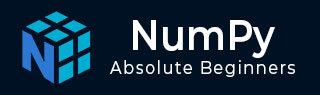
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境配置
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 数组的创建和操作
- NumPy - 数组创建函数
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 数组迭代
- NumPy - 数组重塑
- NumPy - 数组连接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引与切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组的轴
- NumPy - 字节交换
- NumPy - 复制与视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组的列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 估算缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - 沿轴排序
NumPy 中的沿轴排序
在 NumPy 中,数组可以是多维的,排序可以沿着这些维度(轴)中的任何一个进行。沿轴排序意味着根据该轴上的值按特定顺序排列数组的元素。
在 NumPy 中,不同的轴是:**第 0 轴**(行),**第 1 轴**(列),以及**更高阶轴**(深度或附加维度)。
np.sort() 函数
np.sort() 函数对数组的元素进行排序,并返回一个包含排序后元素的新数组。排序可以在指定的轴上进行,如果没有指定轴,则函数默认沿最后一个轴排序。以下是语法:
numpy.sort(a, axis=-1, kind=None, order=None)
其中,
- **a:** 要排序的数组。
- **axis:** 要排序的轴。默认为 -1,表示沿最后一个轴排序。
- **kind:** 要使用的排序算法。选项包括 'quicksort'、'mergesort'、'heapsort' 和 'stable'。
- **order:** 在对结构化数组排序时使用,用于定义要比较的字段。
沿轴 0(行)排序
在 NumPy 中沿轴 0 排序是指沿垂直轴排序数组元素,这对应于二维数组中的行。
当您想要根据每一列中的值来排序矩阵的行时,此操作很有用。
在二维数组中:
- 轴 0 对应于行。
- 轴 1 对应于列。
沿轴 0 排序时,NumPy 会按列排序元素。对于每一列,值都按升序排列,行也相应地重新排序。
示例
在下面的示例中,我们使用 sort() 函数对每一列中的元素进行排序:
import numpy as np # Create a 2D array arr = np.array([[3, 6, 4], [5, 1, 2]]) # Sort along axis 0 (rows) sorted_arr_axis0 = np.sort(arr, axis=0) print("Original Array:\n", arr) print("Sorted Along Axis 0:\n", sorted_arr_axis0)
输出将重新排序行,以便每一列中的值按升序排序:
Original Array: [[3 6 4] [5 1 2]] Sorted Along Axis 0: [[3 1 2] [5 6 4]]
沿轴 1(列)排序
在 NumPy 中沿轴 1 排序是指沿水平轴排序数组元素,这对应于二维数组中的列。当您想要根据它们的值来排序每一行中的元素时,此操作很有用。
沿轴 1 排序时,NumPy 会按行排序元素。对于每一行,值都按升序排列,列的顺序也相应地调整。
示例
在这个例子中,我们使用 sort() 函数对每一行中的元素进行排序:
import numpy as np # Create a 2D array arr = np.array([[3, 2, 1], [6, 5, 4]]) # Sort along axis 1 (columns) sorted_arr_axis1 = np.sort(arr, axis=1) print("Original Array:\n", arr) print("Sorted Along Axis 1:\n", sorted_arr_axis1)
输出将重新排序每一行中的列,以便值按升序排序:
Original Array: [[3 2 1] [6 5 4]] Sorted Along Axis 1: [[1 2 3] [4 5 6]]
多维数组排序
NumPy 中的多维数组排序涉及沿一个或多个特定轴组织数组的元素。
在多维数组中沿特定轴排序类似于二维数组中的排序,但扩展到更高维度。以下是沿不同轴排序的工作方式:
- **轴 0(第一维):**沿轴 0 排序会影响行。
- **轴 1(第二维):**沿轴 1 排序会影响列。
- **轴 2(第三维):**在三维数组中,沿轴 2 排序会影响深度切片。
示例
在下面的示例中,我们沿不同的轴对三维数组进行排序:深度(轴 0)、行(轴 1)和列(轴 2)。每次排序操作都会在指定的维度内排列元素,从而产生不同顺序的数组:
import numpy as np # Create a 3D array arr = np.array([[[3, 2, 1], [6, 5, 4]], [[9, 8, 7], [12, 11, 10]]]) # Sort along axis 0 (depth) sorted_arr_axis0 = np.sort(arr, axis=0) # Sort along axis 1 (rows) sorted_arr_axis1 = np.sort(arr, axis=1) # Sort along axis 2 (columns) sorted_arr_axis2 = np.sort(arr, axis=2) print("Original Array:\n", arr) print("Sorted Along Axis 0:\n", sorted_arr_axis0) print("Sorted Along Axis 1:\n", sorted_arr_axis1) print("Sorted Along Axis 2:\n", sorted_arr_axis2)
以下是上述代码的输出:
Original Array: [[[ 3 2 1] [ 6 5 4]] [[ 9 8 7] [12 11 10]]] Sorted Along Axis 0: [[[ 3 2 1] [ 6 5 4]] [[ 9 8 7] [12 11 10]]] Sorted Along Axis 1: [[[ 3 2 1] [ 6 5 4]] [[ 9 8 7] [12 11 10]]] Sorted Along Axis 2: [[[ 1 2 3] [ 4 5 6]] [[ 7 8 9] [10 11 12]]]
使用不同的算法进行排序
NumPy sort() 函数支持几种排序算法,每种算法都有其自身的优势:
- 快速排序 (Quicksort)
- 归并排序 (Mergesort)
- 堆排序 (Heapsort)
- 稳定排序 (Stable Sort)
可以通过在 np.sort() 函数中设置 **kind** 参数来选择这些算法。默认情况下,NumPy 使用“快速排序”算法。
排序算法是按特定顺序排列元素的方法。不同的算法具有不同的性能特征,并且针对各种类型的数据和数组大小进行了优化。
示例
在这个例子中,数组使用“快速排序”和“归并排序”算法沿轴“1”进行排序。不同的排序算法会影响性能和稳定性:
import numpy as np # Create a 2D array arr = np.array([[3, 2, 1], [6, 5, 4]]) # Sort using different algorithms sorted_quicksort = np.sort(arr, axis=1, kind='quicksort') sorted_mergesort = np.sort(arr, axis=1, kind='mergesort') print("Sorted with Quicksort:\n", sorted_quicksort) print("Sorted with Mergesort:\n", sorted_mergesort)
获得的输出如下所示:
Sorted with Quicksort: [[1 2 3] [4 5 6]] Sorted with Mergesort: [[1 2 3] [4 5 6]]