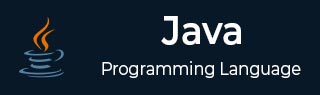
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络
- Java - 网络
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 存档
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 紧凑数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - 进程 API 改进
- Java - 流 API 改进
- Java - 增强的 @Deprecated 注解
- Java - CompletableFuture API 改进
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - throw 关键字
异常(或异常事件)是在程序执行期间出现的错误。当出现异常时,程序的正常流程被打断,程序/应用程序异常终止,这是不建议的,因此,这些异常需要处理。
异常可能由于许多不同的原因发生。以下是发生异常的一些场景。
用户输入了无效数据。
需要打开的文件找不到。
网络连接在通信过程中断开,或者 JVM 内存不足。
其中一些异常是由用户错误引起的,另一些是由程序员错误引起的,还有一些是由以某种方式发生故障的物理资源引起的。
Throws/Throw 关键字
如果方法不处理已检查异常,则该方法必须使用throws关键字声明它。throws 关键字出现在方法签名末尾。
您可以使用throw关键字抛出异常,无论是新实例化的异常还是刚刚捕获的异常。
尝试理解 throws 和 throw 关键字之间的区别,throws用于推迟已检查异常的处理,throw用于显式调用异常。
以下方法声明它抛出 RemoteException -
语法
public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } }
示例
在此示例中,我们调用了一个名为 accessElement() 的方法,该方法声明抛出 CustomException。因此,我们需要在 accessElement() 方法上编写一个 try-catch 块。当程序运行时,它会抛出异常并打印出来。
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) { try { accesElement(); } catch (CustomException e) { e.printStackTrace(); } System.out.println("Out of the block"); } public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } } } class CustomException extends Exception{ private static final long serialVersionUID = 1L; CustomException(Exception e){ super(e); } }
输出
com.tutorialspoint.CustomException: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:20) at com.tutorialspoint.ExcepTest.main(ExcepTest.java:8) Caused by: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:18) ... 1 more Out of the block
以下是另一个展示 throws 关键字用法的示例。
示例
在此示例中,我们调用了一个名为 accessElement() 的方法,该方法声明抛出 CustomException。因此,我们没有在 accessElement() 方法上编写 try-catch 块,而是 main 方法声明抛出异常。当程序运行时,它会抛出异常并打印出来。
// File Name : ExcepTest.java package com.tutorialspoint; public class ExcepTest { public static void main(String args[]) throws CustomException { accesElement(); System.out.println("Out of the block"); } public static void accesElement() throws CustomException { try { int a[] = new int[2]; System.out.println("Access element three :" + a[3]); } catch(Exception e) { throw new CustomException(e); } } } class CustomException extends Exception{ private static final long serialVersionUID = 1L; CustomException(Exception e){ super(e); } }
输出
Exception in thread "main" com.tutorialspoint.CustomException: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:16) at com.tutorialspoint.ExcepTest.main(ExcepTest.java:7) Caused by: java.lang.ArrayIndexOutOfBoundsException: 3 at com.tutorialspoint.ExcepTest.accesElement(ExcepTest.java:14) ... 1 more
由于异常未被处理,因此在此示例中最后一个语句未执行。
一个方法可以声明它抛出多个异常,在这种情况下,这些异常以逗号分隔的列表的形式声明。例如,以下方法声明它抛出 RemoteException 和 InsufficientFundsException -
示例
import java.io.*; public class className { public void withdraw(double amount) throws RemoteException, InsufficientFundsException { // Method implementation } // Remainder of class definition }