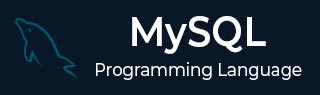
- MySQL 基础
- MySQL - 首页
- MySQL - 简介
- MySQL - 特性
- MySQL - 版本
- MySQL - 变量
- MySQL - 安装
- MySQL - 管理
- MySQL - PHP 语法
- MySQL - Node.js 语法
- MySQL - Java 语法
- MySQL - Python 语法
- MySQL - 连接
- MySQL - Workbench
- MySQL 数据库
- MySQL - 创建数据库
- MySQL - 删除数据库
- MySQL - 选择数据库
- MySQL - 显示数据库
- MySQL - 复制数据库
- MySQL - 数据库导出
- MySQL - 数据库导入
- MySQL - 数据库信息
- MySQL 用户
- MySQL - 创建用户
- MySQL - 删除用户
- MySQL - 显示用户
- MySQL - 修改密码
- MySQL - 授权
- MySQL - 显示权限
- MySQL - 收回权限
- MySQL - 锁定用户帐户
- MySQL - 解锁用户帐户
- MySQL 表
- MySQL - 创建表
- MySQL - 显示表
- MySQL - 修改表
- MySQL - 重命名表
- MySQL - 克隆表
- MySQL - 清空表
- MySQL - 临时表
- MySQL - 修复表
- MySQL - 描述表
- MySQL - 添加/删除列
- MySQL - 显示列
- MySQL - 重命名列
- MySQL - 表锁定
- MySQL - 删除表
- MySQL - 派生表
- MySQL 查询
- MySQL - 查询
- MySQL - 约束
- MySQL - INSERT 查询
- MySQL - SELECT 查询
- MySQL - UPDATE 查询
- MySQL - DELETE 查询
- MySQL - REPLACE 查询
- MySQL - INSERT IGNORE
- MySQL - INSERT ON DUPLICATE KEY UPDATE
- MySQL - INSERT INTO SELECT
- MySQL 运算符和子句
- MySQL - WHERE 子句
- MySQL - LIMIT 子句
- MySQL - DISTINCT 子句
- MySQL - ORDER BY 子句
- MySQL - GROUP BY 子句
- MySQL - HAVING 子句
- MySQL - AND 运算符
- MySQL - OR 运算符
- MySQL - LIKE 运算符
- MySQL - IN 运算符
- MySQL - ANY 运算符
- MySQL - EXISTS 运算符
- MySQL - NOT 运算符
- MySQL - 不等于运算符
- MySQL - IS NULL 运算符
- MySQL - IS NOT NULL 运算符
- MySQL - BETWEEN 运算符
- MySQL - UNION 运算符
- MySQL - UNION vs UNION ALL
- MySQL - MINUS 运算符
- MySQL - INTERSECT 运算符
- MySQL - INTERVAL 运算符
- MySQL 连接
- MySQL - 使用连接
- MySQL - INNER JOIN
- MySQL - LEFT JOIN
- MySQL - RIGHT JOIN
- MySQL - CROSS JOIN
- MySQL - FULL JOIN
- MySQL - 自连接
- MySQL - 删除连接
- MySQL - UPDATE 连接
- MySQL - UNION vs JOIN
- MySQL 触发器
- MySQL - 触发器
- MySQL - 创建触发器
- MySQL - 显示触发器
- MySQL - 删除触发器
- MySQL - BEFORE INSERT 触发器
- MySQL - AFTER INSERT 触发器
- MySQL - BEFORE UPDATE 触发器
- MySQL - AFTER UPDATE 触发器
- MySQL - BEFORE DELETE 触发器
- MySQL - AFTER DELETE 触发器
- MySQL 数据类型
- MySQL - 数据类型
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL 正则表达式
- MySQL - 正则表达式
- MySQL - RLIKE 运算符
- MySQL - NOT LIKE 运算符
- MySQL - NOT REGEXP 运算符
- MySQL - regexp_instr() 函数
- MySQL - regexp_like() 函数
- MySQL - regexp_replace() 函数
- MySQL - regexp_substr() 函数
- MySQL 函数 & 运算符
- MySQL - 日期和时间函数
- MySQL - 算术运算符
- MySQL - 数字函数
- MySQL - 字符串函数
- MySQL - 聚合函数
- MySQL 其他概念
- MySQL - NULL 值
- MySQL - 事务
- MySQL - 使用序列
- MySQL - 处理重复项
- MySQL - SQL 注入
- MySQL - 子查询
- MySQL - 注释
- MySQL - CHECK 约束
- MySQL - 存储引擎
- MySQL - 将表导出到 CSV 文件
- MySQL - 将 CSV 文件导入数据库
- MySQL - UUID
- MySQL - 公共表表达式
- MySQL - ON DELETE CASCADE
- MySQL - Upsert
- MySQL - 水平分区
- MySQL - 垂直分区
- MySQL - 游标
- MySQL - 存储函数
- MySQL - SIGNAL
- MySQL - RESIGNAL
- MySQL - 字符集
- MySQL - 排序规则
- MySQL - 通配符
- MySQL - 别名
- MySQL - ROLLUP
- MySQL - 今日日期
- MySQL - 字面量
- MySQL - 存储过程
- MySQL - EXPLAIN
- MySQL - JSON
- MySQL - 标准差
- MySQL - 查找重复记录
- MySQL - 删除重复记录
- MySQL - 选择随机记录
- MySQL - SHOW PROCESSLIST
- MySQL - 更改列类型
- MySQL - 重置自动递增
- MySQL - Coalesce() 函数
- MySQL 有用资源
- MySQL - 有用函数
- MySQL - 语句参考
- MySQL - 快速指南
- MySQL - 有用资源
- MySQL - 讨论
MySQL - 删除连接
MySQL 中简单的删除操作可以对表中的单个实体或多个实体执行。但是,如果此删除操作需要对多个表的多个实体执行怎么办?这就是连接发挥作用的地方。
MySQL DELETE... JOIN
正如我们之前在本教程中讨论的那样,连接用于通过组合这些表的列(基于公共字段)来检索来自两个或多个表的记录。此合并的数据可以被删除,所有更改都反映在原始表中。
语法
以下是 MySQL 中 DELETE... JOIN 语句的基本语法:
DELETE table(s) FROM table1 JOIN table2 ON table1.common_field = table2.common_field;
在执行删除操作时,可以使用任何连接子句(INNER JOIN、LEFT JOIN、RIGHT JOIN 等)。
示例
在此示例中,我们首先创建一个名为 CUSTOMERS 的表,其中包含客户的个人详细信息,包括他们的姓名、年龄、地址和薪水等。
CREATE TABLE CUSTOMERS ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, ADDRESS CHAR (25), SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
现在,使用 INSERT 语句按如下方式向此表插入值:
INSERT INTO CUSTOMERS VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00), (2, 'Khilan', 25, 'Delhi', 1500.00), (3, 'Kaushik', 23, 'Kota', 2000.00), (4, 'Chaitali', 25, 'Mumbai', 6500.00), (5, 'Hardik', 27, 'Bhopal', 8500.00), (6, 'Komal', 22, 'Hyderabad', 4500.00), (7, 'Muffy', 24, 'Indore', 10000.00);
表将被创建为:
ID | 姓名 | 年龄 | 地址 | 薪水 |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
4 | Chaitali | 25 | Mumbai | 6500.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | 4500.00 |
7 | Muffy | 24 | Indore | 10000.00 |
让我们创建另一个名为 ORDERS 的表,其中包含已下订单的详细信息以及下单日期。
CREATE TABLE ORDERS ( OID INT NOT NULL, DATE VARCHAR (20) NOT NULL, CUSTOMER_ID INT NOT NULL, AMOUNT DECIMAL (18, 2), );
使用 INSERT 语句,按如下方式向此表插入值:
INSERT INTO ORDERS VALUES (102, '2009-10-08 00:00:00', 3, 3000.00), (100, '2009-10-08 00:00:00', 3, 1500.00), (101, '2009-11-20 00:00:00', 2, 1560.00), (103, '2008-05-20 00:00:00', 4, 2060.00);
表显示如下:
OID | 日期 | 客户ID | 金额 |
---|---|---|---|
102 | 2009-10-08 00:00:00 | 3 | 3000.00 |
100 | 2009-10-08 00:00:00 | 3 | 1500.00 |
101 | 2009-11-20 00:00:00 | 2 | 1560.00 |
103 | 2008-05-20 00:00:00 | 4 | 2060.00 |
删除操作是通过对这些表应用DELETE... JOIN查询来执行的。
DELETE a FROM CUSTOMERS AS a INNER JOIN ORDERS AS b ON a.ID = b.CUSTOMER_ID;
验证
要验证更改是否反映在表中,可以使用 SELECT 语句打印表。
ID | 姓名 | 年龄 | 地址 | 薪水 |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | 4500.00 |
7 | Muffy | 24 | Indore | 10000.00 |
带有 WHERE 子句的 DELETE... JOIN
DELETE... JOIN 查询中的ON子句用于对记录应用约束。此外,我们还可以使用 WHERE 子句使过滤更严格。观察下面的查询;在这里,我们尝试删除 CUSTOMERS 表中薪水低于 2000.00 卢比的客户记录。
DELETE a FROM CUSTOMERS AS a INNER JOIN ORDERS AS b ON a.ID = b.CUSTOMER_ID WHERE a.SALARY < 2000.00;
验证
为了验证更改是否反映在原始表中,我们将使用 SELECT 语句。
删除后,CUSTOMERS 表如下所示:
ID | 姓名 | 年龄 | 地址 | 薪水 |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
4 | Chaitali | 25 | Mumbai | 6500.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | 4500.00 |
7 | Muffy | 24 | Indore | 10000.00 |
使用客户端程序进行删除连接
除了使用 MySQL 查询连接两个或多个表之外,我们还可以使用客户端程序执行 Delete Join 操作。
语法
要通过 PHP 程序执行 Delete Join,我们需要使用mysqli函数query()执行带有 JOIN 子句的 DELETE 语句,如下所示:
$sql = 'DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author'; $mysqli->query($sql);
要通过 JavaScript 程序执行 Delete Join,我们需要使用mysql2库的query()函数执行带有 JOIN 子句的 DELETE 语句,如下所示:
sql = "DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author"; con.query(sql);
要通过 Java 程序执行 Delete Join,我们需要使用JDBC函数executeUpdate()执行带有 JOIN 子句的 DELETE 语句,如下所示:
String sql = "DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author"; statement.executeUpdate(sql);
要通过 python 程序执行 Delete Join,我们需要使用MySQL Connector/Python的execute()函数执行带有 JOIN 子句的 DELETE 语句,如下所示:
delete_join_query = "DELETE a FROM CUSTOMERS AS a INNER JOIN ORDERS AS b ON a.ID = b.CUST_ID" cursorObj.execute(delete_join_query)
示例
以下是程序:
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $dbname = 'TUTORIALS'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname); if ($mysqli->connect_errno) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } // printf('Connected successfully.
'); $sql = 'DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author'; if ($mysqli->query($sql)) { printf("Join deleted successfully!.
"); } if ($mysqli->errno) { printf("Join could not be deleted !.
", $mysqli->error); } $mysqli->close();
输出
获得的输出如下:
Join deleted successfully!.
var mysql = require("mysql2"); var con = mysql.createConnection({ host: "localhost", user: "root", password: "password", }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; // console.log("Connected successfully...!"); // console.log("--------------------------"); sql = "USE TUTORIALS"; con.query(sql); //Delete Join sql = "DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author"; con.query(sql, function (err, result) { if (err) throw err; console.log(result); }); });
输出
产生的输出如下所示:
ResultSetHeader { fieldCount: 0, affectedRows: 2, insertId: 0, info: '', serverStatus: 34, warningStatus: 0, changedRows: 0 }
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class DeleteJoin { public static void main(String[] args) { String url = "jdbc:mysql://127.0.0.1:3306/TUTORIALS"; String username = "root"; String password = "password"; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection connection = DriverManager.getConnection(url, username, password); Statement statement = connection.createStatement(); System.out.println("Connected successfully...!"); //MySQL Delete JOIN...!; String sql = "DELETE tutorials_tbl, tcount_tbl FROM tcount_tbl INNER JOIN tutorials_tbl ON tcount_tbl.tutorial_author = tutorials_tbl.tutorial_author"; statement.executeUpdate(sql); System.out.println("JOIN Deleted successfully...!"); connection.close(); } catch (Exception e) { System.out.println(e); } } }
输出
获得的输出如下所示:
Connected successfully...! JOIN Deleted successfully...!
import mysql.connector #establishing the connection connection = mysql.connector.connect( host='localhost', user='root', password='password', database='tut' ) cursorObj = connection.cursor() delete_join_query = f"""DELETE a FROM CUSTOMERS AS a INNER JOIN ORDERS AS b ON a.ID = b.CUST_ID""" cursorObj.execute(delete_join_query) connection.commit() print("deleted succesfully") cursorObj.close() connection.close()
输出
以下是上述代码的输出:
deleted successfully