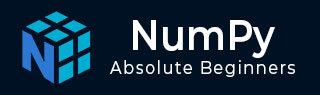
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境配置
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 数组的创建和操作
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 数组迭代
- NumPy - 数组重塑
- NumPy - 数组拼接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引与切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步幅
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 复制与视图
- NumPy - 元素级的数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 缺失数据插补
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级的矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级的矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - Matplotlib
Matplotlib 是 Python 的一个绘图库。它与 NumPy 一起使用,提供了一个有效的开源替代 MatLab 的环境。它也可以与 PyQt 和 wxPython 等图形工具包一起使用。
Matplotlib 模块最初由 John D. Hunter 编写。自 2012 年以来,Michael Droettboom 担任主要开发者。目前,Matplotlib 1.5.1 版本是可用的稳定版本。该软件包以二进制分发版和源代码形式在 www.matplotlib.org 上提供。
通常,通过添加以下语句将软件包导入 Python 脚本:
from matplotlib import pyplot as plt
这里pyplot() 是 matplotlib 库中最重要的函数,用于绘制二维数据。以下脚本绘制方程式 y = 2x + 5
示例
import numpy as np from matplotlib import pyplot as plt x = np.arange(1,11) y = 2 * x + 5 plt.title("Matplotlib demo") plt.xlabel("x axis caption") plt.ylabel("y axis caption") plt.plot(x,y) plt.show()
从np.arange() 函数创建一个 ndarray 对象 x 作为x 轴上的值。y 轴上的对应值存储在另一个ndarray 对象 y 中。这些值使用 matplotlib 包的 pyplot 子模块的plot() 函数绘制。
图形表示由show() 函数显示。
以上代码应产生以下输出:
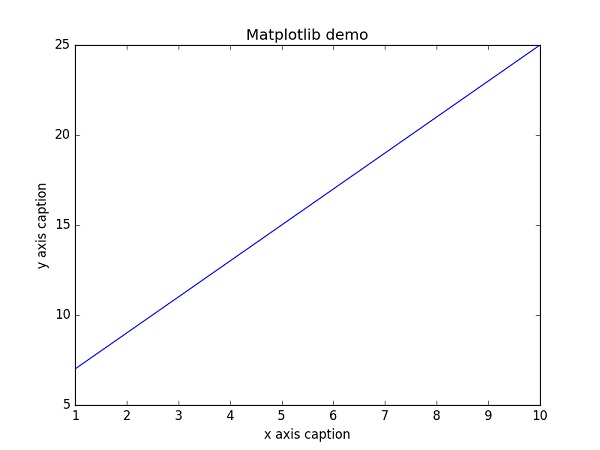
除了线性图,还可以通过向plot() 函数添加格式字符串来离散地显示值。可以使用以下格式字符。
序号 | 字符 & 说明 |
---|---|
1 | '-' 实线样式 |
2 | '--' 虚线样式 |
3 | '-.' 点划线样式 |
4 | ':' 点线样式 |
5 | '.' 点标记 |
6 | ',' 像素标记 |
7 | 'o' 圆圈标记 |
8 | 'v' 下三角标记 |
9 | '^' 上三角标记 |
10 | '<' 左三角标记 |
11 | '>' 右三角标记 |
12 | '1' 下三角标记 |
13 | '2' 上三角标记 |
14 | '3' 左三角标记 |
15 | '4' 右三角标记 |
16 | 's' 正方形标记 |
17 | 'p' 五角星标记 |
18 | '*' 星形标记 |
19 | 'h' 六角形1标记 |
20 | 'H' 六角形2标记 |
21 | '+' 加号标记 |
22 | 'x' X 标记 |
23 | 'D' 菱形标记 |
24 | 'd' 细菱形标记 |
25 | '|' 垂直线标记 |
26 | '_' 水平线标记 |
还定义了以下颜色缩写。
字符 | 颜色 |
---|---|
'b' | 蓝色 |
'g' | 绿色 |
'r' | 红色 |
'c' | 青色 |
'm' | 品红色 |
'y' | 黄色 |
'k' | 黑色 |
'w' | 白色 |
要在上面的示例中显示表示点的圆圈而不是线,请在 plot() 函数中使用“ob”作为格式字符串。
示例
import numpy as np from matplotlib import pyplot as plt x = np.arange(1,11) y = 2 * x + 5 plt.title("Matplotlib demo") plt.xlabel("x axis caption") plt.ylabel("y axis caption") plt.plot(x,y,"ob") plt.show()
以上代码应产生以下输出:
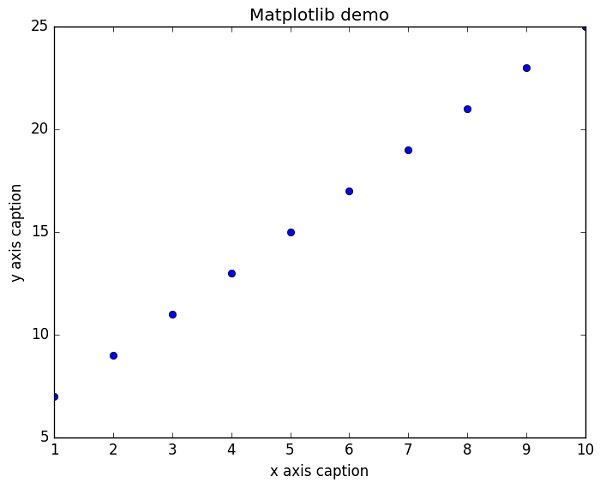
正弦波图
以下脚本使用 matplotlib 绘制正弦波图。
示例
import numpy as np import matplotlib.pyplot as plt # Compute the x and y coordinates for points on a sine curve x = np.arange(0, 3 * np.pi, 0.1) y = np.sin(x) plt.title("sine wave form") # Plot the points using matplotlib plt.plot(x, y) plt.show()
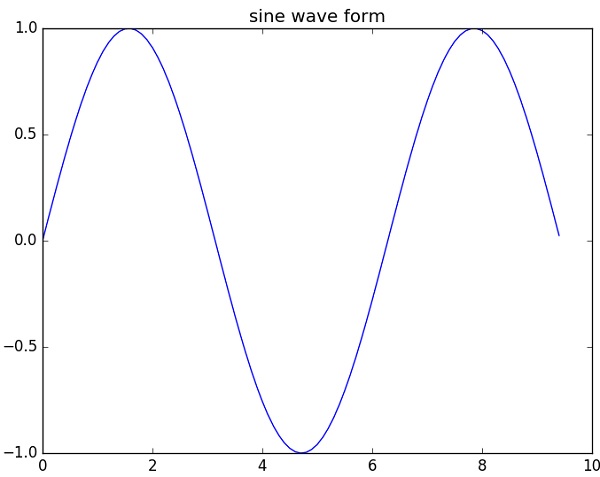
subplot()
subplot() 函数允许您在同一图形中绘制不同的内容。在以下脚本中,绘制了正弦和余弦值。
示例
import numpy as np import matplotlib.pyplot as plt # Compute the x and y coordinates for points on sine and cosine curves x = np.arange(0, 3 * np.pi, 0.1) y_sin = np.sin(x) y_cos = np.cos(x) # Set up a subplot grid that has height 2 and width 1, # and set the first such subplot as active. plt.subplot(2, 1, 1) # Make the first plot plt.plot(x, y_sin) plt.title('Sine') # Set the second subplot as active, and make the second plot. plt.subplot(2, 1, 2) plt.plot(x, y_cos) plt.title('Cosine') # Show the figure. plt.show()
以上代码应产生以下输出:
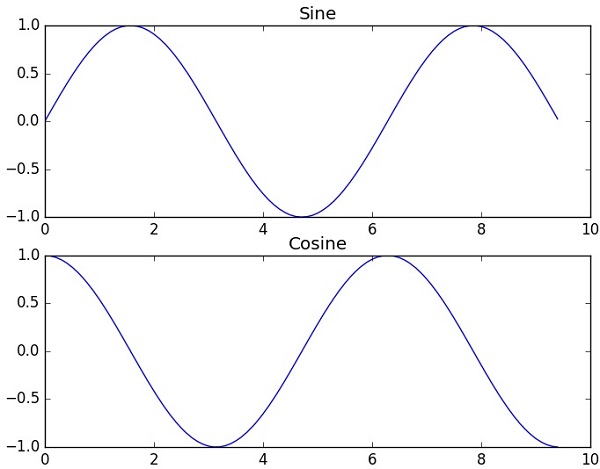
bar()
pyplot 子模块提供bar() 函数来生成条形图。以下示例生成了两组x 和y 数组的条形图。
示例
from matplotlib import pyplot as plt x = [5,8,10] y = [12,16,6] x2 = [6,9,11] y2 = [6,15,7] plt.bar(x, y, align = 'center') plt.bar(x2, y2, color = 'g', align = 'center') plt.title('Bar graph') plt.ylabel('Y axis') plt.xlabel('X axis') plt.show()
此代码应产生以下输出:
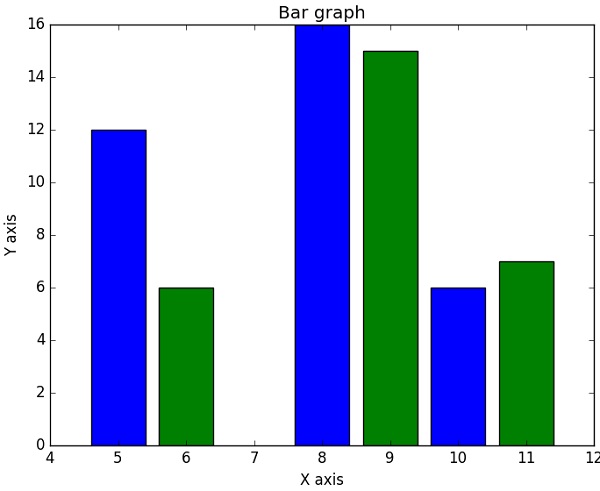