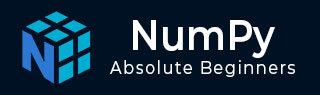
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 迭代数组
- NumPy - 数组重塑
- NumPy - 连接数组
- NumPy - 堆叠数组
- NumPy - 分割数组
- NumPy - 展平数组
- NumPy - 转置数组
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组轴
- NumPy - 字节交换
- NumPy - 复制和视图
- NumPy - 元素级数组比较
- NumPy - 过滤数组
- NumPy - 连接数组
- NumPy - 排序、搜索和计数函数
- NumPy - 搜索数组
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O
- NumPy 排序和高级操作
- NumPy - 数组排序
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- Numpy - 加载数组
- Numpy - 保存数组
- NumPy - 将值追加到数组
- NumPy - 交换数组的列
- NumPy - 将轴插入数组
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 估算缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行矢量化
- NumPy - 数组的内存布局
- Numpy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy 右移() 函数
NumPy 的right_shift()函数用于对数组的元素执行按位右移运算。此函数将输入数组中每个元素的位向右移动指定数量的位置。每当一位移动时,在左侧插入一个零。
此函数可以接受数组或标量作为输入,以及要移动的位置数。结果是一个与输入形状相同的数组,其中包含移位后的值。它通常用于涉及按位操作的任务,例如二进制算术或数字信号处理。
语法
以下是 Numpy right_shift() 函数的语法:
numpy.right_shift(x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True, [,signature])
参数
Numpy right_shift() 函数接受以下参数:
- x1: 应用右移运算的输入值。
- x2: 要应用的右移位置数。
- out(ndarray, None 或 ndarray 和 None 的元组,可选):存储结果的位置。
- where(array_like,可选):这是必须应用右移运算的条件。
- **kwargs: 参数(如 casting、order、dtype、subok)是其他关键字参数,可根据需要使用。
返回值
此函数返回一个数组,其中每个元素都是将输入值中相应元素的位向右移动 shift 指定的位置数的结果。
示例 1
以下是 Numpy right_shift() 函数的基本示例,其中将整数的位向右移动指定数量的位置,有效地将数字除以 2 的幂:
import numpy as np # Define an integer number = 16 # Binary: 00010000 # Define the number of positions to shift shift_positions = 2 # Perform the right shift operation result = np.right_shift(number, shift_positions) print('Original number:', number) print('Binary representation:', np.binary_repr(number, width=8)) print(f'Right shift by {shift_positions} positions results in:', result) print('Binary representation after shift:', np.binary_repr(result, width=8))
以下是应用于整数的right_shift() 函数的输出:
Original number: 16 Binary representation: 00010000 Right shift by 2 positions results in: 4 Binary representation after shift: 00000100
示例 2
在处理按位移位运算时,通常不支持负移位值。尝试使用负移位值通常会导致错误或未定义的行为,具体取决于实现和特定的操作。以下是它的示例:
import numpy as np # Define an array of integers array = np.array([16, 32, 64, 128]) # Define a negative number of positions to shift negative_shift_positions = -2 try: # Attempt to perform the right shift operation with a negative shift value result = np.right_shift(array, negative_shift_positions) print('Result with negative shift value:', result) except ValueError as e: print('Error:', e)
以下是负右移的输出:
Result with negative shift value: [0 0 0 0]
示例 3
这是使用right_shift() 函数执行位右移运算的另一个示例:
import numpy as np # Create a One-Dimensional array arr = np.array([56, 87, 23, 92, 81, 98, 45, 98]) # Displaying our array print("Array:", arr) # Get the datatype print("Array datatype:", arr.dtype) # Get the dimensions of the Array print("Array Dimensions:", arr.ndim) # Get the shape of the Array print("Our Array Shape:", arr.shape) # Get the number of elements of the Array print("Elements in the Array:", arr.size) # The count of right shift valRight = 3 # To shift the bits of an integer to the right, use the numpy.right_shift() method in Python Numpy result = np.right_shift(arr, valRight) # Display the result of the right shift operation print("Result (right shift):", result)
以下是右移的输出:
Array: [56 87 23 92 81 98 45 98] Array datatype: int64 Array Dimensions: 1 Our Array Shape: (8,) Elements in the Array: 8 Result (right shift): [ 7 10 2 11 10 12 5 12]
numpy_binary_operators.htm
广告