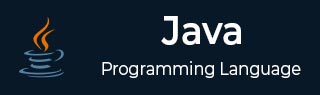
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK、JRE 和 JVM 的区别
- Java - HelloWorld 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for 循环
- Java - for-each 循环
- Java - while 循环
- Java - do-while 循环
- Java - break
- Java - continue
面向对象编程
- Java - 面向对象概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - Socket 编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 归档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾收集
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs 和框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 动态绑定
绑定是一种在方法调用和方法实际实现之间创建链接的机制。根据Java 中的多态性概念,对象可以具有许多不同的形式。对象的形态可以在编译时和运行时解析。
Java 动态绑定
动态绑定是指在运行时解析方法调用和方法实现之间链接的过程(或者,在运行时调用重写方法的过程)。动态绑定也称为运行时多态性或后期绑定。动态绑定使用对象来解析绑定。
Java 动态绑定的特性
链接- 方法调用和方法实现之间的链接在运行时解析。
解析机制- 动态绑定使用对象类型来解析绑定。
示例- 方法重写是动态绑定的示例。
方法类型- 虚方法使用动态绑定。
Java 动态绑定的示例
在这个例子中,我们创建了两个类 Animal 和 Dog,其中 Dog 类扩展了 Animal 类。在 main() 方法中,我们使用 Animal 类引用并为其分配 Dog 类的一个对象来检查动态绑定的效果。
package com.tutorialspoint; class Animal { public void move() { System.out.println("Animals can move"); } } class Dog extends Animal { public void move() { System.out.println("Dogs can walk and run"); } } public class Tester { public static void main(String args[]) { Animal a = new Animal(); // Animal reference and object // Dynamic Binding Animal b = new Dog(); // Animal reference but Dog object a.move(); // runs the method in Animal class b.move(); // runs the method in Dog class } }
输出
Animals can move Dogs can walk and run
在上面的例子中,您可以看到,即使b是 Animal 类型,它也会运行 Dog 类中的 move 方法。其原因是:在编译时,检查是在引用类型上进行的。但是,在运行时,JVM 会确定对象类型,并运行属于该特定对象的方法。
因此,在上面的例子中,程序将正确编译,因为 Animal 类具有 move 方法。然后,在运行时,它运行特定于该对象的方法。
Java 动态绑定:使用 super 关键字
当调用重写方法的超类版本时,使用super关键字,以便在使用动态绑定时可以使用父类方法。
示例:使用 super 关键字
在这个例子中,我们创建了两个类 Animal 和 Dog,其中 Dog 类扩展了 Animal 类。Dog 类重写了其超类 Animal 的 move 方法。但是它使用 super 关键字调用父 move() 方法,以便由于动态绑定调用子方法时,会调用这两个 move 方法。在 main() 方法中,我们使用 Animal 类引用并为其分配 Dog 类的一个对象来检查动态绑定的效果。
class Animal { public void move() { System.out.println("Animals can move"); } } class Dog extends Animal { public void move() { super.move(); // invokes the super class method System.out.println("Dogs can walk and run"); } } public class TestDog { public static void main(String args[]) { Animal b = new Dog(); // Animal reference but Dog object b.move(); // runs the method in Dog class } }
输出
Animals can move Dogs can walk and run
广告