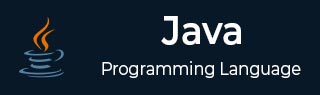
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境设置
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期与时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - if-else
- Java - switch
- Java - for循环
- Java - for-each循环
- Java - while循环
- Java - do-while循环
- Java - break
- Java - continue
面向对象编程
- Java - OOPs概念
- Java - 对象与类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 方法覆盖
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误与异常
- Java - 异常
- Java - try-catch块
- Java - try-with-resources
- Java - 多重catch块
- Java - 嵌套try块
- Java - finally块
- Java - throw异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程连接
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络编程
- Java - 网络编程
- Java - Socket编程
- Java - URL处理
- Java - URL类
- Java - URLConnection类
- Java - HttpURLConnection类
- Java - Socket类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级Java
- Java - 命令行参数
- Java - Lambda表达式
- Java - 发送邮件
- Java - Applet基础
- Java - Javadoc注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本Jar文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64编码解码
- Java - switch表达式
- Java - Teeing收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态CDS存档
- Java - Z垃圾收集器(ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 简洁的数字格式化
- Java - 垃圾回收
- Java - JIT编译器
Java 其他
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API改进
- Java - Stream API改进
- Java - 增强的@Deprecated注解
- Java - CompletableFuture API改进
- Java - 流
- Java - 日期时间API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java APIs与框架
Java 类引用
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - transient关键字
在Java中,序列化是一个概念,我们可以使用它将对象的状态写入字节流中,以便我们可以通过网络传输它(使用JPA和RMI等技术)。
序列化类的对象时,如果希望JVM忽略特定的实例变量,可以将其声明为transient。
语法
public transient int limit = 55; // will not persist public int b; // will persist
示例
在下面的Java程序中,Student类有两个实例变量name和age,其中age被声明为transient。在名为ExampleSerialize的另一个类中,我们尝试序列化和反序列化Student对象并显示其实例变量。由于age被设置为不可见(transient),因此只显示name的值。
package com.tutorialspoint; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; class Student implements Serializable{ private static final long serialVersionUID = 1L; private String name; private transient int age; public Student(String name, int age){ this.name = name; this.age = age; } public String getName() { return this.name; } public void setName(String name) { this.name = name; } public void setAge(int age) { this.age = age; } public int getAge() { return this.age; } } public class ExampleSerialize{ public static void main(String args[]) throws Exception{ Student std1 = new Student("Krishna", 30); FileOutputStream fos = null; ObjectOutputStream oos = null; FileInputStream fis = null; ObjectInputStream ois = null; try { fos = new FileOutputStream("/tmp/student.ser"); oos = new ObjectOutputStream(fos); oos.writeObject(std1); fis = new FileInputStream("/tmp/student.ser"); ois = new ObjectInputStream(fis); Student std2 = (Student) ois.readObject(); System.out.println(std2.getName()); } catch(IOException e) { oos.close(); fos.close(); ois.close(); fis.close(); } } }
输出
Krishna
示例
在下面的Java程序中,我们创建、序列化和反序列化一个employee对象。
员工
package com.tutorialspoint; public class Employee implements java.io.Serializable { public String name; public String address; public transient int SSN; public int number; public void mailCheck() { System.out.println("Mailing a check to " + name + " " + address); } }
SerializeDemo
package com.tutorialspoint; import java.io.*; public class SerializeDemo { public static void main(String [] args) { Employee e = new Employee(); e.name = "Reyan Ali"; e.address = "Phokka Kuan, Ambehta Peer"; e.SSN = 11122333; e.number = 101; try { FileOutputStream fileOut = new FileOutputStream("/tmp/employee.ser"); ObjectOutputStream out = new ObjectOutputStream(fileOut); out.writeObject(e); out.close(); fileOut.close(); System.out.printf("Serialized data is saved in /tmp/employee.ser"); } catch (IOException i) { i.printStackTrace(); } } }
下面的SerializeDemo程序实例化一个Employee对象并将其序列化到文件中。程序执行完毕后,将创建一个名为employee.ser的文件。
DeserializeDemo
package com.tutorialspoint; import java.io.*; public class DeserializeDemo { public static void main(String [] args) { Employee e = null; try { FileInputStream fileIn = new FileInputStream("/tmp/employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); e = (Employee) in.readObject(); in.close(); fileIn.close(); } catch (IOException i) { i.printStackTrace(); return; } catch (ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Name: " + e.name); System.out.println("Address: " + e.address); System.out.println("SSN: " + e.SSN); System.out.println("Number: " + e.number); } }
输出
Deserialized Employee... Name: Reyan Ali Address:Phokka Kuan, Ambehta Peer SSN: 0 Number:101
以下是需要注意的重要几点:
try/catch块尝试捕获ClassNotFoundException,这是readObject()方法声明的。为了使JVM能够反序列化对象,它必须能够找到该类的字节码。如果JVM在对象的反序列化过程中找不到类,则会抛出ClassNotFoundException。
请注意,readObject()的返回值被强制转换为Employee引用。
当对象被序列化时,SSN字段的值为11122333,但由于该字段是transient,因此此值未发送到输出流。反序列化的Employee对象的SSN字段为0。
java_basic_syntax.htm
广告