步
Java 网络
- Java - 网络
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 归档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 紧凑数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - 进程 API 改进
- Java - 流 API 改进
- Java - 增强 @Deprecated 注解
- Java - CompletableFuture API 改进
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - JVM 停止钩子
JVM 关闭
以下是两种不同的 JVM 关闭方式:
受控过程:如果调用了System.exit() 方法,按下 CTRL+C,或最后一个非守护线程终止,则 JVM 开始关闭其进程。
突然方式:如果 JVM 收到终止信号,调用了Runtime.getRuntime().halt() 方法,或发生任何类型的操作系统恐慌,则 JVM 开始关闭其进程。
JVM 停止钩子
停止钩子只是一个已初始化但未启动的线程。当虚拟机开始其关闭序列时,它将以某种未指定的顺序启动所有已注册的停止钩子,并让它们并发运行。当所有钩子都完成后,它将运行所有未调用的终结器(如果已启用退出时的终结)。最后,虚拟机将停止。请注意,守护线程将在关闭序列期间继续运行,如果通过调用 exit 方法启动关闭,则非守护线程也将继续运行。
JVM 停止钩子:addShutdownHook(Thread hook) 方法
该Runtime addShutdownHook(Thread hook) 方法注册一个新的虚拟机停止钩子。
声明
以下是java.lang.Runtime.addShutdownHook()方法的声明
public void addShutdownHook(Thread hook)
参数
hook - 一个已初始化但未启动的 Thread 对象。
返回值
此方法不返回值。
异常
IllegalArgumentException - 如果指定的钩子已注册,或者可以确定钩子已在运行或已运行。
IllegalStateException - 如果虚拟机已在关闭过程中。
SecurityException - 如果存在安全管理器并且它拒绝 RuntimePermission("shutdownHooks")。
JVM 停止钩子的示例
在此示例中,我们通过扩展 Thread 类创建一个名为 CustomThread 的类。此线程对象将用作 JVM 停止钩子。CustomThread 类具有 run() 方法的实现。在主类 TestThread 中,我们使用 Runtime.getRuntime().addShutdownHook() 方法添加了一个停止钩子,方法是将线程对象传递给它。在输出中,您可以验证当程序即将退出时是否调用了 CustomThread run() 方法。
package com.tutorialspoint; class CustomThread extends Thread { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { // register CustomThread as shutdown hook Runtime.getRuntime().addShutdownHook(new CustomThread()); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); } catch (Exception e) { e.printStackTrace(); } } }
输出
Program is starting... Waiting for 3 seconds... Program is closing... JVM is shutting down.
更多 JVM 停止钩子的示例
示例 1
在此示例中,我们通过实现 Runnable 接口创建一个名为 CustomThread 的类。此线程对象将用作 JVM 停止钩子。CustomThread 类具有 run() 方法的实现。在主类 TestThread 中,我们使用 Runtime.getRuntime().addShutdownHook() 方法添加了一个停止钩子,方法是将线程对象传递给它。在输出中,您可以验证当程序即将退出时是否调用了 CustomThread run() 方法。
package com.tutorialspoint; class CustomThread implements Runnable { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { // register CustomThread as shutdown hook Runtime.getRuntime().addShutdownHook(new Thread(new CustomThread())); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); } catch (Exception e) { e.printStackTrace(); } } }
输出
Program is starting... Waiting for 3 seconds... Program is closing... JVM is shutting down.
示例 2
我们也可以使用 removeShutdownHook() 删除停止钩子。在此示例中,我们通过实现 Runnable 接口创建一个名为 CustomThread 的类。此线程对象将用作 JVM 停止钩子。CustomThread 类具有 run() 方法的实现。在主类 TestThread 中,我们使用 Runtime.getRuntime().addShutdownHook() 方法添加了一个停止钩子,方法是将线程对象传递给它。作为最后一条语句,我们使用 Runtime.getRuntime().removeShutdownHook() 方法删除了钩子。
package com.tutorialspoint; class CustomThread implements Runnable { public void run() { System.out.println("JVM is shutting down."); } } public class TestThread { public static void main(String args[]) throws InterruptedException { try { Thread hook = new Thread(new CustomThread()); // register Message as shutdown hook Runtime.getRuntime().addShutdownHook(hook); // print the state of the program System.out.println("Program is starting..."); // cause thread to sleep for 3 seconds System.out.println("Waiting for 3 seconds..."); Thread.sleep(3000); // print that the program is closing System.out.println("Program is closing..."); Runtime.getRuntime().removeShutdownHook(hook); } catch (Exception e) { e.printStackTrace(); } } }
输出
Program is starting... Waiting for 3 seconds... Program is closing...