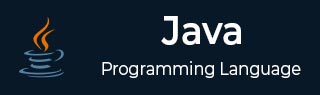
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java vs. C++
- JVM - Java虚拟机
- Java - JDK vs JRE vs JVM
- Java - Hello World程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for循环
- Java - for-each循环
- Java - while循环
- Java - do-while循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造器
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态性
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造器
- Java - 枚举字符串
Java内置类
Java文件处理
Java错误和异常
- Java - 异常
- Java - try-catch块
- Java - try-with-resources
- Java - 多catch块
- Java - 嵌套try块
- Java - finally块
- Java - throw异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java同步
Java网络编程
- Java - 网络编程
- Java - 套接字编程
- Java - URL处理
- Java - URL类
- Java - URLConnection类
- Java - HttpURLConnection类
- Java - Socket类
- Java - 泛型
Java集合
Java接口
Java数据结构
Java集合算法
高级Java
- Java - 命令行参数
- Java - Lambda表达式
- Java - 发送邮件
- Java - Applet基础
- Java - Javadoc注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本Jar文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64编码解码
- Java - switch表达式
- Java - Teeing收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态CDS归档
- Java - Z垃圾收集器(ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁数字格式化
- Java - 垃圾收集
- Java - JIT编译器
Java杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs和框架
Java类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java有用资源
Java - finally关键字
Java finally 关键字用于在 Java 程序中定义 finally 块。Java 中的 finally 块位于 try 块或 catch 块之后。无论 Java 程序中是否发生异常,finally 代码块始终都会执行。
使用 Java finally 块允许你运行任何你想要执行的清理型语句,无论受保护的代码中发生了什么。
finally 块出现在 Java 程序中 catch 块的末尾,并具有以下语法:
语法
try { // Protected code } catch (ExceptionType1 e1) { // Catch block } catch (ExceptionType2 e2) { // Catch block } catch (ExceptionType3 e3) { // Catch block }finally { // The finally block always executes. }
示例
在这个例子中,我们使用无效索引访问数组的元素。当程序运行时,它会抛出 ArrayIndexOutOfBoundsException。由于我们在 catch 块中处理了此异常,因此也会执行以下 finally 块。
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) { int a[] = new int[2]; try { System.out.println("Access element three :" + a[3]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Exception thrown :" + e); }finally { a[0] = 6; System.out.println("First element value: " + a[0]); System.out.println("The finally statement is executed"); } } }
输出
Exception thrown :java.lang.ArrayIndexOutOfBoundsException: 3 First element value: 6 The finally statement is executed
示例
在这个例子中,我们使用无效索引访问数组的元素。当程序运行时,它会抛出 ArrayIndexOutOfBoundsException。由于我们在catch块中处理了此异常,并进一步抛出异常,因此finally块仍然会被执行。
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) throws CustomException { int a[] = new int[2]; try { System.out.println("Access element three :" + a[3]); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Exception thrown :" + e); throw new CustomException(e); }finally { a[0] = 6; System.out.println("First element value: " + a[0]); System.out.println("The finally statement is executed"); } } } class CustomException extends Exception{ private static final long serialVersionUID = 1L; CustomException(Exception e){ super(e); } }
输出
Exception thrown :java.lang.ArrayIndexOutOfBoundsException: 3 Exception in thread "main" First element value: 6 The finally statement is executed com.tutorialspoint.CustomException: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.main(ExcepTest.java:12) Caused by: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.main(ExcepTest.java:9)
注意以下几点:
catch 子句不能没有 try 语句。
并非每次 try/catch 块都必须有 finally 子句。
try 块不能没有 catch 子句或 finally 子句。
try、catch、finally 块之间不能有任何代码。
java_basic_syntax.htm
广告