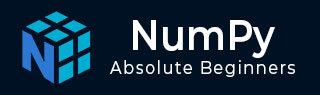
- NumPy 教程
- NumPy - 首页
- NumPy - 简介
- NumPy - 环境
- NumPy 数组
- NumPy - Ndarray 对象
- NumPy - 数据类型
- NumPy 创建和操作数组
- NumPy - 数组创建例程
- NumPy - 数组操作
- NumPy - 从现有数据创建数组
- NumPy - 从数值范围创建数组
- NumPy - 遍历数组
- NumPy - 重塑数组
- NumPy - 连接数组
- NumPy - 堆叠数组
- NumPy - 分割数组
- NumPy - 展平数组
- NumPy - 转置数组
- NumPy 索引和切片
- NumPy - 索引和切片
- NumPy - 高级索引
- NumPy 数组属性和操作
- NumPy - 数组属性
- NumPy - 数组形状
- NumPy - 数组大小
- NumPy - 数组步长
- NumPy - 数组元素大小
- NumPy - 广播
- NumPy - 算术运算
- NumPy - 数组加法
- NumPy - 数组减法
- NumPy - 数组乘法
- NumPy - 数组除法
- NumPy 高级数组操作
- NumPy - 交换数组的轴
- NumPy - 字节交换
- NumPy - 复制和视图
- NumPy - 元素级数组比较
- NumPy - 过滤数组
- NumPy - 连接数组
- NumPy - 排序、搜索和计数函数
- NumPy - 搜索数组
- NumPy - 数组的并集
- NumPy - 查找唯一行
- NumPy - 创建日期时间数组
- NumPy - 二元运算符
- NumPy - 字符串函数
- NumPy - 数学函数
- NumPy - 统计函数
- NumPy - 矩阵库
- NumPy - 线性代数
- NumPy - Matplotlib
- NumPy - 使用 Matplotlib 绘制直方图
- NumPy - NumPy 的 I/O
- NumPy 排序和高级操作
- NumPy - 排序数组
- NumPy - 沿轴排序
- NumPy - 使用花式索引排序
- NumPy - 结构化数组
- NumPy - 创建结构化数组
- NumPy - 操作结构化数组
- NumPy - 字段访问
- NumPy - 记录数组
- NumPy - 加载数组
- NumPy - 保存数组
- NumPy - 将值追加到数组
- NumPy - 交换数组的列
- NumPy - 向数组插入轴
- NumPy 处理缺失数据
- NumPy - 处理缺失数据
- NumPy - 识别缺失值
- NumPy - 删除缺失数据
- NumPy - 填充缺失数据
- NumPy 性能优化
- NumPy - 使用数组进行性能优化
- NumPy - 使用数组进行向量化
- NumPy - 数组的内存布局
- NumPy 线性代数
- NumPy - 线性代数
- NumPy - 矩阵库
- NumPy - 矩阵加法
- NumPy - 矩阵减法
- NumPy - 矩阵乘法
- NumPy - 元素级矩阵运算
- NumPy - 点积
- NumPy - 矩阵求逆
- NumPy - 行列式计算
- NumPy - 特征值
- NumPy - 特征向量
- NumPy - 奇异值分解
- NumPy - 求解线性方程组
- NumPy - 矩阵范数
- NumPy 元素级矩阵运算
- NumPy - 求和
- NumPy - 求平均值
- NumPy - 求中位数
- NumPy - 求最小值
- NumPy - 求最大值
- NumPy 集合运算
- NumPy - 唯一元素
- NumPy - 交集
- NumPy - 并集
- NumPy - 差集
- NumPy 有用资源
- NumPy 编译器
- NumPy - 快速指南
- NumPy - 有用资源
- NumPy - 讨论
NumPy unique() 函数
NumPy 的unique()函数用于返回数组中排序后的唯一元素。它还可以选择性地返回输入数组中给出唯一值的索引以及每个唯一值的计数。
此函数可用于从数组中删除重复项并了解元素的频率。
语法
以下是 NumPy unique()函数的语法:
numpy.unique(arr, return_index, return_inverse, return_counts)
参数
以下是 NumPy unique()函数的参数:
- arr: 输入数组。如果不是一维数组,将被展平。
- return_index: 如果为 True,则返回输入数组中元素的索引。
- return_inverse: 如果为 True,则返回唯一数组的索引,可用于重建输入数组。
- return_counts: 如果为 True,则返回唯一数组中元素在原始数组中出现的次数。
示例 1
以下是 NumPy unique()函数的示例,其中创建了一个包含给定输入数组唯一值的数组:
import numpy as np # Create a 1D array a = np.array([5, 2, 6, 2, 7, 5, 6, 8, 2, 9]) print('First array:') print(a) print('\n') # Get unique values in the array print('Unique values of first array:') u = np.unique(a) print(u) print('\n')
输出
First array: [5 2 6 2 7 5 6 8 2 9] Unique values of first array: [2 5 6 7 8 9]
示例 2
在本示例中,我们使用 unique() 函数获取唯一值及其索引:
import numpy as np # Create a 1D array a = np.array([5, 2, 6, 2, 1, 7, 5, 6, 8, 2, 9]) print('First array:') print(a) print('\n') # Get unique values and their indices in the original array print('Unique array and Indices array:') u, indices = np.unique(a, return_index=True) print(indices) print('\n') print('We can see each number corresponds to index in original array:') print(a) print('\n')
输出
First array: [5 2 6 2 1 7 5 6 8 2 9] Unique array and Indices array: [ 4 1 0 2 5 8 10] We can see each number corresponds to index in original array: [5 2 6 2 1 7 5 6 8 2 9]
示例 3
以下是使用唯一元素及其索引重建原始数组的示例:
import numpy as np # Create a 1D array a = np.array([5, 2, 6, 2, 7, 5, 6, 8, 2, 9]) print('First array:') print(a) print('\n') # Get unique values and indices to reconstruct the original array print('Indices of unique array:') u, indices = np.unique(a, return_inverse=True) print(u) print('\n') print('Indices are:') print(indices) print('\n') print('Reconstruct the original array using indices:') print(u[indices]) print('\n') # Get counts of unique values print('Return the count of repetitions of unique elements:') u, indices = np.unique(a, return_counts=True) print(u) print(indices)
输出
First array: [5 2 6 2 7 5 6 8 2 9] Indices of unique array: [2 5 6 7 8 9] Indices are: [1 0 2 0 3 1 2 4 0 5] Reconstruct the original array using indices: [5 2 6 2 7 5 6 8 2 9] Return the count of repetitions of unique elements: [2 5 6 7 8 9] [3 2 2 1 1 1]
numpy_array_manipulation.htm
广告