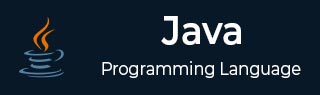
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重捕获块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 合并线程
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络
- Java - 网络
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 存档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 紧凑数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API 增强
- Java - Stream API 增强
- Java - 增强的 @Deprecated 注解
- Java - CompletableFuture API 增强
- Java - 流
- Java - Datetime Api
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 密封类和接口
Java 15 引入了一个密封类作为预览功能,它提供了对继承的细粒度控制。Java 16 提供了一些小的增强功能,并将此功能保留为预览。随着 Java 17 的发布,密封类和接口成为了标准特性。密封类/接口功能添加到 Java 中,为开发人员提供了对继承的细粒度控制。密封类可以定义允许扩展它的子类型,而其他类则不能扩展它。
以下是需要考虑的密封类的要点:
- 密封类使用 sealed 关键字声明。
- 密封类允许使用 permits 关键字声明哪些类可以是子类型。
- 扩展密封类的类必须声明为 sealed、non-sealed 或 final。
- 密封类有助于在继承中创建有限且可确定的类层次结构。
密封接口
一个接口可以使用 sealed 关键字标记为密封接口,然后使用permits关键字,我们可以添加可以扩展此接口的接口。
public sealed interface Person permits Employee, Manager { }
密封接口示例
在此示例中,我们创建了一个密封接口 Person,它允许 Employee 和 Manager 接口扩展它。Employee 和 Manager 接口具有不同的方法来获取人员的 ID。现在,为了获取人员的 ID,我们使用 instanceof 运算符检查实例是否为 Employee 或 Manager 并获取相应的 ID。因此,我们可以看到,预先知道允许的接口有助于在这种情况下进行开发。
package com.tutorialspoint; public class Tester { public static void main(String[] args) { // create an instance of Manager Person manager = new CorpManager(23, "Robert"); // get the id System.out.println("Id: " + getId(manager)); } public static int getId(Person person) { // check if person is employee then return employee id if (person instanceof Employee) { return ((Employee) person).getEmployeeId(); } // if person is manager then return manager id else if (person instanceof Manager) { return ((Manager) person).getManagerId(); } return -1; } } // a sealed interface Person which is to be inherited by Employee // and Manager interfaces sealed interface Person permits Employee, Manager { String getName(); } // Employee and Manager interfaces have to extend Person and can be sealed or non-sealed non-sealed interface Employee extends Person { int getEmployeeId(); } non-sealed interface Manager extends Person { int getManagerId(); } class CorpEmployee implements Employee { String name; int id; public CorpEmployee(int id,String name) { this.name = name; this.id = id; } public String getName() { return name; } public int getEmployeeId() { return id; } } class CorpManager implements Manager { String name; int id; public CorpManager(int id,String name) { this.name = name; this.id = id; } public String getName() { return name; } public int getManagerId() { return id; } }
让我们编译并运行上述程序,这将产生以下结果:
Id: 23
密封类
与密封接口类似,类也可以使用sealed关键字标记为密封类,然后使用 permits 关键字,我们可以添加可以扩展此类的子类。
public abstract sealed class Person permits Employee, Manager { }
子类需要具有 sealed/final 或 non-sealed 修饰符。
public final class Manager extends Person { }
具有 non-sealed 修饰符的子类对所有类开放以进行扩展。
public non-sealed class Employee extends Person { }
约束
在扩展密封类时,我们应该注意使用密封类的一些约束。
允许的子类应与密封类位于同一模块中。
允许的子类必须扩展密封类。
允许的子类必须使用 final、sealed 或 non-sealed 修饰符之一。
密封类示例
在此示例中,我们创建了一个密封抽象类 Person,它允许 Employee 和 Manager 类扩展它。Employee 和 Manager 类具有不同的方法来获取人员的 ID。现在,为了获取人员的 ID,我们使用 instanceof 运算符检查实例是否为 Employee 或 Manager 并获取相应的 ID。因此,我们可以看到,预先知道允许的子类有助于在这种情况下进行开发。
package com.tutorialspoint; public class Tester { public static void main(String[] args) { // create an instance of Manager Person manager = new Manager(23, "Robert"); // get the id System.out.println("Id: " + getId(manager)); } public static int getId(Person person) { // check if person is employee then return employee id if (person instanceof Employee) { return ((Employee) person).getEmployeeId(); } // if person is manager then return manager id else if (person instanceof Manager) { return ((Manager) person).getManagerId(); } return -1; } } // a sealed class Person which is to be inherited by Employee // and Manager classes abstract sealed class Person permits Employee, Manager { String name; String getName() { return name; } } // Employee class has to extend Person and should have a modifier final class Employee extends Person { String name; int id; Employee(int id, String name){ this.id = id; this.name = name; } int getEmployeeId() { return id; } } // We can mark a sub-class as non-sealed, so that it can be extended if required non-sealed class Manager extends Person { int id; Manager(int id, String name){ this.id = id; this.name = name; } int getManagerId() { return id; } }
让我们编译并运行上述程序,这将产生以下结果:
Id: 23