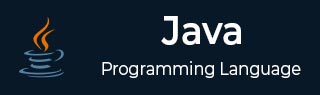
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期与时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for 循环
- Java - for-each 循环
- Java - while 循环
- Java - do-while 循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs 概念
- Java - 对象与类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误与异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - Socket 编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java -泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 归档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁的数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强的 @Deprecated 注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs 和框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 数据结构
由 Java 实用程序包 提供的数据结构非常强大,并执行各种功能。这些数据结构包括以下接口和类:
- 枚举
- BitSet
- Vector
- Stack
- Dictionary
- Hashtable
- Properties
所有这些类现在都是遗留的,Java-2 引入了一个名为 Collections Framework 的新框架,这将在下一章中讨论。
枚举
Enumeration 接口 本身不是数据结构,但在其他数据结构的上下文中非常重要。Enumeration 接口定义了一种从数据结构中检索连续元素的方法。
例如,Enumeration 定义了一个名为 nextElement 的方法,用于获取包含多个元素的数据结构中的下一个元素。
示例
以下是一个显示 Vector 枚举用法的示例。
import java.util.Vector; import java.util.Enumeration; public class EnumerationTester { public static void main(String args[]) { Enumeration<String> days; Vector<String> dayNames = new Vector<>(); dayNames.add("Sunday"); dayNames.add("Monday"); dayNames.add("Tuesday"); dayNames.add("Wednesday"); dayNames.add("Thursday"); dayNames.add("Friday"); dayNames.add("Saturday"); days = dayNames.elements(); while (days.hasMoreElements()) { System.out.println(days.nextElement()); } } }
输出
Sunday Monday Tuesday Wednesday Thursday Friday Saturday
要详细了解此接口,请查看 枚举。
BitSet
BitSet 类 实现了一组可以单独设置和清除的位或标志。
当您需要跟进一组布尔值时,此类非常有用;您只需为每个值分配一位,并根据需要设置或清除它。
示例
以下程序说明了 BitSet 数据结构支持的几种方法。
import java.util.BitSet; public class BitSetDemo { public static void main(String args[]) { BitSet bits1 = new BitSet(16); BitSet bits2 = new BitSet(16); // set some bits for(int i = 0; i < 16; i++) { if((i % 2) == 0) bits1.set(i); if((i % 5) != 0) bits2.set(i); } System.out.println("Initial pattern in bits1: "); System.out.println(bits1); System.out.println("\nInitial pattern in bits2: "); System.out.println(bits2); // AND bits bits2.and(bits1); System.out.println("\nbits2 AND bits1: "); System.out.println(bits2); // OR bits bits2.or(bits1); System.out.println("\nbits2 OR bits1: "); System.out.println(bits2); // XOR bits bits2.xor(bits1); System.out.println("\nbits2 XOR bits1: "); System.out.println(bits2); } }
输出
Initial pattern in bits1: {0, 2, 4, 6, 8, 10, 12, 14} Initial pattern in bits2: {1, 2, 3, 4, 6, 7, 8, 9, 11, 12, 13, 14} bits2 AND bits1: {2, 4, 6, 8, 12, 14} bits2 OR bits1: {0, 2, 4, 6, 8, 10, 12, 14} bits2 XOR bits1: {}
Vector
Vector 类 类似于传统的 Java 数组,不同之处在于它可以根据需要增长以容纳新元素。
与数组一样,Vector 对象的元素可以通过向量中的索引访问。
使用 Vector 类的好处是,您不必担心在创建时将其设置为特定大小;它会在需要时自动收缩和增长。
示例
以下程序说明了 Vector 集合支持的几种方法。
import java.util.*; public class VectorDemo { public static void main(String args[]) { // initial size is 3, increment is 2 Vector v = new Vector(3, 2); System.out.println("Initial size: " + v.size()); System.out.println("Initial capacity: " + v.capacity()); v.addElement(new Integer(1)); v.addElement(new Integer(2)); v.addElement(new Integer(3)); v.addElement(new Integer(4)); System.out.println("Capacity after four additions: " + v.capacity()); v.addElement(new Double(5.45)); System.out.println("Current capacity: " + v.capacity()); v.addElement(new Double(6.08)); v.addElement(new Integer(7)); System.out.println("Current capacity: " + v.capacity()); v.addElement(new Float(9.4)); v.addElement(new Integer(10)); System.out.println("Current capacity: " + v.capacity()); v.addElement(new Integer(11)); v.addElement(new Integer(12)); System.out.println("First element: " + (Integer)v.firstElement()); System.out.println("Last element: " + (Integer)v.lastElement()); if(v.contains(new Integer(3))) System.out.println("Vector contains 3."); // enumerate the elements in the vector. Enumeration vEnum = v.elements(); System.out.println("\nElements in vector:"); while(vEnum.hasMoreElements()) System.out.print(vEnum.nextElement() + " "); System.out.println(); } }
输出
Initial size: 0 Initial capacity: 3 Capacity after four additions: 5 Current capacity: 5 Current capacity: 7 Current capacity: 9 First element: 1 Last element: 12 Vector contains 3. Elements in vector: 1 2 3 4 5.45 6.08 7 9.4 10 11 12
Stack
Stack 类实现元素的后进先出 (LIFO) 堆栈。
您可以将堆栈从字面上理解为对象的垂直堆栈;当您添加新元素时,它会堆叠在其他元素之上。
当您从堆栈中取出一个元素时,它会从顶部取出。换句话说,您添加到堆栈的最后一个元素是第一个被取出的元素。
示例
以下程序说明了 Stack 集合支持的几种方法。
import java.util.*; public class StackDemo { static void showpush(Stack st, int a) { st.push(new Integer(a)); System.out.println("push(" + a + ")"); System.out.println("stack: " + st); } static void showpop(Stack st) { System.out.print("pop -> "); Integer a = (Integer) st.pop(); System.out.println(a); System.out.println("stack: " + st); } public static void main(String args[]) { Stack st = new Stack(); System.out.println("stack: " + st); showpush(st, 42); showpush(st, 66); showpush(st, 99); showpop(st); showpop(st); showpop(st); try { showpop(st); } catch (EmptyStackException e) { System.out.println("empty stack"); } } }
输出
stack: [ ] push(42) stack: [42] push(66) stack: [42, 66] push(99) stack: [42, 66, 99] pop -> 99 stack: [42, 66] pop -> 66 stack: [42] pop -> 42 stack: [ ] pop -> empty stack
Dictionary
Dictionary 类是一个抽象类,它定义了将键映射到值的数据结构。
这在您希望能够通过特定键而不是整数索引访问数据的情况下很有用。
由于 Dictionary 类是抽象的,因此它只提供键映射数据结构的框架,而不是具体的实现。
示例
以下示例显示了 Java Dictionary keys() 方法的用法。我们使用 Integer、Integer 的 Hashtable 对象创建字典实例。然后我们向其中添加了一些元素。使用 keys() 方法检索枚举,然后迭代枚举以打印字典的键。
package com.tutorialspoint; import java.util.Enumeration; import java.util.Dictionary; import java.util.Hashtable; public class DictionaryDemo { public static void main(String[] args) { // create a new hashtable Dictionary<Integer, Integer> dictionary = new Hashtable<>(); // add 2 elements dictionary.put(1, 1); dictionary.put(2, 2); Enumeration<Integer> enumeration = dictionary.keys(); while(enumeration.hasMoreElements()) { System.out.println(enumeration.nextElement()); } } }
输出
2 1
Hashtable
Hashtable 类提供了一种根据某些用户定义的键结构组织数据的方法。
例如,在地址列表哈希表中,您可以根据邮政编码等键而不是人的姓名来存储和排序数据。
哈希表中键的具体含义完全取决于哈希表的用途及其包含的数据。
示例
以下示例演示了如何使用 Java Hashtable 的 contains() 方法来检查哈希表中是否存在某个值。我们创建了一个 Integer,Integer 类型的 Hashtable 对象。然后添加了一些条目,打印表格,并使用 contains() 方法检查表格中的两个值。
package com.tutorialspoint; import java.util.Hashtable; public class HashtableDemo { public static void main(String args[]) { // create hash table Hashtable<Integer,Integer> hashtable = new Hashtable<>(); // populate hash table hashtable.put(1, 1); hashtable.put(2, 2); hashtable.put(3, 3); System.out.println("Initial table elements: " + hashtable); System.out.println("Hashtable contains 2 as value: " + hashtable.contains(2)); System.out.println("Hashtable contains 4 as value: " + hashtable.contains(4)); } }
输出
Initial table elements: {3=3, 2=2, 1=1} Hashtable contains 2 as value: true Hashtable contains 4 as value: false
属性
Properties 是 Hashtable 的一个子类。它用于维护值列表,其中键是字符串,值也是字符串。
许多其他 Java 类都使用 Properties 类。例如,在获取环境值时,System.getProperties() 返回的对象就是这种类型。
示例
以下示例演示了如何使用 Java Properties 的 getProperty(String key) 方法根据键从 Properties 中获取值。我们创建了一个 Properties 对象。然后添加了一些条目。使用 getProperty() 方法检索并打印值。
package com.tutorialspoint; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties properties = new Properties(); //populate properties object properties.put("1", "tutorials"); properties.put("2", "point"); properties.put("3", "is best"); System.out.println("Properties elements: " + properties); System.out.println("Value: " + properties.getProperty("1")); } }
输出
Properties elements: {1=tutorials, 2=point, 3=is best} Value: tutorials