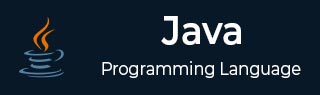
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境设置
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络
- Java - 网络
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 存档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 紧凑数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API 增强
- Java - Stream API 增强
- Java - 增强 @Deprecated 注解
- Java - CompletableFuture API 增强
- Java - 流
- Java - Datetime Api
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - HttpURLConnection getErrorStream()
Java HttpURLConnection getErrorStream() 方法在连接失败但服务器仍然发送了有用的数据时返回错误流。典型的例子是当 HTTP 服务器响应 404 时,这将导致在连接中抛出 FileNotFoundException,但服务器发送了一个 HTML 帮助页面,其中包含有关如何操作的建议。
此方法不会导致连接启动。如果连接未连接,或者服务器在连接过程中没有错误,或者服务器有错误但没有发送错误数据,则此方法将返回 null。这是默认值。
声明
以下是 java.net.HttpURLConnection.getErrorStream() 方法的声明
public InputStream getErrorStream()
参数
无
返回值
如果有错误流,则返回错误流;如果没有错误、连接未连接或服务器未发送任何有用的数据,则返回 null。
异常
无
示例 1
以下示例演示了如何使用 Java HttpURLConnection getErrorStream() 方法处理使用 https 协议的无效 url。在此示例中,我们正在创建 URL 类的实例。使用 url.openConnection() 方法,我们获取 HttpURLConnection 实例。使用 getErrorStream(),我们检查服务器是否返回错误响应并打印出来。
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://tutorialspoint.com/index1.htm?language=en#j2se"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setConnectTimeout(1000); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getErrorStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getErrorStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Error Stream is null"); } } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行以上程序,这将产生以下结果:
输出
Connected. Error Stream is null
示例 2
以下示例演示了如何使用 Java HttpURLConnection getErrorStream() 方法处理使用 http 协议的无效 url。在此示例中,我们正在创建 URL 类的实例。使用 url.openConnection() 方法,我们获取 HttpURLConnection 实例。使用 getErrorStream(),我们检查服务器是否返回错误响应并打印出来。
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://tutorialspoint.com/index1.htm?language=en#j2se"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setConnectTimeout(1000); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getErrorStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getErrorStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Error Stream is null"); } } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行以上程序,这将产生以下结果:
输出
Connected. Error Stream is null
示例 3
以下示例演示了如何使用 Java HttpURLConnection getErrorStream() 方法处理使用 http 协议的无效 url。在此示例中,我们正在创建 URL 类的实例。使用 url.openConnection() 方法,我们获取 HttpURLConnection 实例。使用 getErrorStream(),我们检查服务器是否返回错误响应并打印出来。
package com.tutorialspoint; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class HttpUrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://www.google.com/index1.htm"); HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection(); urlConnection.setConnectTimeout(1000); urlConnection.connect(); System.out.println("Connected."); if(urlConnection.getErrorStream() != null) { BufferedReader in = new BufferedReader( new InputStreamReader(urlConnection.getErrorStream())); String content = ""; String current; while((current = in.readLine()) != null) { content += current; } System.out.println(content); }else { System.out.println("Error Stream is null"); } } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行以上程序,这将产生以下结果:
输出
Connected. Error Stream is null