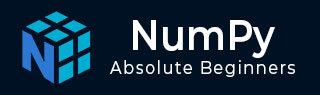
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境配置
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 数组的创建和操作
- NumPy - 数组创建函数
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 数组迭代
- NumPy - 数组重塑
- NumPy - 数组拼接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引与切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组运算
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 拷贝与视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- NumPy - 加载数组
- NumPy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 缺失数据插补
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- NumPy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 平均值
- NumPy - 中位数
- NumPy - 最小值
- NumPy - 最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - 结构化数组
NumPy中的结构化数组
NumPy中的结构化数组是一个数组,其中每个元素都是复合数据类型。这种复合数据类型可以包含多个字段,每个字段都有自己的数据类型,类似于表或记录。
例如,您可以拥有一个数组,其中每个元素都同时包含姓名(作为字符串)和年龄(作为整数)。这有助于您更灵活地处理复杂数据,因为您可以分别访问和操作每个字段。
创建结构化数组
创建结构化数组的第一步是定义数据类型 (dtype),它指定每个元素的结构。**dtype** 定义为元组列表或字典,其中每个元组或字典条目定义一个字段名及其数据类型。
以下是结构化数组中可用的数据类型:
- **'U10':** 长度为 10 的 Unicode 字符串
- **'i4':** 4 字节整数
- **'f8':** 8 字节浮点数
- **'b':** 布尔值
使用元组列表
您可以使用元组列表定义 dtype 并创建结构化数组,其中每个元组表示一个字段。每个元组包含两个元素:第一个元素是字段名,第二个元素是该字段的数据类型。
示例
在下面的示例中,我们使用指定的 dtype 定义了一个包含“name”、“age”和“height”字段的结构化数组。然后我们用相应的数据创建这个数组。
import numpy as np # Define the dtype dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] # Define the data data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] # Create the structured array structured_array = np.array(data, dtype=dtype) print("Structured Array:\n", structured_array)
以下是获得的输出:
Structured Array: [('Alice', 30, 5.6) ('Bob', 25, 5.8) ('Charlie', 35, 5.9)]
使用字典
或者,您可以使用字典定义数据和 dtype,以清晰地指定字段的名称和类型。字典中的每个键代表一个字段名,与每个键关联的值定义该字段的数据类型。
示例
在这个例子中,我们使用字典格式定义结构化数组的 dtype,以指定“name”、“age”和“height”字段。然后我们创建并显示这个结构化数组及其相应的数据,将其组织成支持每个记录中多种数据类型的格式。
import numpy as np # Define the dtype using a dictionary dtype = np.dtype([('name', 'U10'), ('age', 'i4'), ('height', 'f4')]) # Define the data data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] # Create the structured array structured_array = np.array(data, dtype=dtype) print("Structured Array from Dictionary:\n", structured_array)
这将产生以下结果:
Structured Array from Dictionary: [('Alice', 30, 5.6) ('Bob', 25, 5.8) ('Charlie', 35, 5.9)]
访问结构化数组中的字段
您可以使用字段名访问结构化数组中的各个字段。这可以通过使用字段名作为字符串来索引数组来完成。
示例:访问单个字段
在下面的示例中,我们定义了一个包含 'name'、'age' 和 'height' 字段的结构化数组,然后分别访问这些字段。
import numpy as np # Define a dtype and data for a structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] structured_array = np.array(data, dtype=dtype) # Access the 'name' field names = structured_array['name'] print("Names:", names) # Access the 'age' field ages = structured_array['age'] print("Ages:", ages) # Access the 'height' field heights = structured_array['height'] print("Heights:", heights)
以下是上述代码的输出:
Names: ['Alice' 'Bob' 'Charlie'] Ages: [30 25 35] Heights: [5.6 5.8 5.9]
示例:访问行
您可以使用索引访问结构化数组的特定行。这允许您检索完整的记录。在这里,我们检索结构化数组的第一行和第二行。
import numpy as np # Define a dtype and data for a structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] structured_array = np.array(data, dtype=dtype) # Access the first row first_row = structured_array[0] print("First Row:", first_row) # Access the second row second_row = structured_array[1] print("Second Row:", second_row)
以下是上述代码的输出:
First Row: ('Alice', 30, 5.6) Second Row: ('Bob', 25, 5.8)
修改结构化数组的字段
您可以通过索引并将新值赋给它们来修改结构化数组中各个字段的值。
要向结构化数组添加新字段,您可以结合使用 np.concatenate() 函数和创建一个包含附加字段的新 dtype。
NumPy 不支持直接向现有的结构化数组添加字段。
示例:更新字段
在下面的示例中,我们通过直接赋值来更新结构化数组中第一条记录的 'age' 字段。
import numpy as np # Define a dtype and data for a structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] structured_array = np.array(data, dtype=dtype) # Update the 'age' of the first record structured_array[0]['age'] = 31 print("Updated Structured Array:\n", structured_array)
获得的输出如下所示:
Updated Structured Array: [('Alice', 31, 5.6) ('Bob', 25, 5.8) ('Charlie', 35, 5.9)]
示例:添加新字段
在这里,我们通过向其 dtype 添加一个新字段 'weight' 并更新数据以包含此字段来扩展结构化数组。
import numpy as np # Define a dtype and data for the original structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 30, 5.6), ('Bob', 25, 5.8), ('Charlie', 35, 5.9)] structured_array = np.array(data, dtype=dtype) # Define a new dtype with an additional field 'weight' new_dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4'), ('weight', 'f4')] # Define new data including the additional field new_data = [('Alice', 30, 5.6, 55.0), ('Bob', 25, 5.8, 70.0), ('Charlie', 35, 5.9, 80.0)] # Create a new structured array with the additional field new_structured_array = np.array(new_data, dtype=new_dtype) print("New Structured Array with Additional Field:\n", new_structured_array)
执行上述代码后,我们将获得以下输出:
New Structured Array with Additional Field: [('Alice', 30, 5.6, 55.) ('Bob', 25, 5.8, 70.) ('Charlie', 35, 5.9, 80.)]
排序结构化数组
对 NumPy 中的结构化数组进行排序意味着根据一个或多个字段的值来排列数组的元素。
由于结构化数组具有多个字段,因此排序可以基于这些字段中的值。例如,您可以根据年龄或身高对人员数组进行排序。
示例
在下面的示例中,我们通过首先获得将年龄按升序排列的索引来根据 'age' 字段对结构化数组进行排序。然后,我们使用这些索引重新排序整个数组。
import numpy as np # Define a structured array dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 30), ('Bob', 25), ('Charlie', 35)] structured_array = np.array(data, dtype=dtype) # Sort the array by 'age' sorted_indices = np.argsort(structured_array['age']) sorted_array = structured_array[sorted_indices] print("Sorted by Age:\n", sorted_array)
产生的结果如下:
Sorted by Age: [('Bob', 25) ('Alice', 30) ('Charlie', 35)]
过滤结构化数组
过滤结构化数组涉及对一个或多个字段应用条件并检索满足这些条件的元素。
当您想要检索满足特定条件的记录时,这非常有用,例如提取特定字段超过阈值或与特定值匹配的所有条目。
示例
在这个例子中,我们过滤结构化数组,只包含 'age' 字段大于 30 的记录。
import numpy as np # Define a structured array dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 30), ('Bob', 25), ('Charlie', 35)] structured_array = np.array(data, dtype=dtype) # Filter array for ages greater than 30 filtered_array = structured_array[structured_array['age'] > 30] print("Filtered Array (Age > 30):\n", filtered_array)
我们得到如下所示的输出:
Filtered Array (Age > 30):[('Charlie', 35)]
组合结构化数组
组合结构化数组涉及合并或连接具有已定义 dtype 和命名字段的数组。在 NumPy 中,这可以使用 np.concatenate() 函数完成。
示例
在下面的示例中,我们使用 np.concatenate() 函数将两个具有相同 dtype 的结构化数组组合成一个数组。
import numpy as np # Define two structured arrays dtype = [('name', 'U10'), ('age', 'i4')] data1 = [('Alice', 30), ('Bob', 25)] data2 = [('Charlie', 35), ('Dave', 40)] structured_array1 = np.array(data1, dtype=dtype) structured_array2 = np.array(data2, dtype=dtype) # Combine the arrays combined_array = np.concatenate((structured_array1, structured_array2)) print("Combined Structured Array:\n", combined_array)
这将产生一个新的结构化数组,其中包含两个原始数组中的所有记录,如下所示:
Combined Structured Array: [('Alice', 30) ('Bob', 25) ('Charlie', 35) ('Dave', 40)]