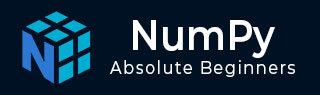
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境配置
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 数组的创建和操作
- NumPy - 数组创建函数
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 数组迭代
- NumPy - 数组重塑
- NumPy - 数组连接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引与切片
- NumPy - 高级索引
- NumPy 数组属性和运算
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组运算
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 复制与视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- NumPy - 加载数组
- NumPy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 填充缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- NumPy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - 索引与切片
ndarray 对象的内容可以通过索引或切片访问和修改,就像 Python 的内置容器对象一样。
如前所述,ndarray 对象中的项目遵循基于零的索引。有三种索引方法可用:字段访问、基本切片和高级索引。
基本切片是 Python 基本切片概念到 n 维的扩展。Python 切片对象是通过向内置的 `slice` 函数提供 `start`、`stop` 和 `step` 参数来构造的。此切片对象传递给数组以提取数组的一部分。
示例 1
import numpy as np a = np.arange(10) s = slice(2,7,2) print a[s]
其输出如下:
[2 4 6]
在上面的示例中,通过 `arange()` 函数准备了一个 `ndarray` 对象。然后定义一个切片对象,其 `start`、`stop` 和 `step` 值分别为 2、7 和 2。当此切片对象传递给 ndarray 时,将切片其从索引 2 开始到 7(不包括 7)的部分,步长为 2。
也可以通过直接在 `ndarray` 对象中使用冒号 `:` 分隔的切片参数 (start:stop:step) 来获得相同的结果。
示例 2
import numpy as np a = np.arange(10) b = a[2:7:2] print b
这里,我们将获得相同的输出:
[2 4 6]
如果只设置一个参数,则将返回对应于该索引的单个项目。如果在其前面插入一个 `:`,则将提取从该索引开始的所有项目。如果使用两个参数(用 `:` 分隔),则将切片两个索引之间的项目(不包括停止索引),默认步长为 1。
示例 3
# slice single item import numpy as np a = np.arange(10) b = a[5] print b
其输出如下:
5
示例 4
# slice items starting from index import numpy as np a = np.arange(10) print a[2:]
现在,输出将是:
[2 3 4 5 6 7 8 9]
示例 5
# slice items between indexes import numpy as np a = np.arange(10) print a[2:5]
这里的输出将是:
[2 3 4]
以上描述也适用于多维 `ndarray`。
示例 6
import numpy as np a = np.array([[1,2,3],[3,4,5],[4,5,6]]) print a # slice items starting from index print 'Now we will slice the array from the index a[1:]' print a[1:]
输出如下:
[[1 2 3] [3 4 5] [4 5 6]] Now we will slice the array from the index a[1:] [[3 4 5] [4 5 6]]
切片还可以包含省略号 (...) 以创建一个与数组维度长度相同的选择元组。如果在行位置使用省略号,它将返回一个包含行中项目的 ndarray。
示例 7
# array to begin with import numpy as np a = np.array([[1,2,3],[3,4,5],[4,5,6]]) print 'Our array is:' print a print '\n' # this returns array of items in the second column print 'The items in the second column are:' print a[...,1] print '\n' # Now we will slice all items from the second row print 'The items in the second row are:' print a[1,...] print '\n' # Now we will slice all items from column 1 onwards print 'The items column 1 onwards are:' print a[...,1:]
此程序的输出如下:
Our array is: [[1 2 3] [3 4 5] [4 5 6]] The items in the second column are: [2 4 5] The items in the second row are: [3 4 5] The items column 1 onwards are: [[2 3] [4 5] [5 6]]
广告