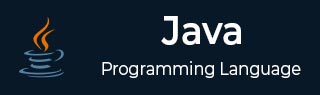
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK、JRE 和 JVM 的区别
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造方法
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造方法
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送电子邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 归档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - 进程 API 改进
- Java - Stream API 改进
- Java - 增强的 @Deprecated 注解
- Java - CompletableFuture API 改进
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - 使用 JavaDoc 工具生成文档
本章主要讲解 Javadoc。我们将了解如何使用 Javadoc 为 Java 代码生成有用的文档。
Java 文档可以使用 javadoc 工具生成。它目前生成 html 4.0 格式的文档。从 Java 9 开始,我们可以通过在命令行参数中使用 -html5 选项来生成 html 5 格式的文档。
什么是 Javadoc?
Javadoc 是一个随 JDK 提供的工具,用于从 Java 源代码生成 HTML 格式的 Java 代码文档,这些源代码需要以预定义的格式进行文档记录。
下面是一个简单的示例,其中 /*….*/ 内部的行是 Java 多行注释。类似地,// 前面的行是 Java 单行注释。
示例
/** * The HelloWorld program implements an application that * simply displays "Hello World!" to the standard output. * * @author Zara Ali * @version 1.0 * @since 2014-03-31 */ public class HelloWorld { public static void main(String[] args) { // Prints Hello, World! on standard output. System.out.println("Hello World!"); } }
输出
Hello World!
您可以在描述部分包含所需的 HTML 标签。例如,以下示例使用 <h1>....</h1> 用于标题,<p> 用于创建段落换行符 -
示例
/** * <h1>Hello, World!</h1> * The HelloWorld program implements an application that * simply displays "Hello World!" to the standard output. * <p> * Giving proper comments in your program makes it more * user friendly and it is assumed as a high quality code. * * * @author Zara Ali * @version 1.0 * @since 2014-03-31 */ public class HelloWorld { public static void main(String[] args) { // Prints Hello, World! on standard output. System.out.println("Hello World!"); } }
输出
Hello World!
Javadoc 标签
Javadoc 工具识别以下标签 -
标签 | 描述 | 语法 |
---|---|---|
@author | 添加类的作者。 | @author name-text |
{@code} | 以代码字体显示文本,而不将其解释为 HTML 标记或嵌套的 javadoc 标签。 | {@code text} |
{@docRoot} | 表示从任何生成的页面到生成的文档根目录的相对路径。 | {@docRoot} |
@deprecated | 添加一条注释,指示不再应使用此 API。 | @deprecated deprecatedtext |
@exception | 在生成的文档中添加一个“Throws”小标题,其中包含类名和描述文本。 | @exception class-name description |
{@inheritDoc} | 从最近的可继承类或可实现接口继承注释。 | 从直接父类继承注释。 |
{@link} | 插入一个内联链接,该链接具有指向指定包、类或引用的类的成员名称的文档的可见文本标签。 | {@link package.class#member label} |
{@linkplain} | 与 {@link} 相同,只是链接的标签以纯文本而不是代码字体显示。 | {@linkplain package.class#member label} |
@param | 在“参数”部分添加一个参数,该参数后跟指定的 parameter-name 和描述。 | @param parameter-name description |
@return | 添加一个“返回值”部分,其中包含描述文本。 | @return description |
@see | 添加一个“另请参见”标题,其中包含指向引用的链接或文本条目。 | @see reference |
@serial | 用于默认可序列化字段的文档注释中。 | @serial field-description | include | exclude |
@serialData | 记录由 writeObject( ) 或 writeExternal( ) 方法写入的数据。 | @serialData data-description |
@serialField | 记录 ObjectStreamField 组件。 | @serialField field-name field-type field-description |
@since | 在生成的文档中添加一个“自”标题,其中包含指定的 since-text。 | @since release |
@throws | @throws 和 @exception 标签是同义词。 | @throws class-name description |
{@value} | 当 {@value} 用于静态字段的文档注释中时,它会显示该常量的值。 | {@value package.class#field} |
@version | 当使用 -version 选项时,在生成的文档中添加一个“版本”小标题,其中包含指定的 version-text。 | @version version-text |
示例 - 使用旧版 Java 文档
以下程序使用了文档注释中的一些重要标签。您可以根据需要使用其他标签。
有关 AddNum 类的文档将生成在 HTML 文件 AddNum.html 中,但同时也会创建一个名为 index.html 的主文件。
import java.io.*; /** * <h1>Add Two Numbers!</h1> * The AddNum program implements an application that * simply adds two given integer numbers and Prints * the output on the screen. * <p> * <b>Note:</b> Giving proper comments in your program makes it more * user friendly and it is assumed as a high quality code. * * @author Zara Ali * @version 1.0 * @since 2014-03-31 */ public class AddNum { /** * This method is used to add two integers. This is * a the simplest form of a class method, just to * show the usage of various javadoc Tags. * @param numA This is the first paramter to addNum method * @param numB This is the second parameter to addNum method * @return int This returns sum of numA and numB. */ public int addNum(int numA, int numB) { return numA + numB; } /** * This is the main method which makes use of addNum method. * @param args Unused. * @return Nothing. * @exception IOException On input error. * @see IOException */ public static void main(String args[]) throws IOException { AddNum obj = new AddNum(); int sum = obj.addNum(10, 20); System.out.println("Sum of 10 and 20 is :" + sum); } }
让我们编译并运行上述程序,这将产生以下结果 -
Sum of 10 and 20 is :30
现在,使用 javadoc 实用程序处理上述 AddNum.java 文件,如下所示 -
$ javadoc AddNum.java Loading source file AddNum.java... Constructing Javadoc information... Standard Doclet version 1.7.0_51 Building tree for all the packages and classes... Generating /AddNum.html... AddNum.java:36: warning - @return tag cannot be used in method with void return type. Generating /package-frame.html... Generating /package-summary.html... Generating /package-tree.html... Generating /constant-values.html... Building index for all the packages and classes... Generating /overview-tree.html... Generating /index-all.html... Generating /deprecated-list.html... Building index for all classes... Generating /allclasses-frame.html... Generating /allclasses-noframe.html... Generating /index.html... Generating /help-doc.html... 1 warning $
您可以在此处查看所有生成的文档 - AddNum。如果您使用的是 JDK 1.7,则 javadoc 不会生成很好的 stylesheet.css,因此我们建议您从 https://docs.oracle.com 下载并使用标准样式表
示例 - 使用旧版 Java 文档
使用 -html5 标志运行 jdk 9 的 javadoc 工具以生成新型文档。
$ javadoc -html5 AddNum.java Loading source file AddNum.java... Constructing Javadoc information... Building index for all the packages and classes... Standard Doclet version 21.0.2+13-LTS-58 Building tree for all the packages and classes... Generating .\AddNum.html... AddNum.java:32: error: invalid use of @return * @return Nothing. ^ AddNum.java:16: warning: use of default constructor, which does not provide a comment public class AddNum { ^ Generating .\package-summary.html... Generating .\package-tree.html... Generating .\overview-tree.html... Building index for all classes... Generating .\allclasses-index.html... Generating .\allpackages-index.html... Generating .\index-all.html... Generating .\search.html... Generating .\index.html... Generating .\help-doc.html... 1 error 1 warning $
它将创建一个如下所示的文档
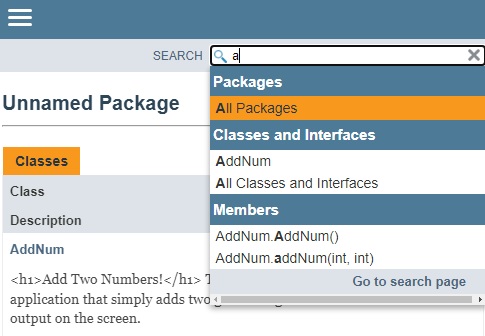
广告