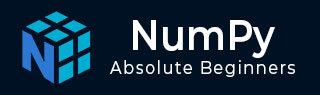
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 迭代数组
- NumPy - 数组重塑
- NumPy - 数组连接
- NumPy - 数组堆叠
- NumPy - 数组分割
- NumPy - 数组展平
- NumPy - 数组转置
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和运算
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组运算
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 复制和视图
- NumPy - 元素级数组比较
- NumPy - 数组过滤
- NumPy - 数组连接
- NumPy - 排序、搜索和计数函数
- NumPy - 数组搜索
- NumPy - 数组并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- NumPy - 加载数组
- NumPy - 保存数组
- NumPy - 向数组追加值
- NumPy - 交换数组列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 估算缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- NumPy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 平均值
- NumPy - 中位数
- NumPy - 最小值
- NumPy - 最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy 左移() 函数
NumPy 的left_shift()函数用于对数组元素执行按位左移运算。
当给出两个数组或一个数组和一个标量时,它会将第一个数组中每个元素的位向左移动第二个数组或标量中指定的位数。结果相当于将数组元素乘以 2**shift。
- 此函数通过允许对不同形状的数组进行运算来支持广播。它在低级数据操作(如二进制编码、图像处理和需要精确位控制的操作)中很有用。
- 在按位运算的上下文中,负移位值通常与右移运算一起使用,其中位向右移动。
- 在左移运算中,负值没有有意义的解释,行为可能不一致或未定义。
语法
以下是 NumPy left_shift() 函数的语法:
numpy.left_shift(x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature])
参数
以下是 NumPy left_shift() 函数的参数:
- x1(array_like): 对其执行左移运算的输入数组。
- x2(array_like 或 int): 将 x1 的每个元素左移的位数。它可以是单个整数或与 x1 形状相同的数组。
- out(可选): 这是存储结果的可选输出数组。
- where(可选): 用于确定应执行移位运算的位置的条件。在此条件为 True 的情况下计算结果。
- **kwargs: 参数 casting、order、dtype、subok 属于附加关键字参数。
返回值
该函数返回一个数组,其中每个元素都是左移原始数组对应位的位的结果。
示例 1
以下是 NumPy left_shift() 函数的基本示例,其中数组的单个整数被左移:
import numpy as np # Shift the bits of the integer 5 to the left by 1 position result = np.left_shift(5, 1) print(result)
以下是 left_shift() 函数的输出:
10
示例 2
我们可以通过将每个元素的位向左移动指定的位数来对数组的所有元素执行统一的标量移位。在此示例中,我们将数组左移 3 位:
import numpy as np # Define an array of integers array = np.array([5, 10, 15, 20]) # Define the scalar shift value shift = 3 # Perform the left shift operation result = np.left_shift(array, shift) print("Original array:", array) print("Shifted array:", result)
以下是上述示例的输出:
Original array: [ 5 10 15 20] Shifted array: [ 40 80 120 160]
示例 3
以下示例对数字 10 执行左移两位,并显示结果,包括原始值和移位值的二进制表示:
import numpy as np print('Left shift of 10 by two positions:') print(np.left_shift(10, 2)) print('Binary representation of 10:') print(np.binary_repr(10, width=8)) print('Binary representation of 40:') print(np.binary_repr(40, width=8))
以下是上述示例的输出:
Left shift of 10 by two positions: 40 Binary representation of 10: 00001010 Binary representation of 40: 00101000
示例 2
在此示例中,left_shift() 不会以标准方式处理负移位,并且左移的结果可能与预期不符。相反,结果通常没有意义,并且可能默认为与在此示例中看到的值相同的值:
import numpy as np # Define an array of integers array = np.array([16, 32, 64, 128]) # Define the array of shift values, including negative values shifts = np.array([-1, -2, -3, -4]) # Perform the left shift operation result = np.left_shift(array, shifts) print('Original array:', array) print('Shift values:', shifts) print('Shifted array:', result)
以下是上述示例的输出:
Original array: [ 16 32 64 128] Shift values: [-1 -2 -3 -4] Shifted array: [0 0 0 0]
numpy_binary_operators.htm
广告