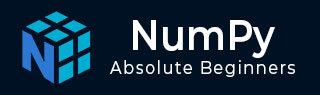
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 遍历数组
- NumPy - 数组重塑
- NumPy - 连接数组
- NumPy - 堆叠数组
- NumPy - 分割数组
- NumPy - 扁平化数组
- NumPy - 转置数组
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 副本和视图
- NumPy - 元素级数组比较
- NumPy - 过滤数组
- NumPy - 连接数组
- NumPy - 排序、搜索和计数函数
- NumPy - 搜索数组
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O 操作
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 将值追加到数组
- NumPy - 交换数组的列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 填充缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 平均值
- NumPy - 中位数
- NumPy - 最小值
- NumPy - 最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy resize() 函数
Numpy 的resize()函数返回一个具有指定形状的新数组,并调整输入数组的大小。reshape()函数要求元素总数保持不变,而resize()可以通过截断或填充数组来更改元素总数。
如果新形状大于原始形状,则数组将用原始数据的重复副本填充。如果新形状较小,则数组将被截断。
此函数以输入数组和新形状作为参数,并可以选择一个refcheck参数来控制是否检查对原始数组的引用。
语法
以下是 Numpy resize() 函数的语法:
numpy.resize(arr, shape)
参数
以下是 Numpy resize() 函数的参数:
- arr: 要调整大小的输入数组。
- shape: 结果数组的新形状。
- shape: 结果数组的新形状。
示例 1
以下是 Numpy resize() 函数的示例,它展示了如何重塑一个二维数组,通过截断或重复元素以适应新的指定维度:
import numpy as np # Create a 2D array a = np.array([[1, 2, 3], [4, 5, 6]]) print('First array:') print(a) print('\n') print('The shape of the first array:') print(a.shape) print('\n') # Resize the array to shape (3, 2) b = np.resize(a, (3, 2)) print('Second array:') print(b) print('\n') print('The shape of the second array:') print(b.shape) print('\n') # Resize the array to shape (3, 3) # Note: This will repeat elements of 'a' to fill the new shape print('Resize the second array:') b = np.resize(a, (3, 3)) print(b)
以上程序将产生以下输出:
First array: [[1 2 3] [4 5 6]] The shape of first array: (2, 3) Second array: [[1 2] [3 4] [5 6]] The shape of second array: (3, 2) Resize the second array: [[1 2 3] [4 5 6] [1 2 3]]
示例 2
以下是另一个将大小为 4x3 的给定数组调整为 6x2 和 3x4 的示例:
import numpy as np # Create an initial 4x3 array array = np.array([[1, 2, 3], [4, 5, 6],[7,8,9],[10,11,12]]) print("Original array:") print(array) print("\n") # Resize the array to shape (6, 2) resized_array = np.resize(array, (6, 2)) print("Resized array to shape (6, 2):") print(resized_array) print("\n") # Resize the array to shape (3, 4) resized_array_larger = np.resize(array, (3, 4)) print("Resized array to shape (3, 4) with repeated elements:") print(resized_array_larger)
以上程序将产生以下输出:
Original array: [[ 1 2 3] [ 4 5 6] [ 7 8 9] [10 11 12]] Resized array to shape (6, 2): [[ 1 2] [ 3 4] [ 5 6] [ 7 8] [ 9 10] [11 12]] Resized array to shape (3, 4) with repeated elements: [[ 1 2 3 4] [ 5 6 7 8] [ 9 10 11 12]]
numpy_array_manipulation.htm
广告