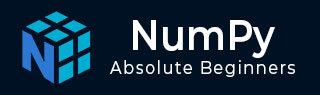
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 遍历数组
- NumPy - 数组重塑
- NumPy - 连接数组
- NumPy - 堆叠数组
- NumPy - 分割数组
- NumPy - 扁平化数组
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组的轴
- NumPy - 字节交换
- NumPy - 复制和视图
- NumPy - 元素级数组比较
- NumPy - 过滤数组
- NumPy - 连接数组
- NumPy - 排序、搜索和计数函数
- NumPy - 搜索数组
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 将值追加到数组
- NumPy - 交换数组的列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 填充缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 平均值
- NumPy - 中位数
- NumPy - 最小值
- NumPy - 最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - 字段访问
NumPy 字段访问
NumPy 中的字段访问是指根据字段名称检索或修改结构化数组中的特定元素。它允许您处理数组中每个记录的各个属性或特性。
NumPy 中的结构化数组使您能够定义包含多个字段的记录数组,每个字段都有自己的数据类型。可以单独访问结构化数组中的字段,从而可以操作数据。
按名称访问单个字段
NumPy 中的结构化数组允许您为每个元素内的不同字段分配名称。此命名约定使您可以轻松地使用这些名称直接访问特定字段。
在 NumPy 中,当使用结构化数组时,访问单个字段允许您与数组中每个元素的特定组件或属性进行交互。这在处理包含多种类型数据的数组时非常重要。
示例
在以下示例中,我们访问结构化数组的“name”字段以提取并检索数组中的所有名称 -
import numpy as np # Define a structured array with fields 'name' and 'age' dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 25), ('Bob', 30)] structured_array = np.array(data, dtype=dtype) # Access the 'name' field names = structured_array['name'] print("Names:", names)
以下是获得的输出 -
Names: ['Alice' 'Bob']
多维数组中的字段访问
要访问多维结构化数组中的特定字段,您可以使用类似于一维和二维数组中使用的索引技术,但应用于多个维度。
多维结构化数组是一个数组,其中每个元素本身都是一个结构化数组,这些元素按多个维度(例如,二维、三维数组)组织。数组中的每个元素都可以有多个字段,类似于一个表,其中每一行都是一个具有多个属性的记录。
示例
在下面的示例中,我们访问三维结构化数组的第一层中的“name”字段 -
import numpy as np # Define a 3D structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [[[('Alice', 25, 5.5), ('Bob', 30, 6.0)], [('Charlie', 35, 5.8), ('David', 40, 6.2)]], [[('Eve', 28, 5.7), ('Frank', 33, 6.1)], [('Grace', 29, 5.6), ('Hank', 32, 6.3)]]] structured_array_3d = np.array(data, dtype=dtype) # Access the 'name' field from the first layer names_layer_0 = structured_array_3d[0]['name'] print("Names in the first layer:\n", names_layer_0)
以下是上述代码的输出 -
Names in the first layer: [['Alice' 'Bob'] ['Charlie' 'David']]
访问特定切片中的字段
访问特定切片中的字段意味着从结构化数组中的特定子集或数据范围检索值。
当您切片结构化数组的单个维度时,您可以访问结果切片中的特定字段。要访问涉及多个维度的切片中的字段,您需要跨维度应用切片,然后从结果子数组中选择字段。
示例:切片一维和访问字段
在以下示例中,我们对结构化数组进行切片以获取行子集,具体为第 1 行和第 2 行。切片后,我们访问并检索此子集中的“name”和“age”字段 -
import numpy as np # Define a structured array with fields 'name' and 'age' dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 25), ('Bob', 30), ('Charlie', 35), ('David', 40)] structured_array = np.array(data, dtype=dtype) # Slice the array to get a subset of rows sliced_array = structured_array[1:3] # Gets rows 1 and 2 # Access the 'name' field from the sliced array names = sliced_array['name'] # Access the 'age' field from the sliced array ages = sliced_array['age'] print("Sliced names:", names) print("Sliced ages:", ages)
获得的输出如下所示 -
Sliced names: ['Bob' 'Charlie'] Sliced ages: [30 35]
示例:切片二维和访问字段
在这里,我们对二维结构化数组进行切片以提取行和列的子集。然后,我们访问并检索此数组切片部分中的“name”和“age”字段 -
import numpy as np # Define a 2D array with structured data dtype = [('name', 'U10'), ('age', 'i4')] data = [[('Alice', 25), ('Bob', 30)], [('Charlie', 35), ('David', 40)]] structured_array = np.array(data, dtype=dtype).view(np.recarray) # Slice the array to get a subset of rows and columns sliced_array = structured_array[0:2, 0:2] # Gets all rows and columns # Access the 'name' field from the sliced array names = sliced_array['name'] # Access the 'age' field from the sliced array ages = sliced_array['age'] print("Sliced names:", names) print("Sliced ages:", ages)
执行上述代码后,我们得到以下输出 -
Sliced names: [['Alice' 'Bob'] ['Charlie' 'David']] Sliced ages: [[25 30] [35 40]]
同时访问多个字段
同时访问多个字段意味着同时从结构化数组中的多个字段检索数据,允许您一起处理字段子集。
要在 NumPy 中同时访问多个字段,您可以使用以下方法 -
- 使用字段名称列表访问:您可以指定字段名称列表以获取仅包含这些字段的结构化数组。
- 使用结构化数组的字段索引:如果您需要按索引访问字段,则可以使用其位置选择它们。
示例
在下面的示例中,我们通过指定字段名称或索引访问结构化数组的不同字段,并打印结果。我们检索特定字段,如“name”和“age”,以及所有字段 -
import numpy as np # Define a structured array with fields 'name', 'age', and 'height' dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 25, 5.5), ('Bob', 30, 6.0), ('Charlie', 35, 5.8)] structured_array = np.array(data, dtype=dtype) # 1. Accessing multiple fields with a list of field names selected_fields = structured_array[['name', 'age']] print("Selected fields (name and age):") print(selected_fields) # 2. Accessing fields by index names = structured_array['name'] ages = structured_array['age'] heights = structured_array['height'] print("\nNames:", names) print("Ages:", ages) print("Heights:", heights) # Accessing all fields simultaneously all_fields = structured_array[['name', 'age', 'height']] print("\nAll fields:") print(all_fields)
产生的结果如下 -
Selected fields (name and age): [('Alice', 25) ('Bob', 30) ('Charlie', 35)] Names: ['Alice' 'Bob' 'Charlie'] Ages: [25 30 35] Heights: [5.5 6. 5.8] All fields: [('Alice', 25, 5.5) ('Bob', 30, 6. ) ('Charlie', 35, 5.8)]
将字段访问与布尔索引结合使用
将字段访问与布尔索引结合使用是指根据应用于数组的条件或过滤器从结构化数组中检索特定字段。
布尔索引允许您选择满足给定条件的数组元素。通过将布尔掩码(一个布尔值数组)应用于结构化数组,您可以根据应用于一个或多个字段的条件过滤数组。
示例
在以下示例中,我们使用布尔掩码根据“age”字段过滤结构化数组。然后,我们选择并打印“age”大于 30 的条目的“name”和“height”字段 -
import numpy as np # Define a structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 25, 5.5), ('Bob', 30, 6.0), ('Charlie', 35, 5.8), ('David', 40, 6.2)] structured_array = np.array(data, dtype=dtype) # Create a boolean mask for filtering based on 'age' mask = structured_array['age'] > 30 # Apply boolean indexing and select 'name' and 'height' fields filtered_fields = structured_array[mask][['name', 'height']] print("Filtered Fields (name and height) where age > 30:\n", filtered_fields)
我们得到如下所示的输出 -
Filtered Fields (name and height) where age > 30:[('Charlie', 5.8) ('David', 6.2)]