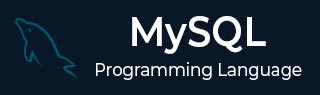
- MySQL 基础
- MySQL - 首页
- MySQL - 简介
- MySQL - 特性
- MySQL - 版本
- MySQL - 变量
- MySQL - 安装
- MySQL - 管理
- MySQL - PHP 语法
- MySQL - Node.js 语法
- MySQL - Java 语法
- MySQL - Python 语法
- MySQL - 连接
- MySQL - Workbench
- MySQL 数据库
- MySQL - 创建数据库
- MySQL - 删除数据库
- MySQL - 选择数据库
- MySQL - 显示数据库
- MySQL - 复制数据库
- MySQL - 数据库导出
- MySQL - 数据库导入
- MySQL - 数据库信息
- MySQL 用户
- MySQL - 创建用户
- MySQL - 删除用户
- MySQL - 显示用户
- MySQL - 修改密码
- MySQL - 授予权限
- MySQL - 显示权限
- MySQL - 收回权限
- MySQL - 锁定用户账户
- MySQL - 解锁用户账户
- MySQL 表
- MySQL - 创建表
- MySQL - 显示表
- MySQL - 修改表
- MySQL - 重命名表
- MySQL - 克隆表
- MySQL - 清空表
- MySQL - 临时表
- MySQL - 修复表
- MySQL - 描述表
- MySQL - 添加/删除列
- MySQL - 显示列
- MySQL - 重命名列
- MySQL - 表锁定
- MySQL - 删除表
- MySQL - 派生表
- MySQL 查询
- MySQL - 查询
- MySQL - 约束
- MySQL - 插入查询
- MySQL - 选择查询
- MySQL - 更新查询
- MySQL - 删除查询
- MySQL - 替换查询
- MySQL - 插入忽略
- MySQL - 插入重复键更新
- MySQL - 插入到选择
- MySQL 运算符和子句
- MySQL - Where 子句
- MySQL - Limit 子句
- MySQL - Distinct 子句
- MySQL - Order By 子句
- MySQL - Group By 子句
- MySQL - Having 子句
- MySQL - AND 运算符
- MySQL - OR 运算符
- MySQL - Like 运算符
- MySQL - IN 运算符
- MySQL - ANY 运算符
- MySQL - EXISTS 运算符
- MySQL - NOT 运算符
- MySQL - 不等于运算符
- MySQL - IS NULL 运算符
- MySQL - IS NOT NULL 运算符
- MySQL - Between 运算符
- MySQL - UNION 运算符
- MySQL - UNION 与 UNION ALL
- MySQL - MINUS 运算符
- MySQL - INTERSECT 运算符
- MySQL - INTERVAL 运算符
- MySQL 连接
- MySQL - 使用连接
- MySQL - 内连接
- MySQL - 左连接
- MySQL - 右连接
- MySQL - 交叉连接
- MySQL - 全连接
- MySQL - 自连接
- MySQL - 删除连接
- MySQL - 更新连接
- MySQL - Union 与 Join
- MySQL 触发器
- MySQL - 触发器
- MySQL - 创建触发器
- MySQL - 显示触发器
- MySQL - 删除触发器
- MySQL - 插入前触发器
- MySQL - 插入后触发器
- MySQL - 更新前触发器
- MySQL - 更新后触发器
- MySQL - 删除前触发器
- MySQL - 删除后触发器
- MySQL 数据类型
- MySQL - 数据类型
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL 正则表达式
- MySQL - 正则表达式
- MySQL - RLIKE 运算符
- MySQL - NOT LIKE 运算符
- MySQL - NOT REGEXP 运算符
- MySQL - regexp_instr() 函数
- MySQL - regexp_like() 函数
- MySQL - regexp_replace() 函数
- MySQL - regexp_substr() 函数
- MySQL 函数 & 运算符
- MySQL - 日期和时间函数
- MySQL - 算术运算符
- MySQL - 数值函数
- MySQL - 字符串函数
- MySQL - 聚合函数
- MySQL 杂项概念
- MySQL - NULL 值
- MySQL - 事务
- MySQL - 使用序列
- MySQL - 处理重复项
- MySQL - SQL 注入
- MySQL - 子查询
- MySQL - 注释
- MySQL - 检查约束
- MySQL - 存储引擎
- MySQL - 将表导出到 CSV 文件
- MySQL - 将 CSV 文件导入数据库
- MySQL - UUID
- MySQL - 公共表表达式
- MySQL - ON DELETE CASCADE
- MySQL - Upsert
- MySQL - 水平分区
- MySQL - 垂直分区
- MySQL - 游标
- MySQL - 存储函数
- MySQL - Signal
- MySQL - Resignal
- MySQL - 字符集
- MySQL - 校对
- MySQL - 通配符
- MySQL - 别名
- MySQL - ROLLUP
- MySQL - 当天日期
- MySQL - 字面量
- MySQL - 存储过程
- MySQL - Explain
- MySQL - JSON
- MySQL - 标准差
- MySQL - 查找重复记录
- MySQL - 删除重复记录
- MySQL - 选择随机记录
- MySQL - 显示 Processlist
- MySQL - 更改列类型
- MySQL - 重置自动递增
- MySQL - Coalesce() 函数
- MySQL 有用资源
- MySQL - 有用函数
- MySQL - 语句参考
- MySQL - 快速指南
- MySQL - 有用资源
- MySQL - 讨论
MySQL - 临时表
什么是临时表?
临时表是在数据库中创建的用于临时存储数据的表。这些表将在当前客户端会话终止或结束时自动删除。此外,如果用户决定手动删除它们,则可以显式删除这些表。您可以对临时表执行各种 SQL 操作,就像对永久表一样,包括 CREATE、UPDATE、DELETE、INSERT、JOIN 等。
临时表在 MySQL 3.23 版本中引入。如果您使用的是 3.23 之前的旧版 MySQL,则无法使用临时表,而是可以使用堆表。
如前所述,临时表仅在会话处于活动状态时才会存在。如果您在 PHP 脚本中运行代码,则脚本执行完成后,临时表将自动销毁。如果您通过 MySQL 客户端程序连接到 MySQL 数据库服务器,则临时表将一直存在,直到您关闭客户端或手动销毁该表。
在 MySQL 中创建临时表
在 MySQL 中创建临时表与创建常规数据库表非常相似。但是,我们不使用 CREATE TABLE,而是使用 CREATE TEMPORARY TABLE 语句。
语法
以下是 MySQL 中创建临时表的语法:
CREATE TEMPORARY TABLE table_name( column1 datatype, column2 datatype, column3 datatype, ..... columnN datatype, PRIMARY KEY( one or more columns ) );
示例
首先,让我们使用以下查询创建一个名为CUSTOMERS的临时表:
CREATE TEMPORARY TABLE CUSTOMERS( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, ADDRESS CHAR (25) , SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
与普通表类似,我们可以使用 INSERT 语句将记录插入临时表。在这里,我们将三条记录插入到上面创建的临时表中:
INSERT INTO CUSTOMERS VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00 ), (2, 'Khilan', 25, 'Delhi', 1500.00 ), (3, 'kaushik', 23, 'Kota', 2000.00 );
执行以下查询以显示临时表 CUSTOMERS 的所有记录。
SELECT * FROM CUSTOMERS;
以下是 CUSTOMERS 表的记录:
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
当我们发出 SHOW TABLES 命令时,我们的临时表不会显示在表列表中。要验证临时表是否存在,我们需要使用SELECT语句检索其数据。临时表将在我们结束会话时删除,因此如果我们注销 MySQL 然后尝试发出 SELECT 命令,我们将找不到数据库中的临时表。
在 MySQL 中删除临时表
尽管 MySQL 在数据库连接结束时会自动删除临时表,但如果需要,我们仍然可以使用 DROP TEMPORARY TABLE 命令自己删除它们。
语法
以下是 MySQL 中删除临时表的语法:
DROP TEMPORARY TABLE table_name;
示例
在以下查询中,我们删除了在前面示例中创建的临时表 CUSTOMERS:
DROP TEMPORARY TABLE CUSTOMERS;
输出
执行以上查询将产生以下输出:
Query OK, 0 rows affected (0.00 sec)
验证
现在,让我们使用以下查询验证临时表 CUSTOMERS,检索其记录:
SELECT * FROM CUSTOMERS;
由于我们删除了临时表 CUSTOMERS,它将生成一条错误消息,指出表不存在。
ERROR 1146: Table 'TUTORIALS.CUSTOMERS' doesn't exist
使用客户端程序创建临时表
除了使用 MySQL 查询在 MySQL 数据库中创建临时表之外,我们还可以使用客户端程序对表执行“TEMPORARY TABLE”操作。
语法
以下是各种编程语言中在 MySQL 数据库中创建临时表的语法:
要通过 PHP 程序在 MySQL 数据库中创建临时表,我们需要使用mysqli函数query()执行创建临时表语句,如下所示:
$sql="CREATE temporary Table table_name(column_name, column_type, ...)"; $mysqli->query($sql);
要通过 Node.js 程序在 MySQL 数据库中创建临时表,我们需要使用mysql2库的query()函数执行创建临时表语句,如下所示:
sql="CREATE temporary Table table_name(column_name, column_type, ...)"; con.query(sql);
要通过 Java 程序在 MySQL 数据库中创建临时表,我们需要使用JDBC函数executeUpdate()执行创建临时表语句,如下所示:
String sql="CREATE temporary Table table_name(column_name, column_type, ...)"; statement.executeQuery(sql);
要通过 Python 程序在 MySQL 数据库中创建临时表,我们需要使用 MySQLConnector/Python的execute()函数执行创建临时表语句,如下所示:
sql="CREATE temporary Table table_name(column_name, column_type, ...)"; cursorObj.execute(sql);
示例
以下是程序:
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $dbname = 'TUTORIALS'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname); if ($mysqli->connect_errno) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } // printf('Connected successfully.
'); $sql = "CREATE TEMPORARY TABLE SalesSummary (" . " product_name VARCHAR(50) NOT NULL, " . " total_sales DECIMAL(12,2) NOT NULL DEFAULT 0.00, " . " avg_unit_price DECIMAL(7,2) NOT NULL DEFAULT 0.00, " . " total_units_sold INT UNSIGNED NOT NULL DEFAULT 0 )"; if ($mysqli->query($sql)) { printf("temporary table created successfully.
"); } if ($mysqli->errno) { printf("temporary table could not be created: %s
", $mysqli->error); } $mysqli->close();
输出
获得的输出如下:
temporary table created successfully.
var mysql = require('mysql2'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "Nr5a0204@123" }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; console.log("Connected!"); console.log("--------------------------"); //Creating a Database sql = "CREATE DATABASE testdb" con.query(sql); //Selecting a Database sql = "USE testdb" con.query(sql); //Creating table sql = "CREATE TEMPORARY TABLE SalesSummary (product_name VARCHAR(50) NOT NULL, total_sales DECIMAL(12,2) NOT NULL DEFAULT 0.00, avg_unit_price DECIMAL(7,2) NOT NULL DEFAULT 0.00, total_units_sold INT UNSIGNED NOT NULL DEFAULT 0);" con.query(sql); sql = "INSERT INTO SalesSummary(product_name, total_sales, avg_unit_price, total_units_sold)VALUES('cucumber', 100.25, 90, 2);" con.query(sql); sql = "SELECT * FROM SalesSummary;" con.query(sql, function(err, result){ if (err) throw err console.log("**SalesSummary Table:**") console.log(result); }); });
输出
产生的输出如下:
Connected! -------------------------- **SalesSummary Table:** [ { product_name: 'cucumber', total_sales: '100.25', avg_unit_price: '90.00', total_units_sold: 2 } ]
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class TemporaryTable { public static void main(String[] args){ String url = "jdbc:mysql://127.0.0.1:3306/TUTORIALS"; String username = "root"; String password = "password"; try{ Class.forName("com.mysql.cj.jdbc.Driver"); Connection connection = DriverManager.getConnection(url, username, password); Statement statement = connection.createStatement(); System.out.println("Connected successfully...!"); //Create temporary table...! String sql = "CREATE TEMPORARY TABLE SalesSummary (product_name VARCHAR(50) NOT NULL, total_sales DECIMAL(12,2) NOT NULL DEFAULT 0.00, avg_unit_price DECIMAL(7,2) NOT NULL DEFAULT 0.00, total_units_sold INT UNSIGNED NOT NULL DEFAULT 0 )"; statement.executeUpdate(sql); System.out.println("Temporary table created successfully...!"); ResultSet resultSet = statement.executeQuery("DESCRIBE SalesSummary"); while(resultSet.next()) { System.out.print(resultSet.getNString(1)); System.out.println(); } connection.close(); } catch(Exception e){ System.out.println(e); } } }
输出
获得的输出如下所示:
Connected successfully...! Temporary table created successfully...! product_name total_sales avg_unit_price total_units_sold
import mysql.connector #establishing the connection connection = mysql.connector.connect( host='localhost', user='root', password='password', database='tut' ) table_name = 'tutorials_tbl_temp' #Creating a cursor object cursorObj = connection.cursor() create_table_query = f"CREATE TEMPORARY TABLE {table_name} (NAME VARCHAR(50), ID INT)" cursorObj.execute(create_table_query) print(f"Temporary table '{table_name}' is created successfully.") cursorObj.close() connection.close()
输出
以下是上述代码的输出:
Temporary table 'tutorials_tbl_temp' is created successfully.