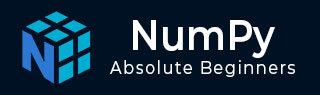
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 遍历数组
- NumPy - 数组重塑
- NumPy - 连接数组
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组扁平化
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组的轴
- NumPy - 字节交换
- NumPy - 复制和视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 连接数组
- NumPy - 排序、搜索和计数函数
- NumPy - 搜索数组
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 将值追加到数组
- NumPy - 交换数组的列
- NumPy - 将轴插入数组
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 估算缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy - 排序、搜索和计数函数
NumPy 提供了各种用于对数组中的元素进行排序、搜索和计数的函数。这些函数对于数据操作和分析非常有用。
NumPy 提供了几种排序算法,每种算法都有其自身的特点。以下是三种常见排序算法的比较:
kind | 速度 | 最坏情况 | 工作空间 | 稳定性 |
---|---|---|---|---|
'quicksort' | 1 | O(n^2) | 0 | 否 |
'mergesort' | 2 | O(n*log(n)) | ~n/2 | 是 |
'heapsort' | 3 | O(n*log(n)) | 0 | 否 |
numpy.sort() 函数
sort() 函数返回输入数组的排序副本。它可以沿任何指定的轴对数组进行排序,并支持不同的排序算法。以下是语法:
numpy.sort(a, axis, kind, order)
其中,
序号 | 参数和描述 |
---|---|
1 | a 要排序的数组 |
2 | axis 要排序的数组的轴。如果为 None,则数组将被展平,并在最后一个轴上排序 |
3 | kind 默认为 quicksort |
4 | order 如果数组包含字段,则要排序的字段的顺序 |
示例
在以下示例中,我们分别使用默认方式和沿特定轴对二维 NumPy 数组进行排序。我们还演示了如何根据特定字段(例如“name”)对结构化数组进行排序:
import numpy as np # Create a 2D array a = np.array([[3, 7], [9, 1]]) print("Our array is:",a) # Default sort print("Applying sort() function:",np.sort(a)) # Sort along axis 0 print("Sort along axis 0:",np.sort(a, axis=0)) # Order parameter in sort function dt = np.dtype([('name', 'S10'), ('age', int)]) a = np.array([("raju", 21), ("anil", 25), ("ravi", 17), ("amar", 27)], dtype=dt) print("Our array is:",a) print("Order by name:",np.sort(a, order='name'))
它将产生以下输出:
Our array is: [[3 7] [9 1]] Applying sort() function: [[3 7] [1 9]] Sort along axis 0: [[3 1] [9 7]] Our array is: [('raju', 21) ('anil', 25) ('ravi', 17) ('amar', 27)] Order by name: [('amar', 27) ('anil', 25) ('raju', 21) ('ravi', 17)]
numpy.argsort() 函数
numpy.argsort() 函数对输入数组沿给定轴并使用指定的排序类型进行间接排序,并返回数据索引的数组。此索引数组用于构造排序数组。
示例
在此示例中,我们使用 argsort() 函数检索索引,即排序元素在原始数组中的位置。使用这些索引,您可以重建排序数组:
import numpy as np # Create an array x = np.array([3, 1, 2]) print("Our array is:",x) # Get indices that would sort the array y = np.argsort(x) print("Applying argsort() to x:",y) # Reconstruct the sorted array using the indices print("Reconstruct original array in sorted order:",x[y]) # Reconstruct the original array using a loop print("Reconstruct the original array using loop:") for i in y: print(x[i], end=' ')
它将产生以下输出:
Our array is: [3 1 2] Applying argsort() to x: [1 2 0] Reconstruct original array in sorted order: [1 2 3] Reconstruct the original array using loop: 1 2 3
numpy.lexsort() 函数
NumPy lexort() 函数使用一系列键执行间接排序。键可以看作电子表格中的列。该函数返回一个索引数组,可以使用它来获取排序数据。请注意,最后一个键恰好是排序的主键。
示例
在此示例中,我们使用 np.lexsort() 函数根据多个键对数据集进行排序,其中最后一个键“nm”是主要排序标准。然后使用排序索引通过组合名称和相应的字段来显示排序数据:
import numpy as np # Define keys nm = ('raju', 'anil', 'ravi', 'amar') dv = ('f.y.', 's.y.', 's.y.', 'f.y.') # Get indices for sorted order ind = np.lexsort((dv, nm)) print("Applying lexsort() function:",ind) # Use indices to get sorted data print("Use this index to get sorted data:",[nm[i] + ", " + dv[i] for i in ind])
它将产生以下输出:
Applying lexsort() function: [3 1 0 2] Use this index to get sorted data: ['amar, f.y.', 'anil, s.y.', 'raju, f.y.', 'ravi, s.y.']
NumPy 提供了用于查找最大值、最小值和非零元素以及满足条件的元素的索引的函数。
numpy.argmax() 和 numpy.argmin() 函数
NumPy argmax() 和 argmin() 函数分别返回沿给定轴的最大和最小元素的索引。
示例
在此示例中,我们使用 np.argmax() 和 np.argmin() 函数查找二维数组中最大值和最小值的索引,包括展平数组和沿特定轴的情况:
import numpy as np # Create a 2D array a = np.array([[30, 40, 70], [80, 20, 10], [50, 90, 60]]) print("Our array is:",a) # Apply argmax() function print("Applying argmax() function:",np.argmax(a)) # Index of maximum number in flattened array print("Index of maximum number in flattened array:",a.flatten()) # Array containing indices of maximum along axis 0 print("Array containing indices of maximum along axis 0:") maxindex = np.argmax(a, axis=0) print(maxindex) # Array containing indices of maximum along axis 1 print("Array containing indices of maximum along axis 1:") maxindex = np.argmax(a, axis=1) print(maxindex) # Apply argmin() function print("Applying argmin() function:") minindex = np.argmin(a) print(minindex) # Flattened array print("Flattened array:",a.flatten()[minindex]) # Flattened array along axis 0 print("Flattened array along axis 0:") minindex = np.argmin(a, axis=0) print(minindex) # Flattened array along axis 1 print("Flattened array along axis 1:") minindex = np.argmin(a, axis=1) print(minindex)
输出包括这些极值的索引,演示了如何在数组中访问和解释这些位置:
Our array is: [[30 40 70] [80 20 10] [50 90 60]] Applying argmax() function: 7 Index of maximum number in flattened array [30 40 70 80 20 10 50 90 60] Array containing indices of maximum along axis 0: [1 2 0] Array containing indices of maximum along axis 1: [2 0 1] Applying argmin() function: 5 Flattened array: 10 Flattened array along axis 0: [0 1 1] Flattened array along axis 1: [0 2 0]
numpy.nonzero() 函数
numpy.nonzero() 函数返回输入数组中非零元素的索引。
示例
在下面的示例中,我们使用 nonzero() 函数检索数组“a”中非零元素的索引:
import numpy as np a = np.array([[30,40,0],[0,20,10],[50,0,60]]) print ('Our array is:',a) print ('Applying nonzero() function:',np.nonzero (a))
它将产生以下输出:
Our array is: [[30 40 0] [ 0 20 10] [50 0 60]] Applying nonzero() function: (array([0, 0, 1, 1, 2, 2]), array([0, 1, 1, 2, 0, 2]))
numpy.where() 函数
where() 函数返回输入数组中满足给定条件的元素的索引,如下面的示例所示:
import numpy as np x = np.arange(9.).reshape(3, 3) print ('Our array is:',x) print ('Indices of elements > 3') y = np.where(x > 3) print (y) print ('Use these indices to get elements satisfying the condition',x[y])
它将产生以下输出:
Our array is: [[ 0. 1. 2.] [ 3. 4. 5.] [ 6. 7. 8.]] Indices of elements > 3 (array([1, 1, 2, 2, 2]), array([1, 2, 0, 1, 2])) Use these indices to get elements satisfying the condition [ 4. 5. 6. 7. 8.]
numpy.extract() 函数
extract() 函数返回满足任何条件的元素,如下面的示例所示:
import numpy as np x = np.arange(9.).reshape(3, 3) print ('Our array is:',x) # define a condition condition = np.mod(x,2) == 0 print ('Element-wise value of condition',condition) print ('Extract elements using condition',np.extract(condition, x))
它将产生以下输出:
Our array is: [[ 0. 1. 2.] [ 3. 4. 5.] [ 6. 7. 8.]] Element-wise value of condition [[ True False True] [False True False] [ True False True]] Extract elements using condition [ 0. 2. 4. 6. 8.]