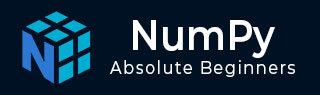
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境配置
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 数组的创建和操作
- NumPy - 数组创建函数
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 数组迭代
- NumPy - 数组重塑
- NumPy - 数组拼接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引与切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播机制
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 副本与视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- NumPy - 加载数组
- NumPy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 缺失数据插补
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- NumPy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
Python NumPy Broadcast() 类
NumPy 的 Broadcast() 类模拟广播机制,并返回一个封装了将一个数组对另一个数组进行广播的结果的对象。此类以数组作为输入参数。
此类允许对不同形状的数组进行运算,方法是虚拟地扩展较小的数组以匹配较大数组的形状,而无需实际在内存中创建更大的数组。
语法
numpy.broadcast() 类的语法如下:
numpy.broadcast(*array_like)
参数
NumPy Broadcast() 类以 *array_like 作为输入参数,它们是要相互广播的输入数组。
返回值
它返回一个 numpy.broadcast 对象,可用于迭代数组。
示例 1
以下是使用 NumPy Broadcast() 类创建广播数组的示例。此处,数组 a 和 b 被广播到公共形状 (3, 3) 并逐元素迭代:
import numpy as np scalar = 5 array_1d = np.array([1, 2, 3]) broadcast_obj = np.broadcast(scalar, array_1d) print("Broadcasted Shape:", broadcast_obj.shape) print("Broadcasted Elements:") for x, y in broadcast_obj: print(f"({x}, {y})")
输出
Broadcasted Shape: (3,) Broadcasted Elements: (5, 1) (5, 2) (5, 3)
示例 2
下面的示例显示了将一维数组 [1, 2, 3] 与二维数组 [[4], [5], [6]] 进行广播。一维数组将在二维数组的第二维上进行广播,并创建一个适合逐元素运算的形状:
import numpy as np array_1d = np.array([1, 2, 3]) array_2d = np.array([[4], [5], [6]]) broadcast_obj = np.broadcast(array_1d, array_2d) print("Broadcasted Shape:", broadcast_obj.shape) print("Broadcasted Elements:") for x, y in broadcast_obj: print(f"({x}, {y})")
输出
Broadcasted Shape: (3, 3) Broadcasted Elements: (1, 4) (2, 4) (3, 4) (1, 5) (2, 5) (3, 5) (1, 6) (2, 6) (3, 6)
示例 3
在此示例中,我们将 numpy.broadcast() 类与 NumPy 库的内置广播机制进行比较:
import numpy as np x = np.array([[1], [2], [3]]) y = np.array([4, 5, 6]) # Broadcasting x against y b = np.broadcast(x, y) # Using nditer to iterate over the broadcasted object print('Broadcast x against y:') for r, c in np.nditer([x, y]): print(r, c) print('\n') # Shape attribute returns the shape of the broadcast object print('The shape of the broadcast object:') print(b.shape) print('\n') # Adding x and y manually using broadcast b = np.broadcast(x, y) c = np.empty(b.shape) print('Add x and y manually using broadcast:') print(c.shape) print('\n') # Compute the addition manually c.flat = [u + v for (u, v) in np.nditer([x, y])] print('After applying the flat function:') print(c) print('\n') # Same result obtained by NumPy's built-in broadcasting support print('The summation of x and y:') print(x + y)
输出
The shape of the broadcast object: (3, 3) Add x and y manually using broadcast: (3, 3) After applying the flat function: [[5. 6. 7.] [6. 7. 8.] [7. 8. 9.]] The summation of x and y: [[5 6 7] [6 7 8] [7 8 9]]
numpy_array_manipulation.htm
广告