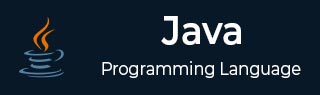
Java 教程
- Java - 首页
- Java - 概述
- Java - 历史
- Java - 特性
- Java 与 C++
- JVM - Java 虚拟机
- Java - JDK 与 JRE 与 JVM
- Java - Hello World 程序
- Java - 环境搭建
- Java - 基本语法
- Java - 变量类型
- Java - 数据类型
- Java - 类型转换
- Java - Unicode 系统
- Java - 基本运算符
- Java - 注释
- Java - 用户输入
- Java - 日期和时间
Java 控制语句
- Java - 循环控制
- Java - 决策制定
- Java - If-else
- Java - Switch
- Java - For 循环
- Java - For-Each 循环
- Java - While 循环
- Java - do-while 循环
- Java - Break
- Java - Continue
面向对象编程
- Java - OOPs 概念
- Java - 对象和类
- Java - 类属性
- Java - 类方法
- Java - 方法
- Java - 变量作用域
- Java - 构造函数
- Java - 访问修饰符
- Java - 继承
- Java - 聚合
- Java - 多态
- Java - 重写
- Java - 方法重载
- Java - 动态绑定
- Java - 静态绑定
- Java - 实例初始化块
- Java - 抽象
- Java - 封装
- Java - 接口
- Java - 包
- Java - 内部类
- Java - 静态类
- Java - 匿名类
- Java - 单例类
- Java - 包装类
- Java - 枚举
- Java - 枚举构造函数
- Java - 枚举字符串
Java 内置类
Java 文件处理
Java 错误和异常
- Java - 异常
- Java - try-catch 块
- Java - try-with-resources
- Java - 多重 catch 块
- Java - 嵌套 try 块
- Java - Finally 块
- Java - throw 异常
- Java - 异常传播
- Java - 内置异常
- Java - 自定义异常
Java 多线程
- Java - 多线程
- Java - 线程生命周期
- Java - 创建线程
- Java - 启动线程
- Java - 线程合并
- Java - 线程命名
- Java - 线程调度器
- Java - 线程池
- Java - 主线程
- Java - 线程优先级
- Java - 守护线程
- Java - 线程组
- Java - 关闭钩子
Java 同步
Java 网络
- Java - 网络
- Java - 套接字编程
- Java - URL 处理
- Java - URL 类
- Java - URLConnection 类
- Java - HttpURLConnection 类
- Java - Socket 类
- Java - 泛型
Java 集合
Java 接口
Java 数据结构
Java 集合算法
高级 Java
- Java - 命令行参数
- Java - Lambda 表达式
- Java - 发送电子邮件
- Java - Applet 基础
- Java - Javadoc 注释
- Java - 自动装箱和拆箱
- Java - 文件不匹配方法
- Java - REPL (JShell)
- Java - 多版本 Jar 文件
- Java - 私有接口方法
- Java - 内部类菱形运算符
- Java - 多分辨率图像 API
- Java - 集合工厂方法
- Java - 模块系统
- Java - Nashorn JavaScript
- Java - Optional 类
- Java - 方法引用
- Java - 函数式接口
- Java - 默认方法
- Java - Base64 编码解码
- Java - Switch 表达式
- Java - Teeing 收集器
- Java - 微基准测试
- Java - 文本块
- Java - 动态 CDS 档案
- Java - Z 垃圾收集器 (ZGC)
- Java - 空指针异常
- Java - 打包工具
- Java - 密封类
- Java - 记录类
- Java - 隐藏类
- Java - 模式匹配
- Java - 紧凑数字格式化
- Java - 垃圾回收
- Java - JIT 编译器
Java 杂项
- Java - 递归
- Java - 正则表达式
- Java - 序列化
- Java - 字符串
- Java - Process API 增强
- Java - Stream API 增强
- Java - 增强 @Deprecated 注解
- Java - CompletableFuture API 增强
- Java - 流
- Java - 日期时间 API
- Java 8 - 新特性
- Java 9 - 新特性
- Java 10 - 新特性
- Java 11 - 新特性
- Java 12 - 新特性
- Java 13 - 新特性
- Java 14 - 新特性
- Java 15 - 新特性
- Java 16 - 新特性
Java API 和框架
Java 类参考
- Java - Scanner
- Java - 数组
- Java - 字符串
- Java - Date
- Java - ArrayList
- Java - Vector
- Java - Stack
- Java - PriorityQueue
- Java - LinkedList
- Java - ArrayDeque
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - TreeMap
- Java - IdentityHashMap
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - TreeSet
- Java - BitSet
- Java - Dictionary
- Java - Hashtable
- Java - Properties
- Java - Collection
- Java - Array
Java 有用资源
Java - URLConnection getReadTimeout() 方法
描述
Java URLConnection getReadTimeout() 方法返回读取超时设置。返回值为 0 表示该选项已禁用(即超时时间为无限大)。
声明
以下是 java.net.URLConnection.getReadTimeout() 方法的声明
public int getReadTimeout()
参数
无
返回值
一个 int 值,表示读取超时值(以毫秒为单位)。
异常
无
示例 1
以下示例演示了如何使用 Java URLConnection getReadTimeout() 方法获取使用 https 协议的有效 url 的读取超时时间。在本例中,我们创建了一个 URL 类的实例。使用 url.openConnection() 方法,我们获得了 URLConnection 实例。使用 getReadTimeout(),我们获取了 URLConnection 实例的读取超时时间并打印出来。
package com.tutorialspoint; import java.io.IOException; import java.net.URL; import java.net.URLConnection; import java.time.Instant; import java.util.Date; public class UrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://tutorialspoint.com"); URLConnection urlConnection = url.openConnection(); long headerValue = urlConnection.getReadTimeout(); System.out.println("Read Timeout: " + Date.from(Instant.ofEpochMilli(headerValue))); } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行上述程序,这将产生以下结果:
输出
Read Timeout: Thu Jan 01 05:30:00 IST 1970
示例 2
以下示例演示了如何使用 Java URLConnection getReadTimeout() 方法获取使用 http 协议的有效 url 的读取超时时间。在本例中,我们创建了一个 URL 类的实例。使用 url.openConnection() 方法,我们获得了 URLConnection 实例。使用 getReadTimeout(),我们获取了 URLConnection 实例的读取超时时间并打印出来。
package com.tutorialspoint; import java.io.IOException; import java.net.URL; import java.net.URLConnection; import java.time.Instant; import java.util.Date; public class UrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("https://tutorialspoint.com"); URLConnection urlConnection = url.openConnection(); long headerValue = urlConnection.getReadTimeout(); System.out.println("Read Timeout: " + Date.from(Instant.ofEpochMilli(headerValue))); } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行上述程序,这将产生以下结果:
输出
Read Timeout: Thu Jan 01 05:30:00 IST 1970
示例 3
以下示例演示了如何使用 Java URLConnection getReadTimeout() 方法获取使用 http 协议的有效 url 的读取超时时间。在本例中,我们创建了一个 URL 类的实例。使用 url.openConnection() 方法,我们获得了 URLConnection 实例。使用 getReadTimeout(),我们获取了 URLConnection 实例的读取超时时间并打印出来。
package com.tutorialspoint; import java.io.IOException; import java.net.URL; import java.net.URLConnection; import java.time.Instant; import java.util.Date; public class UrlConnectionDemo { public static void main(String [] args) { try { URL url = new URL("http://www.google.com"); URLConnection urlConnection = url.openConnection(); long headerValue = urlConnection.getReadTimeout(); System.out.println("Read Timeout: " + Date.from(Instant.ofEpochMilli(headerValue))); } catch (IOException e) { e.printStackTrace(); } } }
让我们编译并运行上述程序,这将产生以下结果:
输出
Read Timeout: Thu Jan 01 05:30:00 IST 1970